How to Handle Text Overflow in Flutter
Whenever we use dynamic text content the chances are high that the content overflows the predefined size of the Text. In this Flutter tutorial, let’s learn how to handle Text overflow with multiple options.
We use maxLines property of text to limit the number of lines and the overflow property decides how to truncate the extra text. See the following code snippet.
Text(
'This is Flutter Text Overflow tutorial and it will truncated!',
maxLines: 1,
textAlign: TextAlign.center,
overflow: TextOverflow.ellipsis,
style: TextStyle(fontSize: 18),
)
The TextOverflow.ellipsis makes the text ends with three dots.
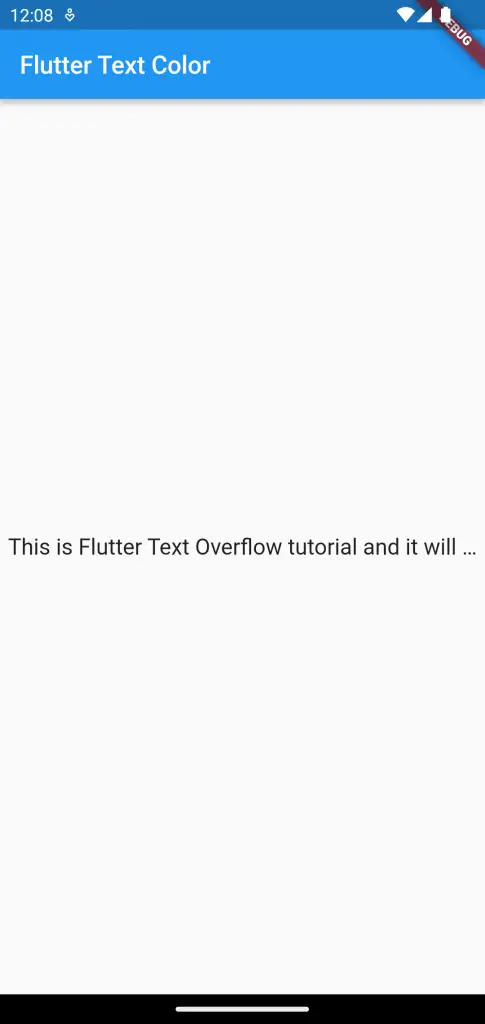
You can also give a fade effect to the Text widget to indicate the text is overflowing.
Text(
'This is Flutter Text Overflow tutorial and it will truncated!',
maxLines: 1,
textAlign: TextAlign.center,
overflow: TextOverflow.fade,
style: TextStyle(fontSize: 18, color: Colors.red),
)
You will get the following output.
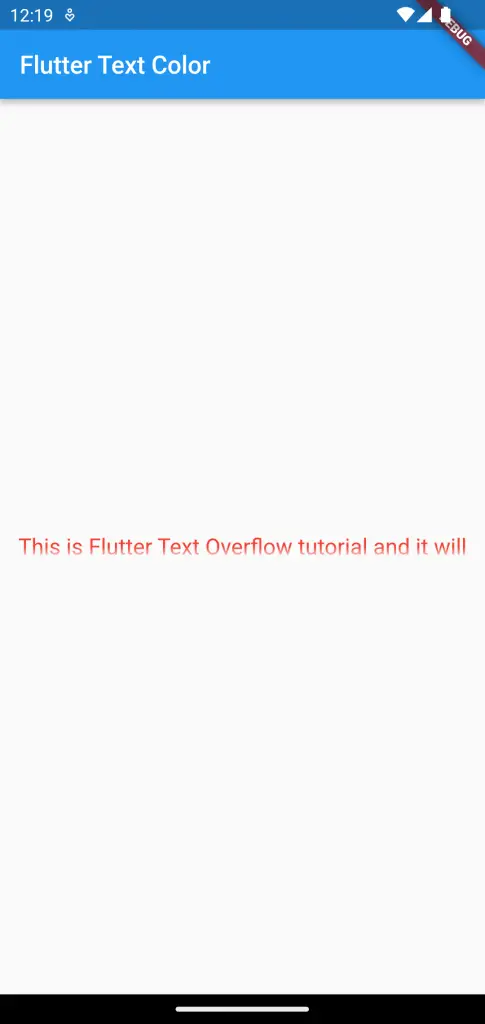
The complete code is given below.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Text Color'),
),
body: const Center(
child: Text(
'This is Flutter Text Overflow tutorial and it will truncated!',
maxLines: 1,
textAlign: TextAlign.center,
overflow: TextOverflow.fade,
style: TextStyle(fontSize: 18, color: Colors.red),
)),
);
}
}
I hope this Flutter text overflow tutorial is helpful for you.