How to Disable Text Wrap in Flutter
By default, text wrapping is enabled in the Flutter Text widget. Thus, the text content breaks at soft line breaks. Let’s check how to disable this Text Wrap feature in Flutter.
The Text widget has a property named softWrap that controls soft wrapping. Let’s check how the Text acts when the softWrap is enabled.
Text(
'This is Flutter Text Wrap tutorial and how to disable it!',
textAlign: TextAlign.center,
style: TextStyle(fontSize: 18, color: Colors.red),
)
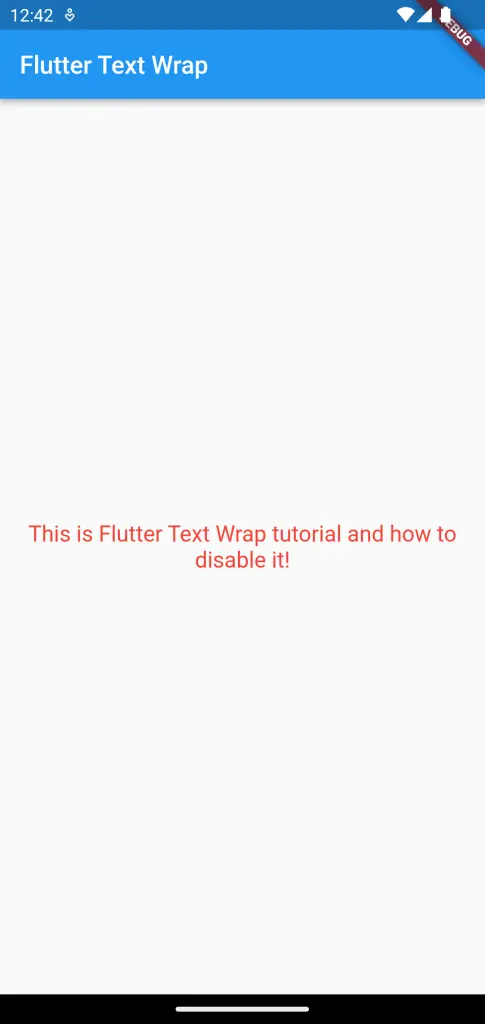
As you see, the soft wrap is enabled by default, and the line breaks for the exceeded text. Now, let’s set softWrap as false.
Text(
'This is Flutter Text Wrap tutorial and how to disable it!',
textAlign: TextAlign.center,
style: TextStyle(fontSize: 18, color: Colors.red),
)
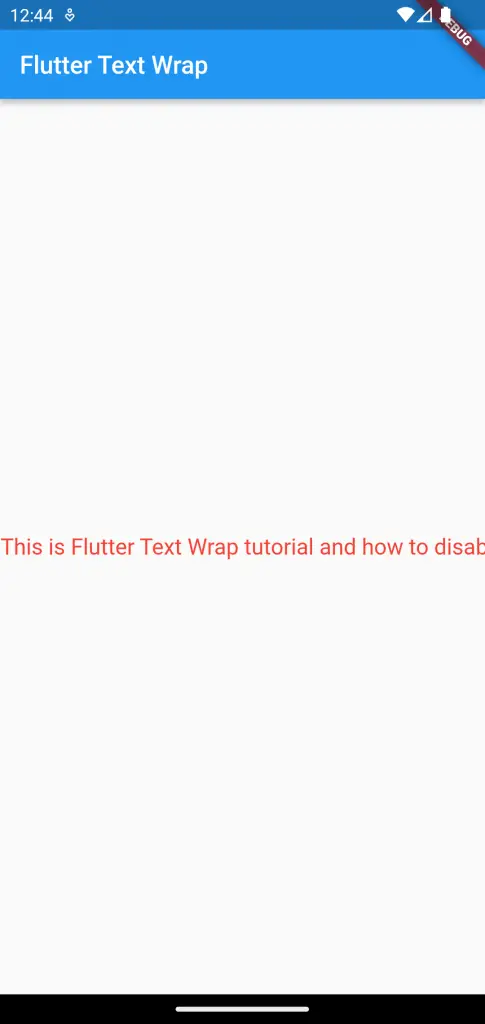
Now you can see the text wrap is disabled. If you want to handle text overflow then please read this Flutter tutorial.
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Text Wrap'),
),
body: const Center(
child: Text(
'This is Flutter Text Wrap tutorial and how to disable it!',
textAlign: TextAlign.center,
softWrap: false,
style: TextStyle(fontSize: 18, color: Colors.red),
)),
);
}
}
That’s how you disable soft text wrap in Flutter.