How to Set DataTable Column Width in Flutter
DataTables play a crucial role in displaying and organizing large amounts of data in a clean, user-friendly manner. However, without proper column width management, it can be difficult for users to effectively read and interpret the data.
In this blog post, let’s learn how to control the width of columns in DataTable.
There’s only one property that directly has control over the width of the columns. That is columnSpacing.
The columnSpacing property determines the horizontal margin between the contents of each column. By default, its value is 56. You can change that value to adjust the width.
For example, the following is a DataTable with default column spacing.
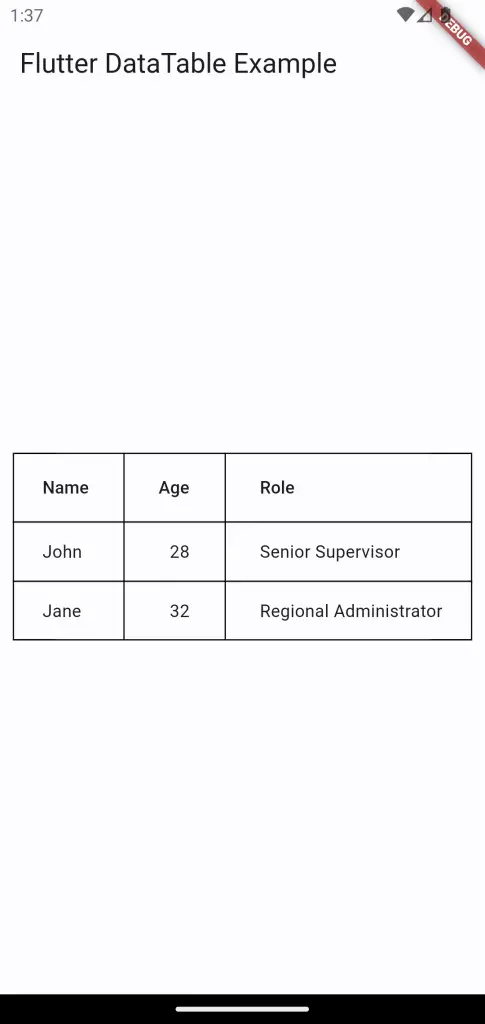
In the following code, I changed the default value of the columnSpacing to 10.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
List<dynamic> data = [
{"Name": "John", "Age": 28, "Role": "Senior Supervisor"},
{"Name": "Jane", "Age": 32, "Role": "Regional Administrator"}
];
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
useMaterial3: true,
colorSchemeSeed: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter DataTable Example'),
),
body: Center(
child: DataTable(
border: TableBorder.all(width: 1),
columnSpacing: 10,
columns: const <DataColumn>[
DataColumn(
label: Text('Name'),
),
DataColumn(
label: Text('Age'),
numeric: true,
),
DataColumn(
label: Text('Role'),
),
],
rows: data
.map(
(item) => DataRow(cells: [
DataCell(Text(item['Name'])),
DataCell(Text(item['Age'].toString())),
DataCell(Text(item['Role'])),
]),
)
.toList(),
),
),
);
}
}
Then you will get the following output with reduced column width.
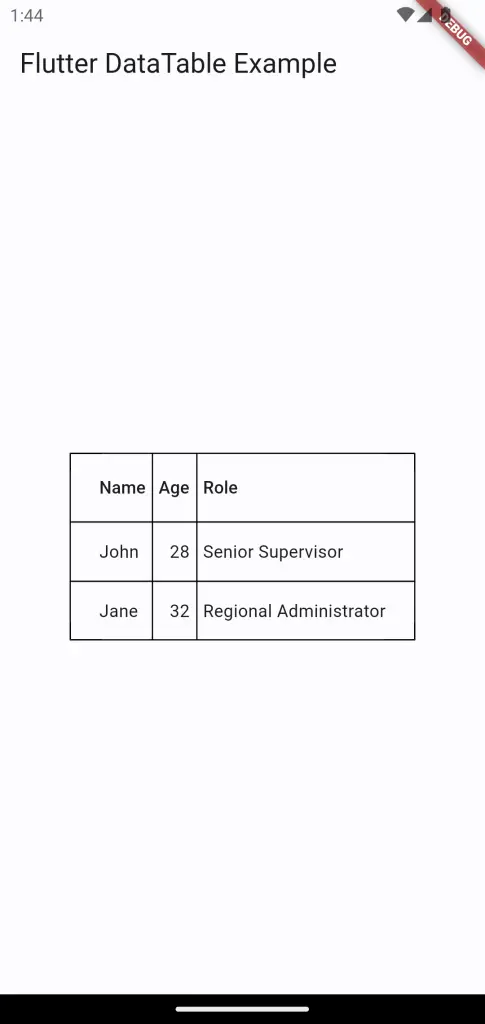
Thus you can adjust the column width using the columnSpacing property.
If you want to wrap the row content then you can use SizedBox to fix the width of the row. This will gradually result in the reduced column width.
In the above example, the Role column size can be reduced by modifying the DataCell. See the following code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
List<dynamic> data = [
{"Name": "John", "Age": 28, "Role": "Senior Supervisor"},
{"Name": "Jane", "Age": 32, "Role": "Regional Administrator"}
];
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
useMaterial3: true,
colorSchemeSeed: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter DataTable Example'),
),
body: Center(
child: DataTable(
border: TableBorder.all(width: 1),
columnSpacing: 10,
columns: const <DataColumn>[
DataColumn(
label: Text('Name'),
),
DataColumn(
label: Text('Age'),
numeric: true,
),
DataColumn(
label: Text('Role'),
),
],
rows: data
.map(
(item) => DataRow(cells: [
DataCell(Text(item['Name'])),
DataCell(Text(item['Age'].toString())),
DataCell(Text(item['Role'])),
]),
)
.toList(),
),
),
);
}
}
Then you will get the following output.
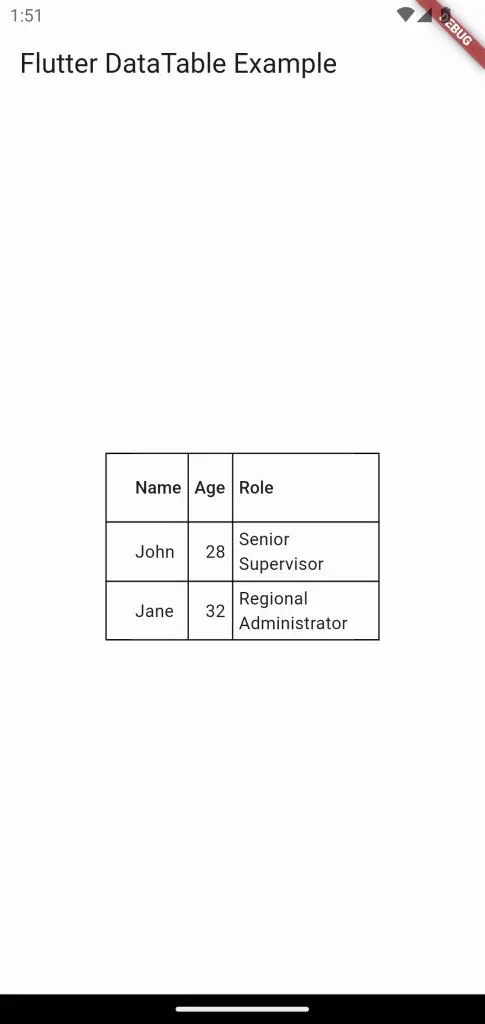
That’s how you adjust the Flutter DataTable column width.