How to add Text with All Caps in Flutter
Text is one of the most important widgets in Flutter to display text content. Let’s learn how to capitalize Text in Flutter easily.
Even though TextField has a feature to capitalize text, there’s no inbuilt way in the Text widget to make text all caps. Hence, we have to use Dart method toUpperCase() to capitalize text.
See the following code snippet.
var text = 'Flutter text all caps'.toUpperCase();
Text(text,
style: const TextStyle(
fontSize: 18,
color: Colors.red,
)),
As you see, we declared a variable and converted it to upper case using the toUpperCase method. Then we pass the variable to the Text widget as data.
The output is given below.
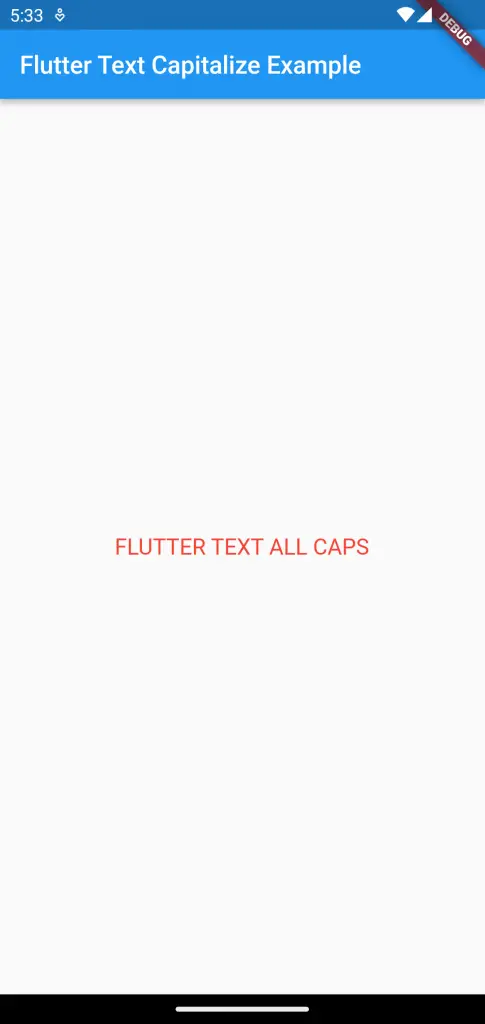
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
var text = 'Flutter text all caps'.toUpperCase();
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Text Capitalize Example'),
),
body: Center(
child: Text(text,
style: const TextStyle(
fontSize: 18,
color: Colors.red,
)),
),
);
}
}
That’s how you show Text in upper case in Flutter.