How to Add Padding to Row in Flutter
Padding is an essential part of designing user interfaces. It adds space between UI elements, making them look less cramped and more aesthetically pleasing. In Flutter, adding padding to a Row
widget can be done in various ways, depending on whether you want to pad the entire Row
or individual children within it.
Let’s explore how to add padding to a Row
in Flutter, enhancing the user interface of your application.
Padding the Entire Row
To add padding around the entire Row
, you can wrap it in a Padding
widget. This approach is useful when you want to inset the Row
from its parent widget.
Example:
const Padding(
padding: EdgeInsets.all(16.0), // Adds padding evenly on all sides
child: Row(
children: [
Icon(Icons.star),
Text('Padded Row'),
],
),
)
Here, EdgeInsets.all
is used to add uniform padding around the entire Row
. You can also use EdgeInsets.symmetric
for vertical and horizontal symmetry, or EdgeInsets.only
to set padding for specific sides.
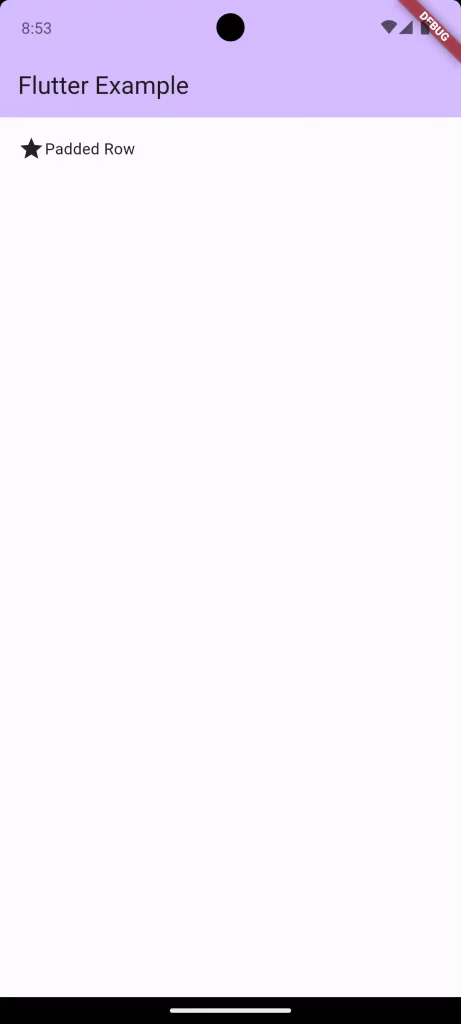
Padding Individual Children
To add space around individual children within a Row
, you have multiple options.
Use Padding Widget
Wrap each child with a Padding
widget if you want to add padding around specific children.
const Row(
children: [
Padding(
padding: EdgeInsets.all(8.0),
child: Text('Item 1'),
),
Padding(
padding: EdgeInsets.all(8.0),
child: Text('Item 2'),
),
],
)
This method allows you to add different padding values to each child.
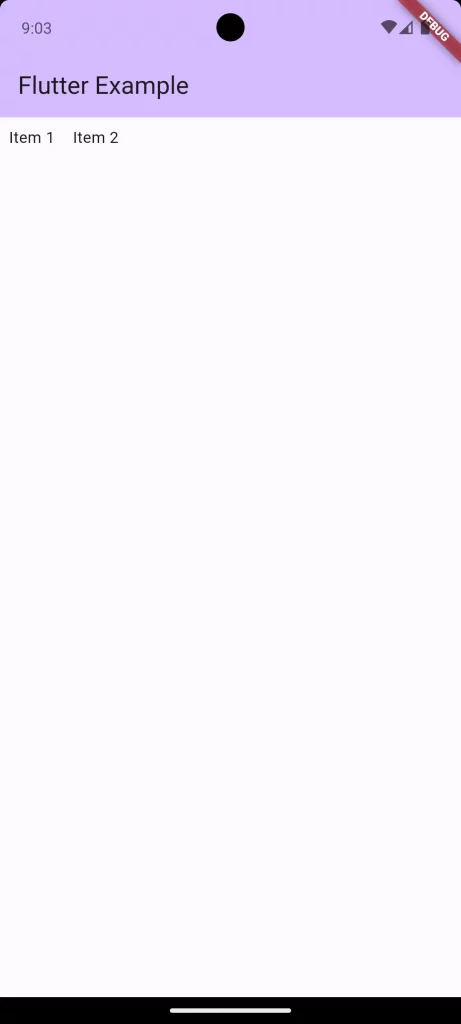
EdgeInsets.fromLTRB
For more granular control, use EdgeInsets.fromLTRB
to specify the padding for left, top, right, and bottom separately for each child.
const Row(
children: [
Padding(
padding: EdgeInsets.fromLTRB(12.0, 8.0, 12.0, 8.0),
child: Text('Item 1'),
),
Padding(
padding: EdgeInsets.fromLTRB(12.0, 8.0, 12.0, 8.0),
child: Text('Item 2'),
),
],
)
Adding padding to a Row
in Flutter is a simple and effective way to create clean and organized layouts. Whether you are padding the entire Row
or individual children, the key is to consider the overall design and user experience.
Padding not only influences the visual impact of the UI but also affects the touch targets, which are crucial for a comfortable user interaction, especially on smaller screens.