How to Change Button Text on Click in Flutter
Sometimes, you may need to change the text label given on button during events such as on press. In this Flutter tutorial, let’s see how to change the button text on click.
We use ElevatedButton in this flutter example. We also use the stateful widget as simple state management is required to change the button text. A variable is declared in the stateful child widget and the variable is changed when the button is pressed using setState. The variable is assigned as Button Text.
See the complete example given below where the Button text is Click Me! and it changes to Loading… when the button is pressed.
import 'package:flutter/material.dart';
void main() => runApp(App());
class App extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter ElevatedButton Shadow Color Example',
home: FlutterExample(),
);
}
}
class FlutterExample extends StatefulWidget {
FlutterExample({Key key}) : super(key: key);
@override
_FlutterExampleState createState() => _FlutterExampleState();
}
class _FlutterExampleState extends State<FlutterExample> {
var buttonText = 'Click Me!';
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('Flutter Change Button Text On Press')),
body: Center(
child: ElevatedButton(
onPressed: () {
setState(() {
buttonText = 'Loading...';
});
},
style: ButtonStyle(
backgroundColor:
MaterialStateProperty.all<Color>(Colors.red)),
child: Text(buttonText))));
}
}
Following is the output.
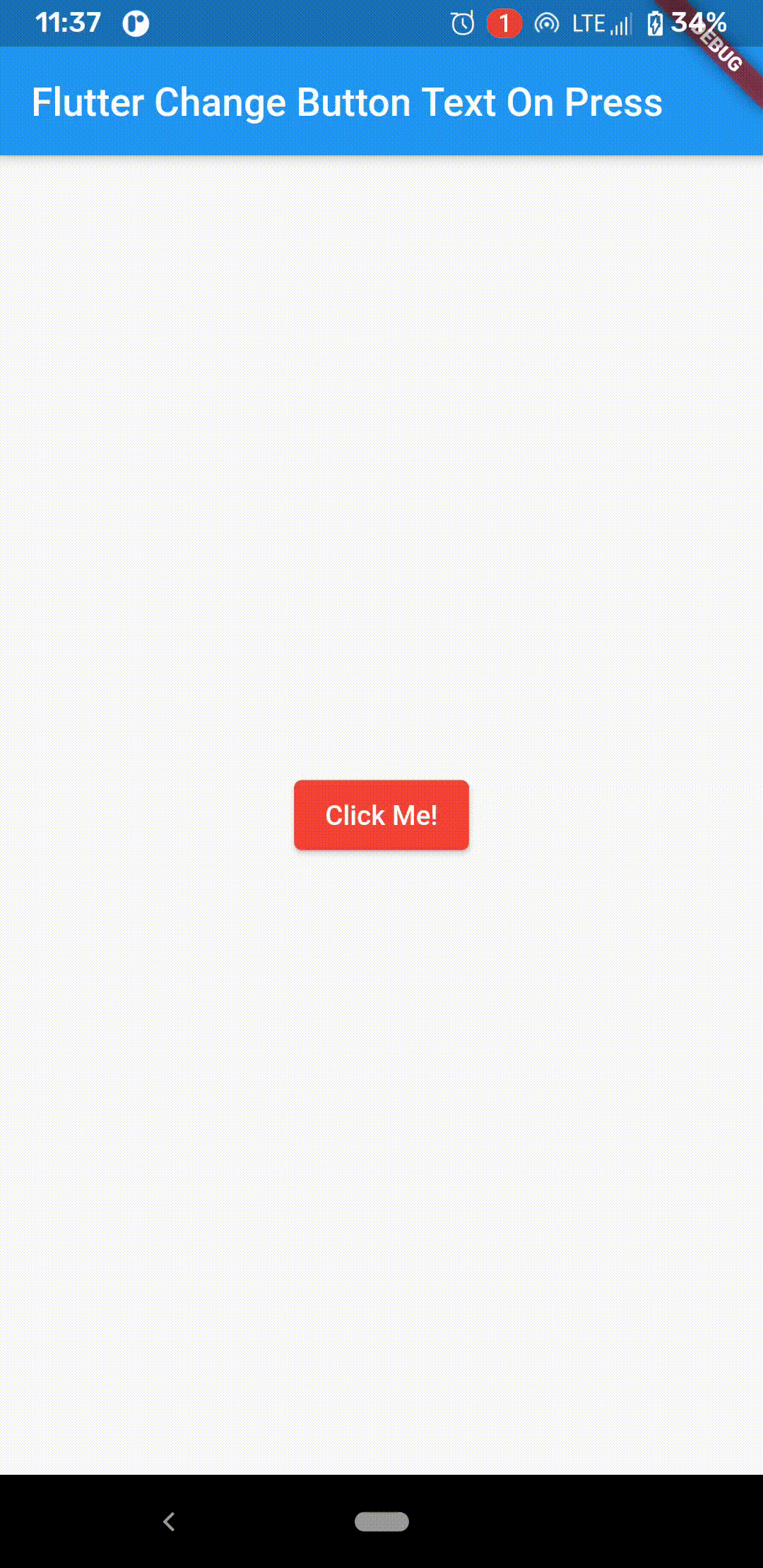
I hope this flutter tutorial to change button text is helpful for you.