How to Center Items in Flutter Row
Creating a balanced and aesthetically pleasing user interface in Flutter often requires centering elements within a row. Whether you’re aiming to place a single item in the middle of the screen or ensure that multiple widgets are centered together, Flutter’s Row
widget provides properties to achieve perfect center alignment.
Row Alignment
The Row
widget in Flutter is a flex container that allows the horizontal arrangement of its child widgets. When it comes to alignment, you have a couple of properties at your disposal:
mainAxisAlignment
: This aligns children along the main axis (horizontally forRow
).crossAxisAlignment
: This aligns children along the cross axis (vertically forRow
).
For centering elements, we primarily focus on mainAxisAlignment
.
Center a Single Child in Row
When you have only one child widget in a Row
and you want to center it horizontally, you use MainAxisAlignment.center
.
Row(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Container(
color: Colors.amber,
child: const Text('Centered Text'),
),
],
)
In this setup, the text within the container will appear centered along the row’s main axis.
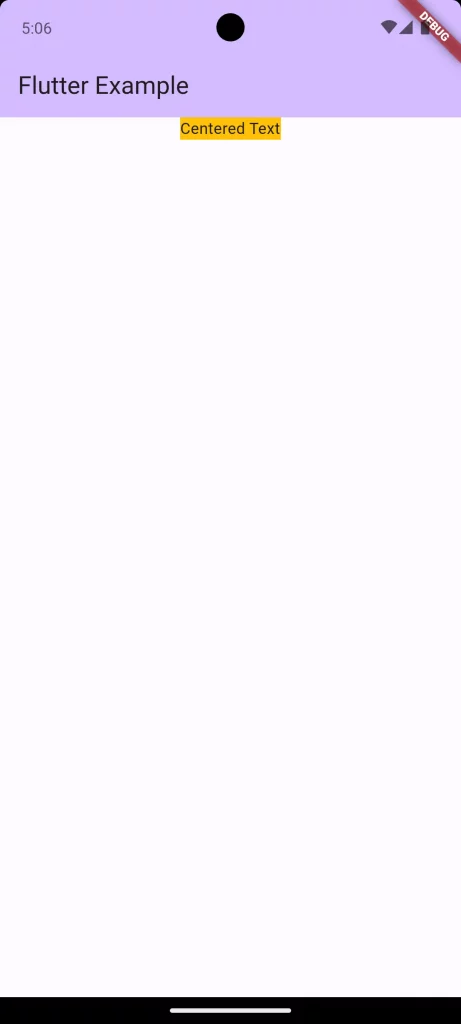
Center Multiple Children in Row
If you have multiple widgets that you want to center together as a group, you again use mainAxisAlignment: MainAxisAlignment.center
.
const Row(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Icon(Icons.star, color: Colors.yellowAccent),
Padding(
padding: const EdgeInsets.all(8.0),
child: Text('Centered Star Rating'),
),
Icon(Icons.star, color: Colors.yellowAccent),
],
)
This configuration centers the star icons and the text together in the middle of the row.
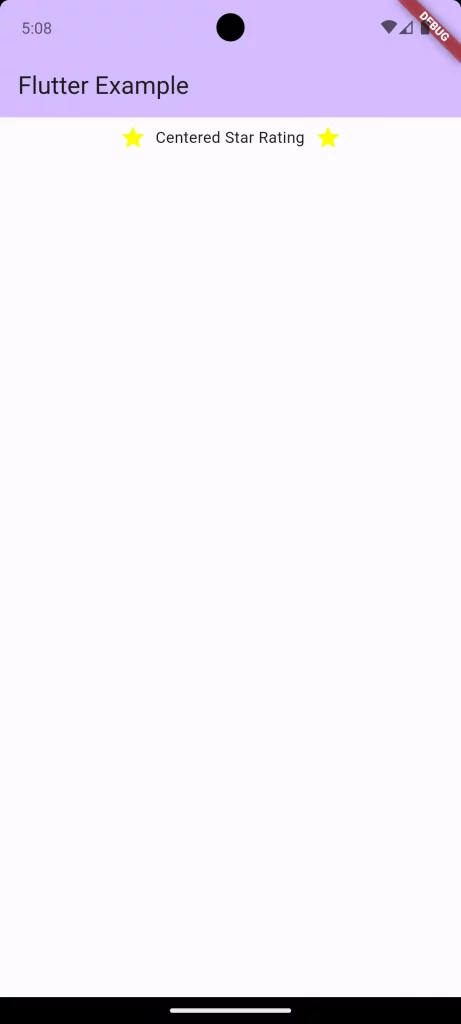
Center with CrossAxisAlignment
Sometimes you may also want to vertically center your row’s children (along the cross axis). This is where crossAxisAlignment
comes into play.
const Row(
mainAxisAlignment: MainAxisAlignment.center,
crossAxisAlignment: CrossAxisAlignment.center,
children: <Widget>[
Text('Vertically and horizontally centered text'),
],
)
This code snippet ensures that the text is centered both horizontally and vertically within the Row
.
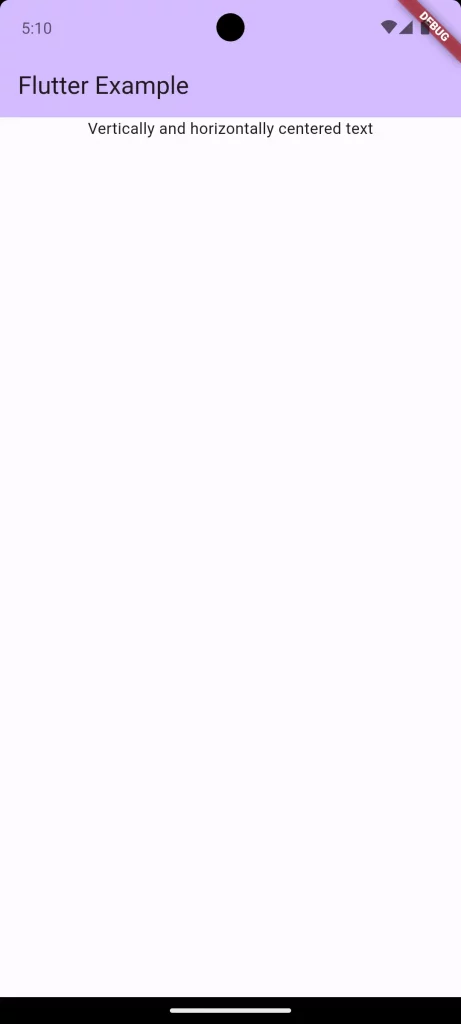
Following is the complete code for reference.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple),
useMaterial3: true,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Theme.of(context).colorScheme.inversePrimary,
title: const Text(
'Flutter Example',
),
),
body: const Row(
mainAxisAlignment: MainAxisAlignment.center,
crossAxisAlignment: CrossAxisAlignment.center,
children: <Widget>[
Text('Vertically and horizontally centered text'),
],
));
}
}
Center alignment within a Row
is a fundamental aspect of Flutter layout design. By using the mainAxisAlignment
and crossAxisAlignment
properties correctly, you can position widgets in the center of the screen or within their parent Row
, achieving a design that is both visually appealing and functionally precise.
Always remember to test your layout on different screen sizes to ensure that the center alignment looks good regardless of the device.