How to make Text Bold in Flutter
The Text widget can be considered as one of the most important widgets in Flutter. Let’s learn how to show bold text in Flutter easily.
The TextStyle class and its property fontWeight help us to make Text bold in Flutter. See the following code snippet.
Text(
'This is Flutter Bold Text Tutorial!',
textAlign: TextAlign.center,
style: TextStyle(fontSize: 18, fontWeight: FontWeight.bold),
)),
The output text will become bold.
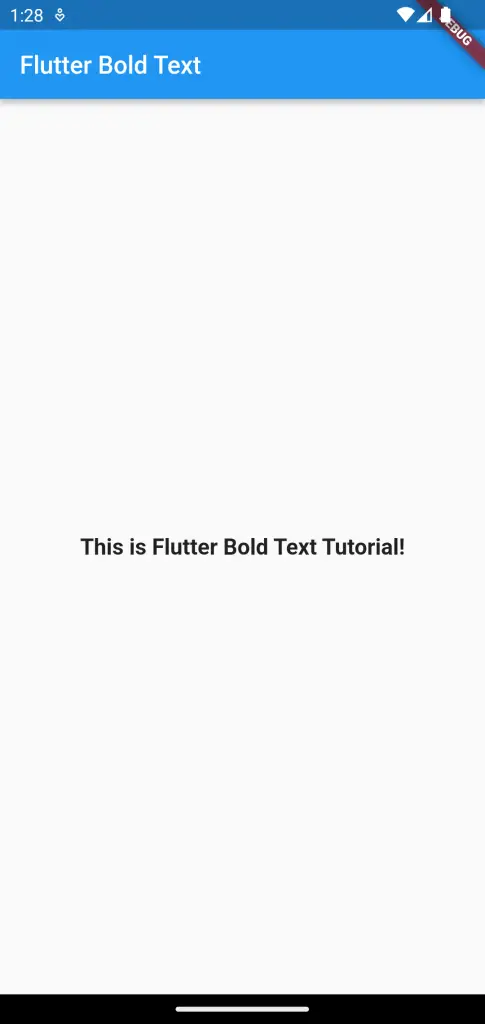
As you see, the whole text becomes bold here. Sometimes, you may only want some specific words to be bold. Then you can make use of Text.rich constructor and TextSpan to get the desired result. See the code snippet given below.
Text.rich(
TextSpan(
text: 'This is a', // default text style
children: <TextSpan>[
TextSpan(
text: ' bold', style: TextStyle(fontWeight: FontWeight.bold)),
TextSpan(
text: ' text!',
style: TextStyle(fontWeight: FontWeight.normal)),
],
),
)
And you will get the following output.
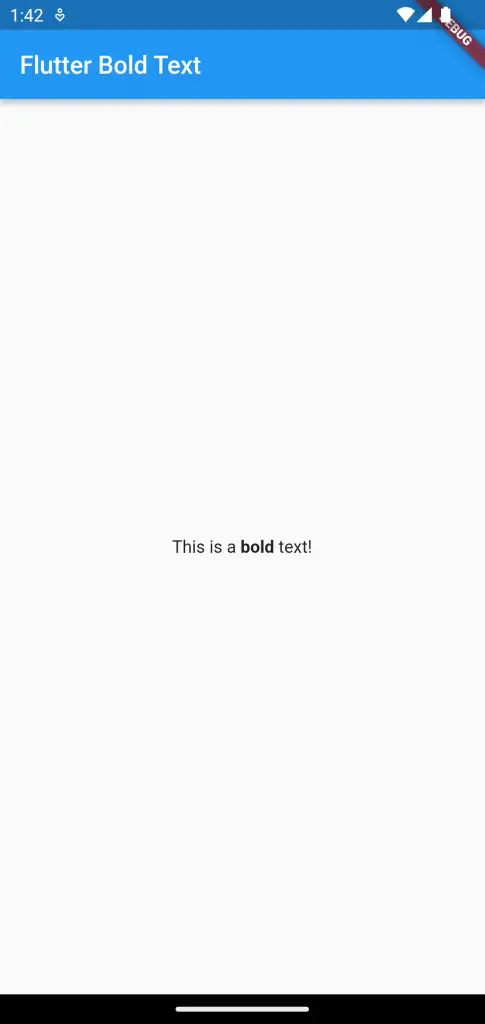
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Bold Text'),
),
body: const Center(
child: Text.rich(
TextSpan(
text: 'This is a', // default text style
children: <TextSpan>[
TextSpan(
text: ' bold', style: TextStyle(fontWeight: FontWeight.bold)),
TextSpan(
text: ' text!',
style: TextStyle(fontWeight: FontWeight.normal)),
],
),
)));
}
}
That’s how you add bold text in Flutter.