How to Create a Slider in Flutter
The slider is an important UI component for mobile apps. It helps users to select a value from a specified range. In this blog post, let’s check how to add a slider component in Flutter.
The Slider class is used to create a slider in Flutter. The slider widget has attributes such as min, max, and divisions to specify the range of values.
We have to use Slider as a part of the stateful widget because when the user changes the value of the slider it should be reflected immediately in the UI. The onChanged is triggered every time the user changes the value of the slider.
See the following code snippet.
Slider(
value: _currentSliderValue,
min: 0,
max: 100,
divisions: 5,
label: _currentSliderValue.round().toString(),
onChanged: (double value) {
setState(() {
_currentSliderValue = value;
});
},
);
Following is the complete example of the Flutter Slider.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Slider Example'),
),
body: const MyStatefulWidget());
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
double _currentSliderValue = 10;
@override
Widget build(BuildContext context) {
return Center(
child: Slider(
value: _currentSliderValue,
min: 0,
max: 100,
divisions: 5,
label: _currentSliderValue.round().toString(),
onChanged: (double value) {
setState(() {
_currentSliderValue = value;
});
},
));
}
}
Following is the output of the flutter slider example.
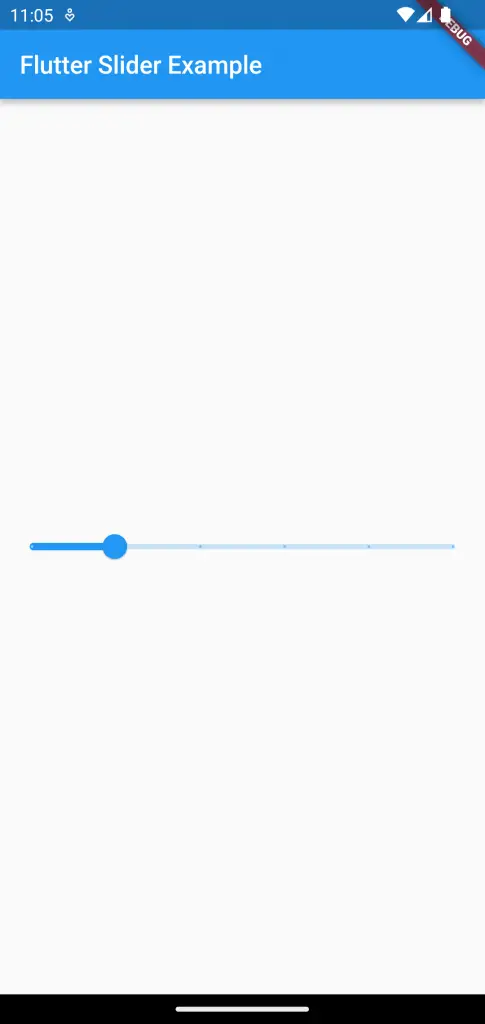
I hope you liked this flutter tutorial.