How to change AppBar Background Color in Flutter
The AppBar is the top most widget that can be seen by the user. The background color of the AppBar is applied according the colors given in ThemeData. In this Flutter tutorial, let’s learn how to change the AppBar background color by overriding the default theme color.
The AppBar widget has a property named backgroundColor and you can change the background color by using it.
AppBar(
backgroundColor: Colors.amber,
title: const Text(
'Flutter AppBar Color',
),
),
Following is the complete code of Flutter AppBar background color example.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Colors.amber,
title: const Text(
'Flutter AppBar Color',
),
),
body: const Center(
child: Text('Flutter AppBar background color tutorial')));
}
}
As you see, the primary color is blue but the backgroundColor overrides the theme color and apply the given yellow color. See the output given below.
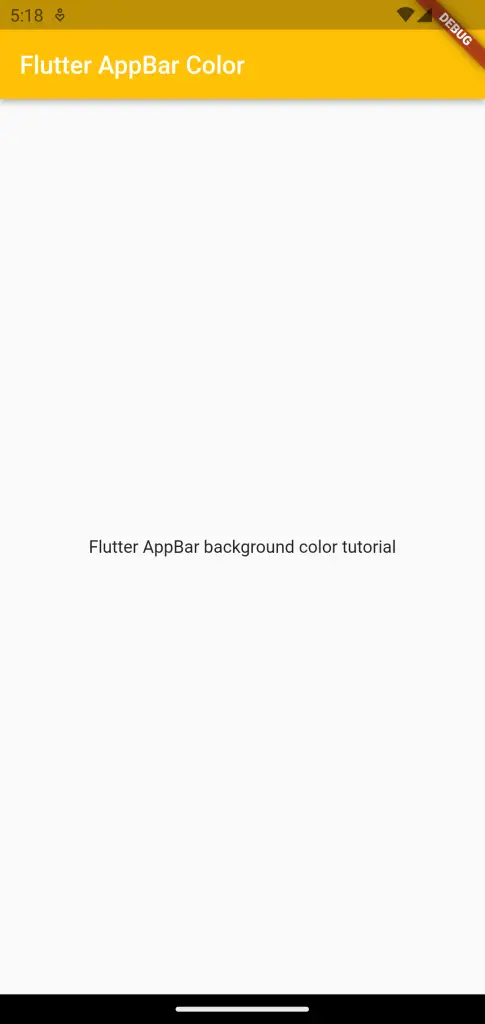
That’s how you change background color of AppBar in Flutter.