How to Create a Horizontal Line in Flutter
Sometimes, you may need to add a horizontal line in your UI. This divider line can help you to separate other components visually. In this flutter tutorial, let’s see how to show a horizontal divider line in Flutter.
There are multiple ways to create a horizontal line. Here, we are using the Divider class. A divider is a thin horizontal line with padding on either side. There are properties such as color, indent, endIndent, height, etc to customize the horizontal line.
const Divider(
color: Colors.black,
height: 25,
thickness: 2,
indent: 5,
endIndent: 5,
),
Following is the complete example where two Expanded widgets are separated by a horizontal line provided by the Divider class.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
'Flutter horizontal line tutorial',
),
),
body: Column(
children: <Widget>[
Expanded(
child: Container(
color: Colors.red,
),
),
const Divider(
color: Colors.black,
height: 25,
thickness: 2,
indent: 5,
endIndent: 5,
),
Expanded(
child: Container(
color: Colors.blue,
),
),
],
),
);
}
}
Following is the output of the flutter example.
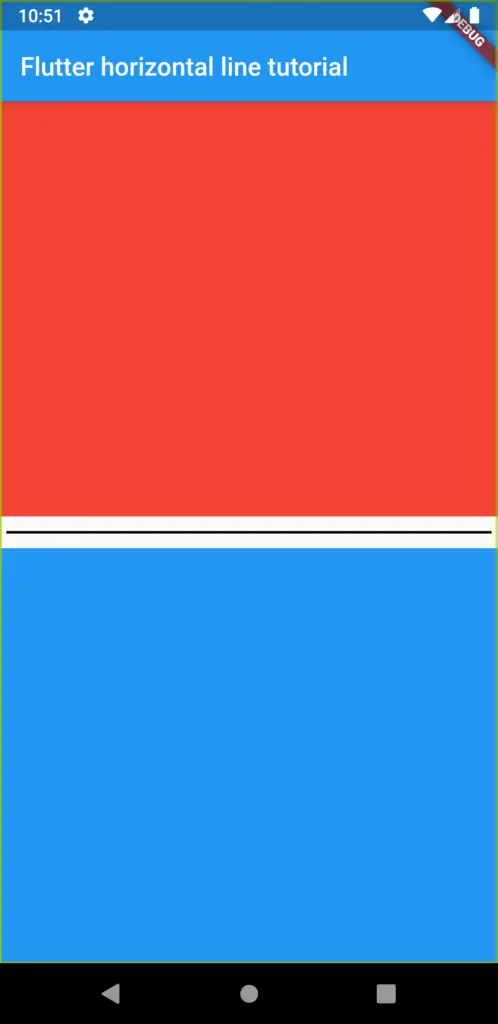
If you want to add a vertical line then refer to this Flutter tutorial.