How to Change DataTable Border Color in Flutter
A well-designed data table can make a big difference in how easily your users can understand and make sense of the information presented. One simple but effective way to enhance the visual appeal of your data table is by changing its border color.
In this blog post, let’s learn how to change the border color of DataTable in Flutter.
The DataTable comes with the border property where we use TableBorder class to draw borders. You can make use of the color property of TableBorder.all constructor to change the border color.
See the code snippet given below.
DataTable(
border: TableBorder.all(width: 1, color: Colors.red),
columns: const <DataColumn>[
DataColumn(
label: Text('Name'),
),
DataColumn(
label: Text('Age'),
numeric: true,
),
DataColumn(
label: Text('Role'),
),
],
rows: List.generate(data.length, (index) {
final item = data[index];
return DataRow(
cells: [
DataCell(Text(item['Name'])),
DataCell(Text(item['Age'].toString())),
DataCell(Text(item['Role'])),
],
);
}),
)
You will get the output of DataTable with red color borders.
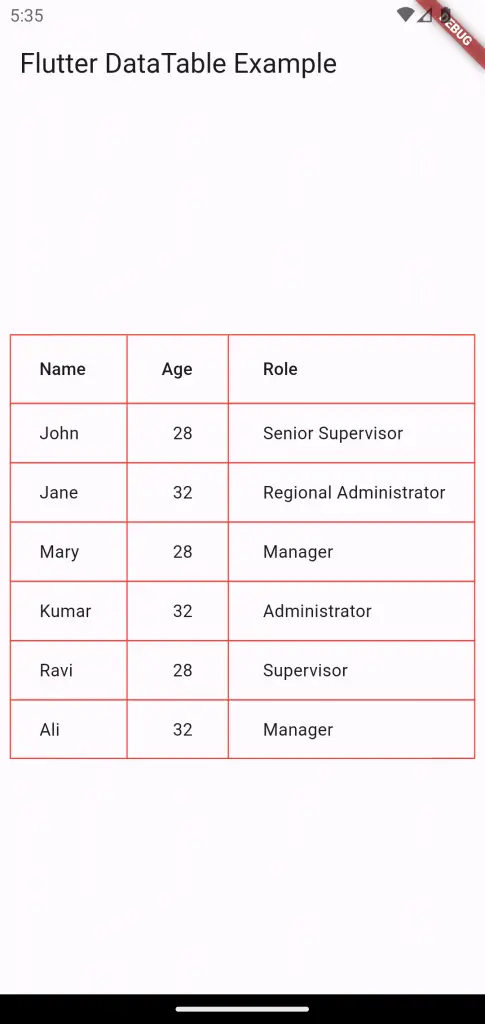
Sometimes, you may want to customize the color of each border. In such cases, you can combine TableBorder and BorderSide classes to get the desired output.
See the code snippet given below.
DataTable(
border: const TableBorder(
top: BorderSide(width: 3, color: Colors.red),
right: BorderSide(width: 3, color: Colors.green),
bottom: BorderSide(width: 3, color: Colors.red),
left: BorderSide(width: 3, color: Colors.green),
verticalInside: BorderSide(width: 1, color: Colors.orange),
horizontalInside: BorderSide(width: 1, color: Colors.blue)),
columns: const <DataColumn>[
DataColumn(
label: Text('Name'),
),
DataColumn(
label: Text('Age'),
numeric: true,
),
DataColumn(
label: Text('Role'),
),
],
rows: List.generate(data.length, (index) {
final item = data[index];
return DataRow(
cells: [
DataCell(Text(item['Name'])),
DataCell(Text(item['Age'].toString())),
DataCell(Text(item['Role'])),
],
);
}),
)
And you will get the following output with colorful borders.
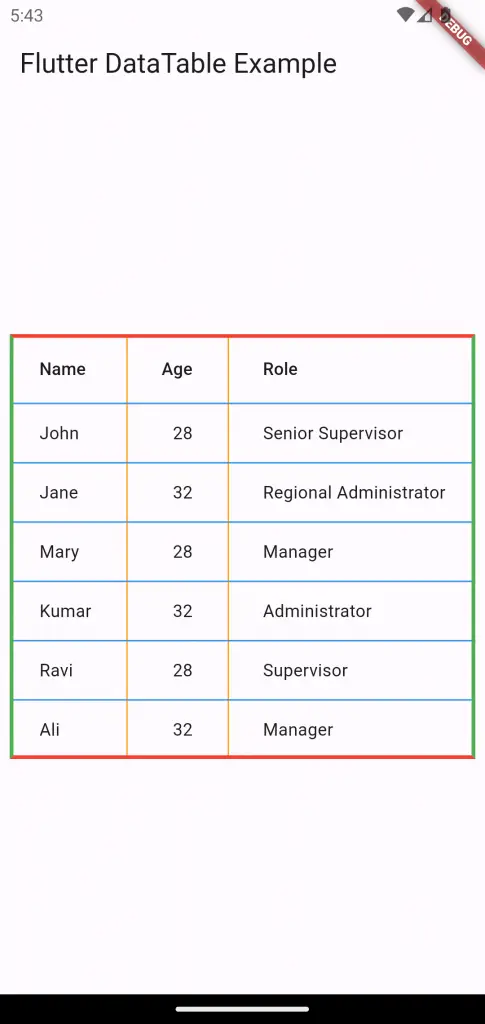
Following is the complete code for reference.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
List<dynamic> data = [
{"Name": "John", "Age": 28, "Role": "Senior Supervisor"},
{"Name": "Jane", "Age": 32, "Role": "Regional Administrator"},
{"Name": "Mary", "Age": 28, "Role": "Manager"},
{"Name": "Kumar", "Age": 32, "Role": "Administrator"},
];
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
useMaterial3: true,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter DataTable Example'),
),
body: Center(
child: DataTable(
border: const TableBorder(
top: BorderSide(width: 3, color: Colors.red),
right: BorderSide(width: 3, color: Colors.green),
bottom: BorderSide(width: 3, color: Colors.red),
left: BorderSide(width: 3, color: Colors.green),
verticalInside: BorderSide(width: 1, color: Colors.orange),
horizontalInside: BorderSide(width: 1, color: Colors.blue)),
columns: const <DataColumn>[
DataColumn(
label: Text('Name'),
),
DataColumn(
label: Text('Age'),
numeric: true,
),
DataColumn(
label: Text('Role'),
),
],
rows: List.generate(data.length, (index) {
final item = data[index];
return DataRow(
cells: [
DataCell(Text(item['Name'])),
DataCell(Text(item['Age'].toString())),
DataCell(Text(item['Role'])),
],
);
}),
),
),
);
}
}
I hope this Flutter DataTable border color tutorial is helpful for you.