How to Display DataTable with Alternate Row Color in Flutter
Adding alternate colors to the rows is not just about aesthetics, it is helpful in improving the overall readability and usability of a table. In this blog post, let’s check how to display a DataTable with alternate row color in Flutter.
The DataTable widget is one of the highly useful widgets in Flutter. We use DataColumn and DataRow to define columns and rows of DataTable.
The DataRow has a color property and it helps us to set the background color of a row.
See the following code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
List<dynamic> data = [
{"Name": "John", "Age": 28, "Role": "Senior Supervisor"},
{"Name": "Jane", "Age": 32, "Role": "Regional Administrator"},
{"Name": "Mary", "Age": 28, "Role": "Manager"},
{"Name": "Kumar", "Age": 32, "Role": "Administrator"},
{"Name": "Ravi", "Age": 28, "Role": "Supervisor"},
{"Name": "Ali", "Age": 32, "Role": "Manager"},
];
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
useMaterial3: true,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
Color getColor(Set<MaterialState> states) {
return Colors.grey;
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter DataTable Example'),
),
body: Center(
child: DataTable(
border: TableBorder.all(width: 1),
columns: const <DataColumn>[
DataColumn(
label: Text('Name'),
),
DataColumn(
label: Text('Age'),
numeric: true,
),
DataColumn(
label: Text('Role'),
),
],
rows: List.generate(data.length, (index) {
final item = data[index];
return DataRow(
color: index % 2 == 0
? MaterialStateProperty.resolveWith(getColor)
: null,
cells: [
DataCell(Text(item['Name'])),
DataCell(Text(item['Age'].toString())),
DataCell(Text(item['Role'])),
],
);
}),
),
),
);
}
}
The DataTable has multiple DataRow objects that represent the rows of data in the table. The color of each row is set based on the ‘index’ value of the row. If the index is even, the color is set to grey using the getColor function, if not, the color is left as null.
You will get the following output.
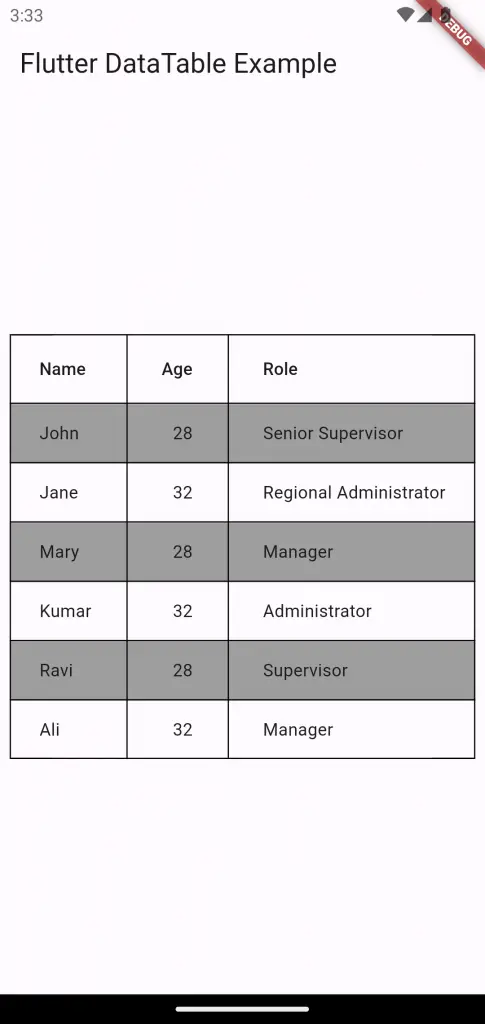
I hope this Flutter DataTable alternate row color tutorial is helpful for you.