How to Make DataTable Scrollable in Flutter
DataTables are an essential component for organizing and displaying large amounts of data in a clean and user-friendly manner. However, as the amount of data grows, the table can become too large to fit on a single screen.
In this tutorial, let’s learn how to make DataTable widget scrollable in Flutter.
By default, the DataTable can’t be scrolled. See the following example.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
List<dynamic> data = [
{"Name": "John", "Age": 28, "Role": "Senior Supervisor"},
{"Name": "Jane", "Age": 32, "Role": "Regional Administrator"},
{"Name": "John", "Age": 28, "Role": "Senior Supervisor"},
{"Name": "Jane", "Age": 32, "Role": "Regional Administrator"},
{"Name": "John", "Age": 28, "Role": "Senior Supervisor"},
{"Name": "Jane", "Age": 32, "Role": "Regional Administrator"},
{"Name": "John", "Age": 28, "Role": "Senior Supervisor"},
{"Name": "Jane", "Age": 32, "Role": "Regional Administrator"},
{"Name": "John", "Age": 28, "Role": "Senior Supervisor"},
{"Name": "Jane", "Age": 32, "Role": "Regional Administrator"},
{"Name": "John", "Age": 28, "Role": "Senior Supervisor"},
{"Name": "Jane", "Age": 32, "Role": "Regional Administrator"},
{"Name": "John", "Age": 28, "Role": "Senior Supervisor"},
{"Name": "Jane", "Age": 32, "Role": "Regional Administrator"},
{"Name": "John", "Age": 28, "Role": "Senior Supervisor"},
{"Name": "Jane", "Age": 32, "Role": "Regional Administrator"},
{"Name": "John", "Age": 28, "Role": "Senior Supervisor"},
{"Name": "Jane", "Age": 32, "Role": "Regional Administrator"},
];
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
useMaterial3: true,
colorSchemeSeed: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter DataTable Example'),
),
body: DataTable(
border: TableBorder.all(width: 1),
columns: const <DataColumn>[
DataColumn(
label: Text('Name'),
),
DataColumn(
label: Text('Age'),
numeric: true,
),
DataColumn(
label: Text('Role'),
),
],
rows: data
.map(
(item) => DataRow(cells: [
DataCell(Text(item['Name'])),
DataCell(Text(item['Age'].toString())),
DataCell(Text(item['Role'])),
]),
)
.toList(),
),
);
}
}
You will get the following output but you can’t scroll it.
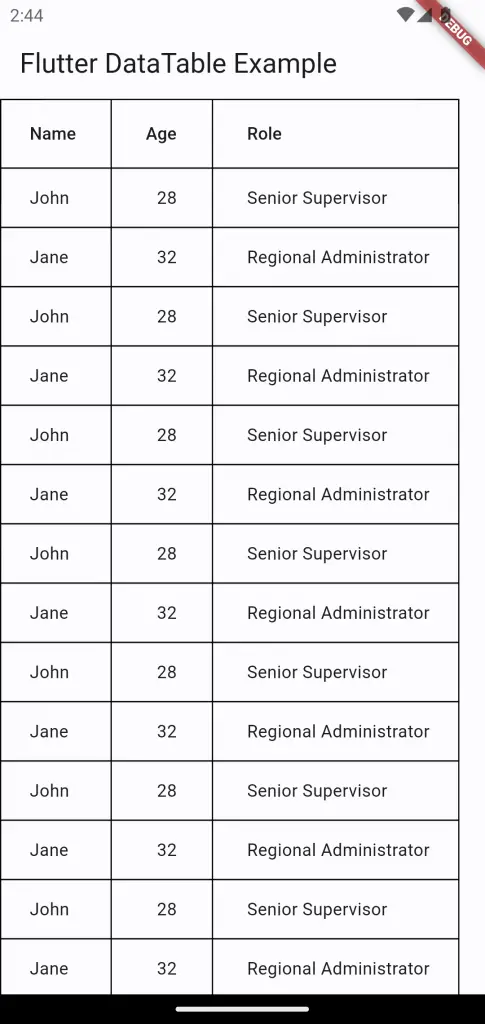
Flutter DataTable Vertical Scrolling
You can add the scrolling functionality by wrapping the Datatable using the SingleChildScrollView widget.
See the code snippet given below.
SingleChildScrollView(
scrollDirection: Axis.vertical,
child: DataTable(
border: TableBorder.all(width: 1),
columns: const <DataColumn>[
DataColumn(
label: Text('Name'),
),
DataColumn(
label: Text('Age'),
numeric: true,
),
DataColumn(
label: Text('Role'),
),
],
rows: data
.map(
(item) => DataRow(cells: [
DataCell(Text(item['Name'])),
DataCell(Text(item['Age'].toString())),
DataCell(Text(item['Role'])),
]),
)
.toList(),
),
)
Now, you can scroll the DataTable vertically.
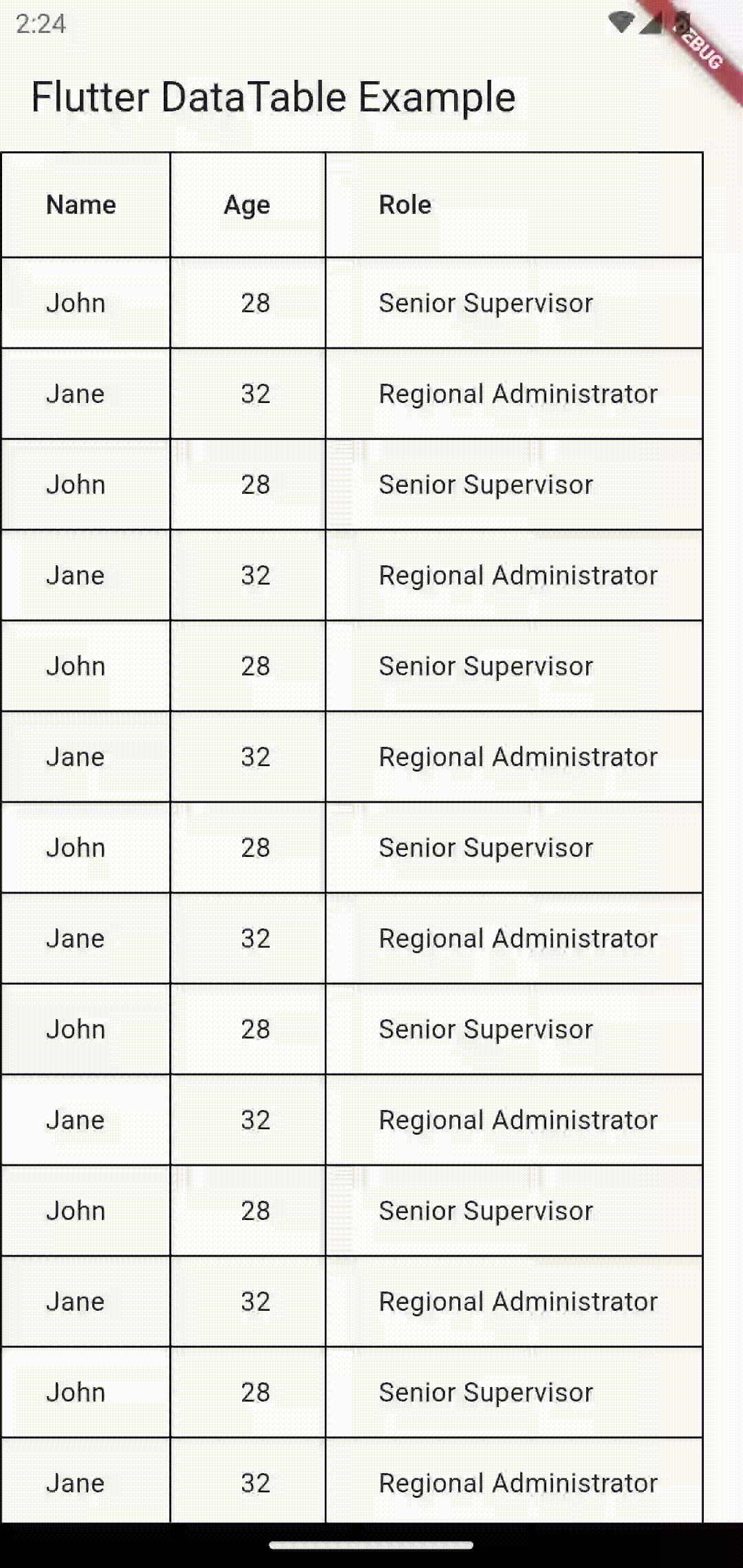
Flutter DataTable Horizontal Scrolling
In the case, you want horizontal scrolling instead of vertical scroll you should set the scrollDirection property of SingleChildScrollView to Axis.horizontal.
We have added an additional column so that you can see horizontal scrolling. See the code snippet given below.
SingleChildScrollView(
scrollDirection: Axis.horizontal,
child: DataTable(
border: TableBorder.all(width: 1),
columns: const <DataColumn>[
DataColumn(
label: Text('Name'),
),
DataColumn(
label: Text('Age'),
numeric: true,
),
DataColumn(
label: Text('Role'),
),
DataColumn(
label: Text('Role'),
),
],
rows: data
.map(
(item) => DataRow(cells: [
DataCell(Text(item['Name'])),
DataCell(Text(item['Age'].toString())),
DataCell(Text(item['Role'])),
DataCell(Text(item['Role'])),
]),
)
.toList(),
),
)
And following is the output.
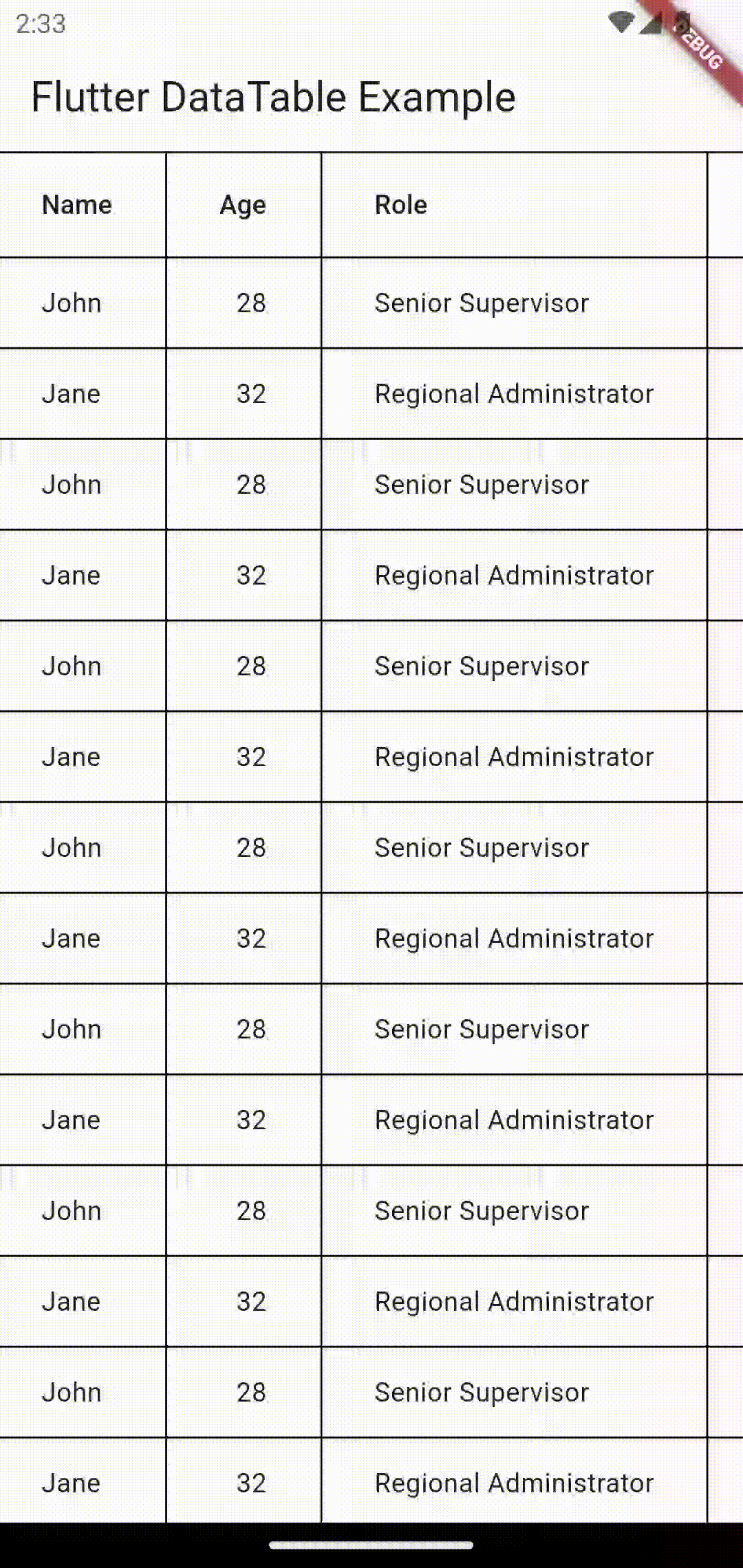
Flutter DataTable Vertical and Horizontal Scrolling
You can make the DataTable scroll bi-directionally. For that, you have to use two SingleChildScrollView widgets.
See the code snippet given below.
SingleChildScrollView(
scrollDirection: Axis.vertical,
child: SingleChildScrollView(
scrollDirection: Axis.horizontal,
child: DataTable(
border: TableBorder.all(width: 1),
columns: const <DataColumn>[
DataColumn(
label: Text('Name'),
),
DataColumn(
label: Text('Age'),
numeric: true,
),
DataColumn(
label: Text('Role'),
),
DataColumn(
label: Text('Role'),
),
],
rows: data
.map(
(item) => DataRow(cells: [
DataCell(Text(item['Name'])),
DataCell(Text(item['Age'].toString())),
DataCell(Text(item['Role'])),
DataCell(Text(item['Role'])),
]),
)
.toList(),
),
),
)
Now, you can scroll the table in both directions.
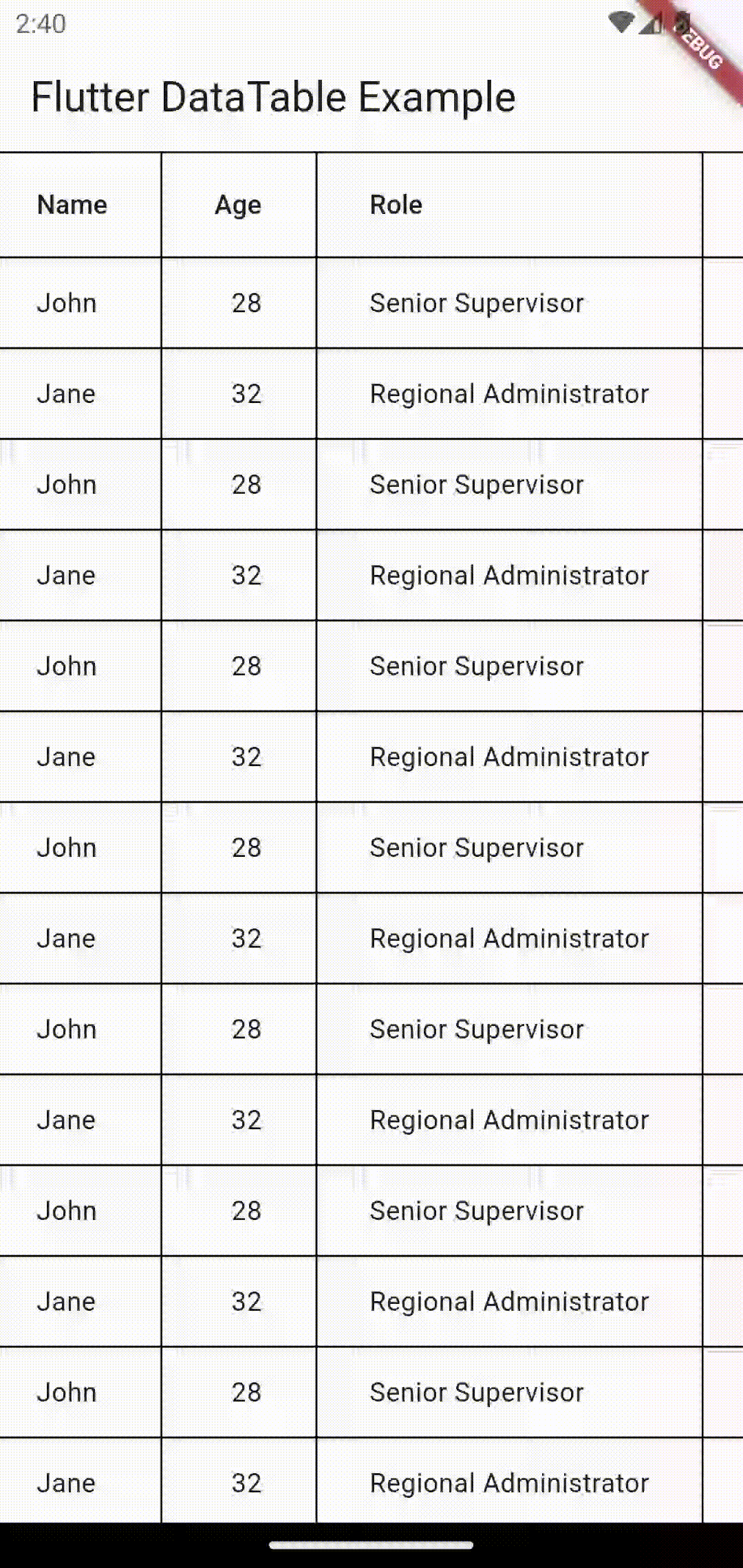
That’s how you make the DataTable scrollable in Flutter.