How to Add Space Between Widgets in Flutter Column
Hey there! If you’re familiar with Flutter, you know how important layout is. One common layout issue developers face is spacing between widgets in a column. In this blog post, I’m going to cover different methods to manage the space between widgets within a Column
in Flutter.
Using mainAxisAlignment
The Code
Here’s how you can use mainAxisAlignment
to manage space:
Column(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
Text('First Child'),
Text('Second Child'),
],
)
Code Explanation
- Column Widget: The
Column
widget is the container for the child widgets. - mainAxisAlignment: By setting
mainAxisAlignment
toMainAxisAlignment.spaceBetween
, we ensure that the maximum space is placed between the widgets.
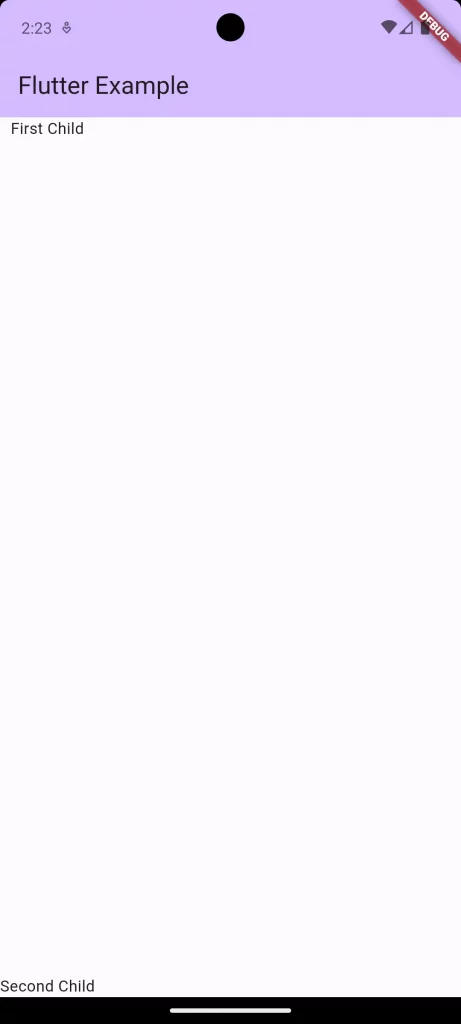
Using Spacer
The Code
Another approach involves using the Spacer
widget:
Column(
children: [
Text('First Child'),
Spacer(),
Text('Second Child'),
],
)
Code Explanation
- Spacer: This widget takes up all available space along the main axis, effectively pushing child widgets apart.
- Column: The
Column
again serves as the container for the children.
Adding Custom Padding
The Code
You can also add custom padding to each widget:
Column(
children: [
Padding(
padding: const EdgeInsets.only(bottom: 20.0),
child: Text('First Child'),
),
Text('Second Child'),
],
)
Code Explanation
- Padding Widget: This widget provides padding to its child widget.
- EdgeInsets.only: Here, we only add padding to the bottom of the first child.
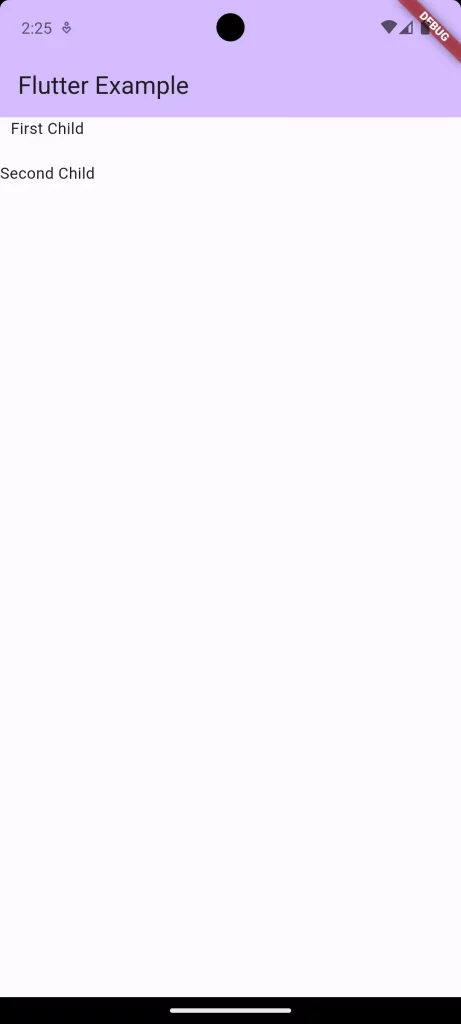
Following is the complete code for reference.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple),
useMaterial3: true,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Theme.of(context).colorScheme.inversePrimary,
title: const Text(
'Flutter Example',
),
),
body: const Column(
children: [
Padding(
padding: EdgeInsets.only(bottom: 20.0),
child: Text('First Child'),
),
Text('Second Child'),
],
));
}
}
Spacing widgets in a Column
in Flutter can be achieved in different ways, each with its advantages and disadvantages. You can use mainAxisAlignment
for a quick solution, Spacer
for more dynamic spacing, or custom Padding
for the most control. Choose the method that best fits your project’s needs!