How to add Shadow to an Icon in Flutter
Icons are important UI elements of a mobile app. As a developer, it’s always important to style icons to increase the visual appeal of the app. In this blog post, let’s learn how to add icon shadow in Flutter.
You can display an icon using the Icon widget. The Icon has a property named shadows and it helps you to create shadows using the Shadow class. You can customize the shadows using properties such as color, blurRadius, etc.
See the code snippet of Icon shadow in Flutter.
Icon(
Icons.verified_user,
color: Colors.teal,
size: 100.0,
shadows: <Shadow>[Shadow(color: Colors.black, blurRadius: 10.0)],
)
Following is the output.
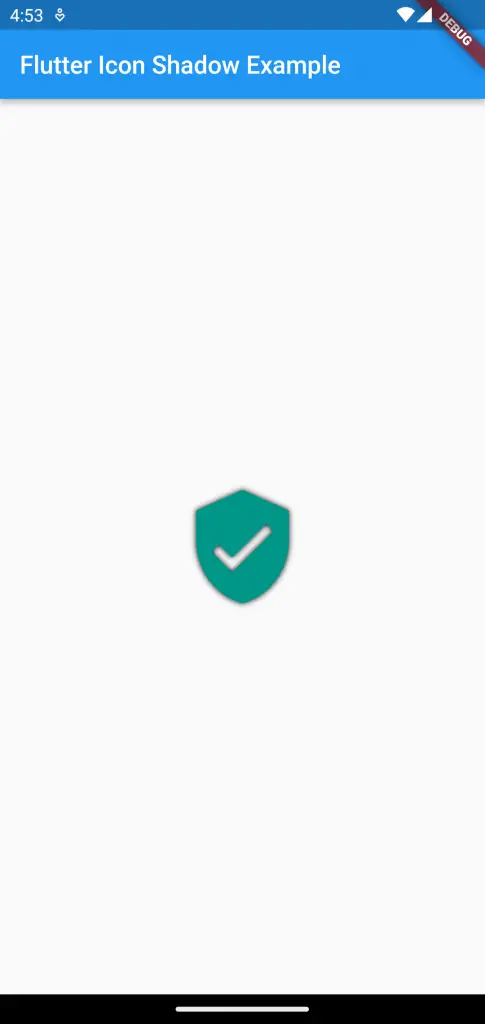
Sometimes, you want to add some offset to the shadows. You can do it using the offset parameter and the Offset class. See the code snippet.
Icon(
Icons.verified_user,
color: Colors.teal,
size: 100.0,
shadows: <Shadow>[
Shadow(color: Colors.black, blurRadius: 10.0, offset: Offset(3, 3))
],
)
Then you will get the following output.
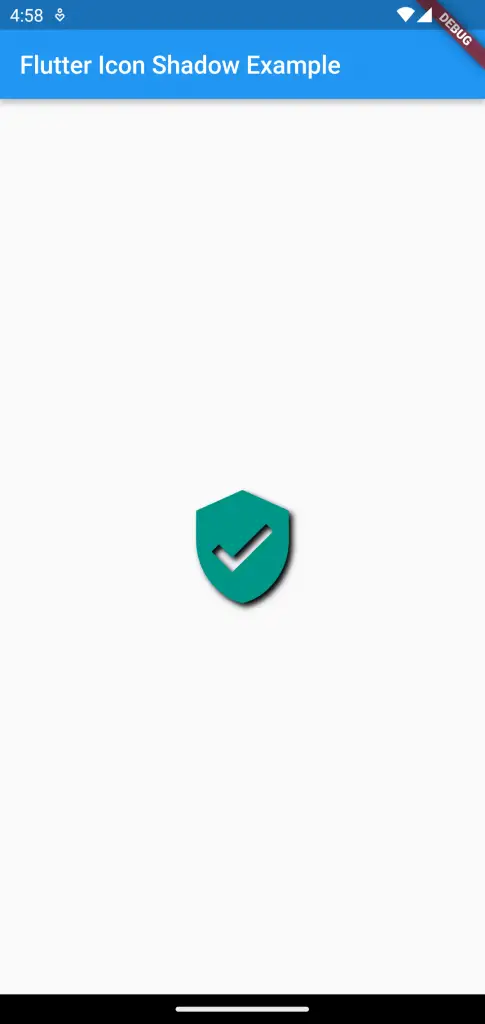
Following is the complete code for reference.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Icon Shadow Example'),
),
body: const Center(
child: Icon(
Icons.verified_user,
color: Colors.teal,
size: 100.0,
shadows: <Shadow>[
Shadow(color: Colors.black, blurRadius: 10.0, offset: Offset(3, 3))
],
)));
}
}
That’s how you add shadows to icons in Flutter.