How to add ListView with Scroll to Top Button in Flutter
ListView content of your mobile app can be dynamic and long. This makes users find it difficult to scroll to the top or bottom of the ListView. In this blog post, let’s learn how to add scroll to the top feature to Flutter ListView.
In order to control the scrolling of ListView, we have to use the ScrollController class. Using its method animateTo you can give the position you want to scroll.
See the following example where I have a Floating Action Button and ListView. When the FAB is pressed, the ListView moves to the top of the screen.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyStatefulWidget(),
);
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
var data = [
"one",
"two",
"three",
"four",
"five",
"six",
"seven",
"eight",
"nine",
"ten"
];
final ScrollController _scrollController = ScrollController();
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter ListView with Scroll to Top'),
),
floatingActionButton: FloatingActionButton(
onPressed: () {
WidgetsBinding.instance.addPostFrameCallback((_) {
if (_scrollController.hasClients) {
_scrollController.animateTo(
_scrollController.position.minScrollExtent,
duration: const Duration(milliseconds: 500),
curve: Curves.fastOutSlowIn);
}
});
},
child: const Icon(Icons.arrow_upward),
),
body: ListView.builder(
controller: _scrollController,
itemCount: data.length,
itemBuilder: (BuildContext context, int index) {
return Container(
height: 150,
color: Colors.white,
child: Center(
child: Text(
data[index],
)),
);
}),
);
}
}
The WidgetsBinding.instance.addPostFrameCallback avoids the system delay. By checking _scrollController.hasClients you ensure no exception will be thrown.
_scrollController.position.minScrollExtent is the given position and the ListView scrolls to the top. If position.minScrollExtent is replaced by position.maxScrollExtent then ListView scrolls to the bottom.
Following is the output of the above code.
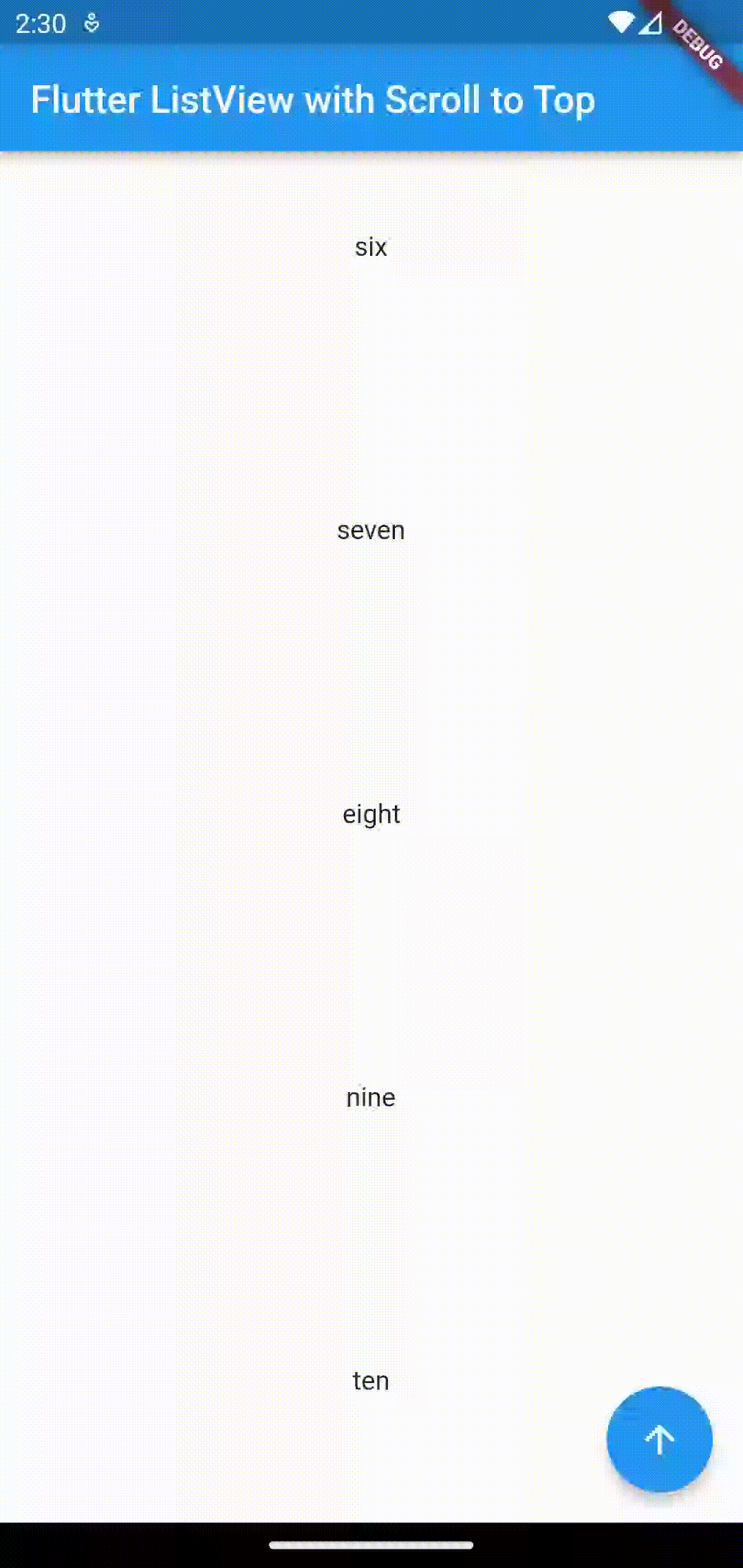
That’s how you add a ListView with a scroll to the top button in Flutter.