How to Add Back Button to WebView in Flutter
In order to provide a better user experience, it is recommended to add a back button to the webview, so that users can navigate back to the previous page easily. In this blog post, let’s learn how to add a WebView with a back button in Flutter.
You can create webview in Flutter using the webview_flutter package. Install the webview package to your Flutter project by following the instructions given here.
Using the webview controller goBack method, you can prompt the webview to go to the previous webpage. See the code snippet given below.
IconButton(
icon: const Icon(Icons.arrow_back),
onPressed: () async {
if (await controller.canGoBack()) {
controller.goBack();
} else {
debugPrint('No history available');
return;
}
},
)
In the similar way, you can also add a forward button to the webview using the goForward method.
It’s always better to add the back button to the AppBar to improve the user experience with the webview.
Following is the complete code to add a webview with a back button in Flutter.
import 'package:flutter/material.dart';
import 'package:webview_flutter/webview_flutter.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
useMaterial3: true,
),
home: const MyStatefulWidget());
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
late final WebViewController controller;
var loadingPercentage = 0;
@override
void initState() {
super.initState();
controller = WebViewController()
..setNavigationDelegate(NavigationDelegate(
onPageStarted: (url) {
setState(() {
loadingPercentage = 0;
});
},
onProgress: (progress) {
setState(() {
loadingPercentage = progress;
});
},
onPageFinished: (url) {
setState(() {
loadingPercentage = 100;
});
},
))
..loadRequest(
Uri.parse('https://flutter.dev'),
);
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter WebView example'),
leading: IconButton(
icon: const Icon(Icons.arrow_back),
onPressed: () async {
if (await controller.canGoBack()) {
controller.goBack();
} else {
debugPrint('No history available');
return;
}
},
)),
body: Stack(
children: [
WebViewWidget(
controller: controller,
),
if (loadingPercentage < 100)
LinearProgressIndicator(
value: loadingPercentage / 100.0,
),
],
));
}
}
Following is the output.
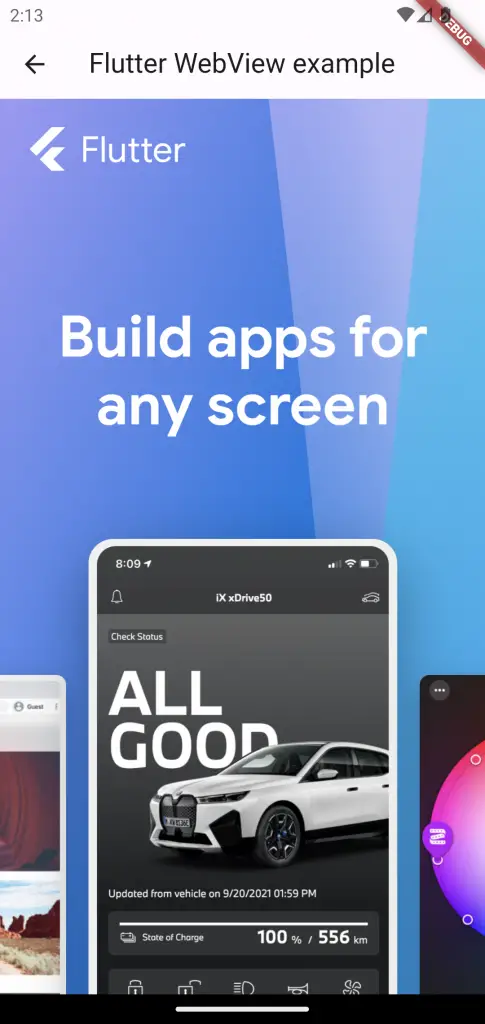
That’s how you add a back button to WebView in Flutter.