How to Sort a List by Date in Flutter
Organizing data is an essential aspect of any app. And the sorting is a fundamental operation for this purpose. In this blog post let’s learn how to sort a List by date in Flutter.
Assume that you have the following List.
List products = [
{'name': 'milk', 'expiry': '2022-11-25'},
{'name': 'egg', 'expiry': '2022-11-23'},
{'name': 'milk', 'expiry': '2022-11-27'},
{'name': 'egg', 'expiry': '2022-11-03'},
];
Let’s change the above List so that the dates will be sorted in descending order. We use the sort method and compareTo method for this purpose.
products.sort((a, b) {
return DateTime.parse(b["expiry"]).compareTo(DateTime.parse(a["expiry"]));
});
debugPrint(products.toString());
As you see we converted the string date to DateTime so that the comparison becomes proper. You will get the output as given below.

We can also sort the List so that the dates will be sorted in ascending order. See the code snippet given below.
products.sort((a, b) {
return DateTime.parse(a["expiry"]).compareTo(DateTime.parse(b["expiry"]));
});
debugPrint(products.toString());
You will get the following output in debug console.

Following is the sample Flutter code.
import 'package:flutter/material.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
useMaterial3: true,
),
home: const MyStatefulWidget());
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
String? sortedProducts;
void sortByDate() {
List products = [
{'name': 'milk', 'expiry': '2022-11-25'},
{'name': 'egg', 'expiry': '2022-11-23'},
{'name': 'milk', 'expiry': '2022-11-27'},
{'name': 'egg', 'expiry': '2022-11-03'},
];
products.sort((a, b) {
return DateTime.parse(b["expiry"]).compareTo(DateTime.parse(a["expiry"]));
});
debugPrint(products.toString());
setState(() {
sortedProducts = products.toString();
});
}
@override
void initState() {
super.initState();
sortByDate();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter List Sort By Date'),
),
body: Center(child: Text('$sortedProducts')));
}
}
Following is the output.
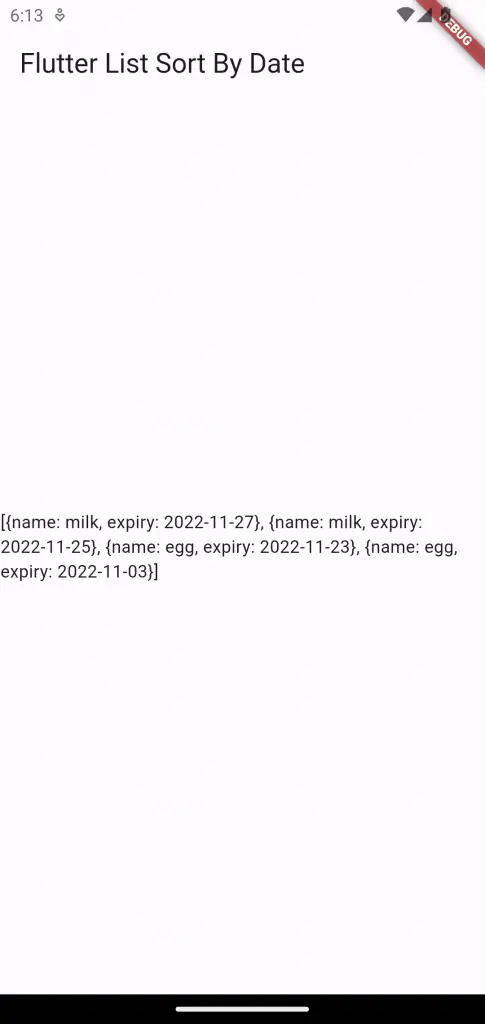
I hope this short tutorial on Flutter sort list by date is useful for you.