How to Calculate Age from a Date in Flutter
A signup form seems incomplete without a field for date of birth. In this blog post, let’s learn how to do age calculation from a given date in Flutter.
We use the date picker here. The age is calculated when the user selects the date. We use the following function to calculate the age in years from a DateTime object.
String calculateAge(DateTime birthDate) {
DateTime currentDate = DateTime.now();
int age = currentDate.year - birthDate.year;
int month1 = currentDate.month;
int month2 = birthDate.month;
if (month2 > month1) {
age--;
} else if (month1 == month2) {
int day1 = currentDate.day;
int day2 = birthDate.day;
if (day2 > day1) {
age--;
}
}
return age.toString();
}
The function takes in a single argument, birthDate, which is a DateTime object representing the date of birth.
The function starts by creating a DateTime object called currentDate that is set to the current date and time using the DateTime.now() method. Then, it calculates the difference in years between the current date and the birth date using currentDate.year – birthDate.year.
Next, the function checks if the birth month is greater than the current month. If so, it decrements the age variable by 1. If the birth month is equal to the current month, it then checks if the birthday is greater than the current day. If so, it also decrements the age variable by 1.
Finally, the function returns the calculated age as a string by converting the age variable to a string using the toString() method.
Following is the complete code where I have TextField and a Text. The date picker appears when you click on the TextField. The age is calculated when a date is chosen and the age will be shown as text.
I have also used the intl flutter package in this example so that the selected date can be displayed in the desired format.
import 'package:flutter/material.dart';
import 'package:intl/intl.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
useMaterial3: true,
),
home: const MyStatefulWidget());
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
final dateController = TextEditingController();
String age = '0';
@override
void dispose() {
// Clean up the controller when the widget is removed
dateController.dispose();
super.dispose();
}
String calculateAge(DateTime birthDate) {
DateTime currentDate = DateTime.now();
int age = currentDate.year - birthDate.year;
int month1 = currentDate.month;
int month2 = birthDate.month;
if (month2 > month1) {
age--;
} else if (month1 == month2) {
int day1 = currentDate.day;
int day2 = birthDate.day;
if (day2 > day1) {
age--;
}
}
return age.toString();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Date Picker Example'),
),
body: Padding(
padding: const EdgeInsets.all(16.0),
child: Center(
child: Column(children: [
TextField(
readOnly: true,
controller: dateController,
decoration: const InputDecoration(hintText: 'Pick your Date'),
onTap: () async {
var date = await showDatePicker(
context: context,
initialDate: DateTime.now(),
firstDate: DateTime(1900),
lastDate: DateTime(2100));
if (date != null) {
setState(() {
age = calculateAge(date);
});
dateController.text = DateFormat('MM/dd/yyyy').format(date);
}
},
),
Text('You are $age years old!')
]))));
}
}
Following is the output.
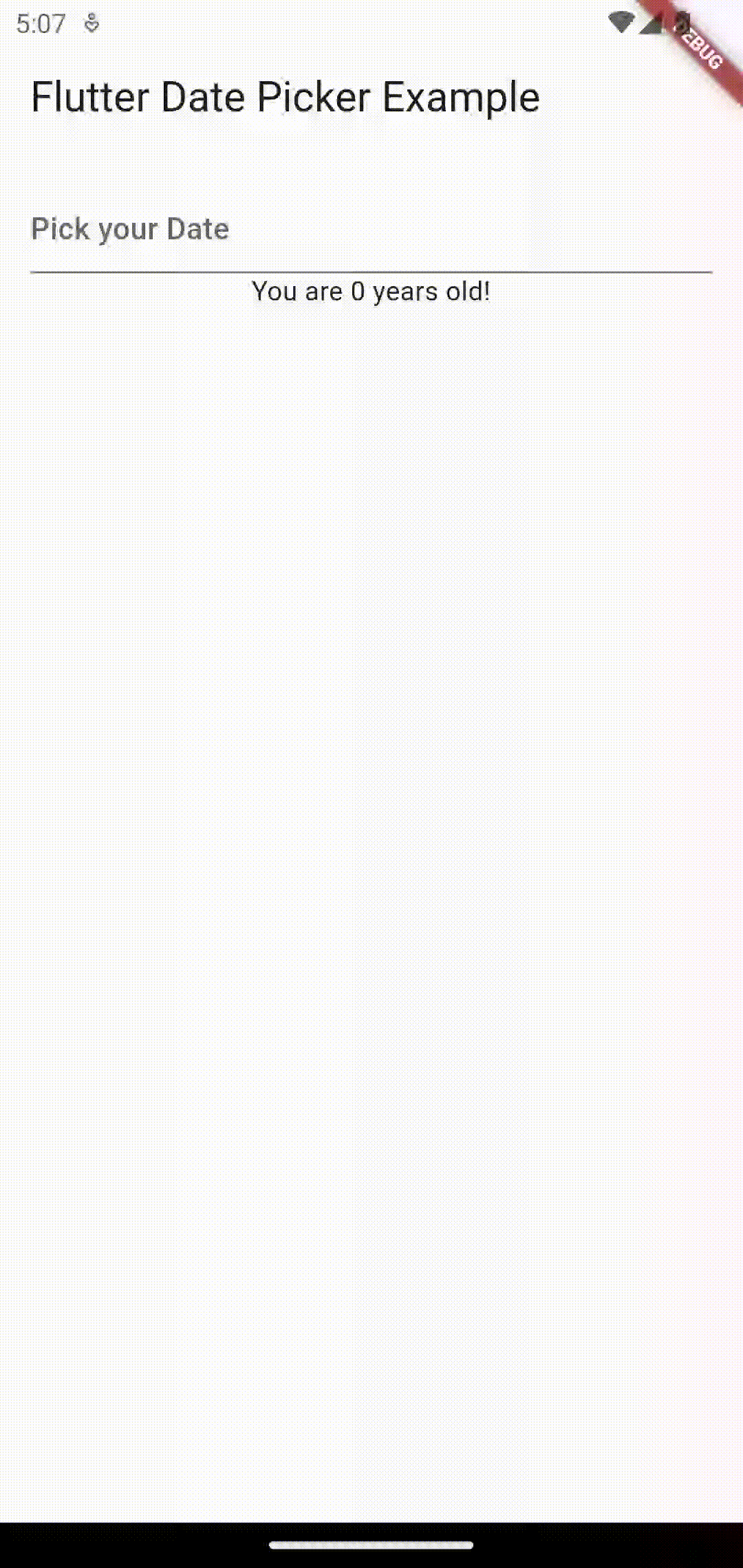
That’s how you calculate age from date of birth in Flutter.