How to Add a Flat Button in Flutter
Update: The FlatButton is deprecated. You should use the OutlinedButton instead.
A Flat Button in Flutter is different from Raised Button. FlatButton widget is basically a text on the material widget which reacts to the touches. Unlike RaisedButton, Flat Button doesn’t have elevation property.
Hence, if you want a button which is not much fancy then you should choose FlatButton over RaisedButton. I have already posted how to add RaisedButton in Flutter. The following Flutter example demonstrates how to add a FlatButton in Flutter.
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Welcome to Flutter',
home: Body()
);
}
}
/// This is the stateless widget that the main application instantiates.
class Body extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Flutter Flat Button Example'),
),
body: Center(
child: FlatButton(
color: Colors.blue,
textColor: Colors.white,
padding: EdgeInsets.all(8.0),
splashColor: Colors.blueAccent,
onPressed: () {},
child: Text(
"Flat Button",
style: TextStyle(fontSize: 20.0),
),
)
),
);
}
}
The following is the output screenshot of the Flutter FlatButton example.
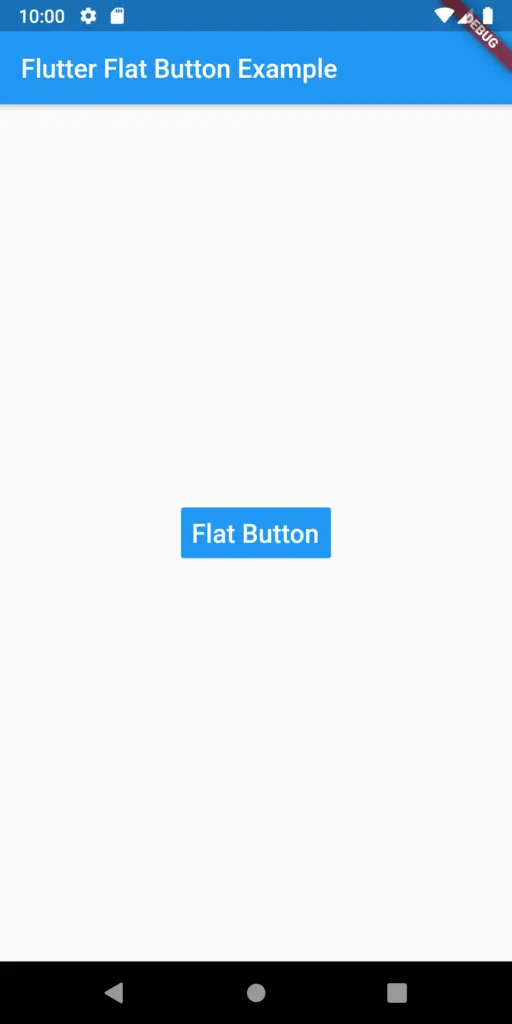