How to Add a Button in Flutter
If you are an absolute beginner in Flutter and don’t know how to add a Button in Flutter then this Flutter beginner tutorial is for you!
The importance of buttons in a mobile app doesn’t need any explanation. Buttons help users with various actions such as login, registration, navigation, etc.
Flutter has different types of buttons – namely ElevatedButton, TextButton, OutlinedButton, etc. In this tutorial, let’s focus more on the ElevatedButton.
The ElevatedButton is a material widget whose elevation increases when the button is pressed. This is what we normally want in our mobile apps with material design.
The syntax of ElevatedButton is the following.
ElevatedButton(
onPressed: () {},
style: ElevatedButton.styleFrom(backgroundColor: Colors.red),
child: const Text(' Elevated Button'))
The ElevatedButton has many useful properties. The onPressed property is used for triggering press actions. Using ElevatedButton.styleFrom method you can style the button.
I hope you already know how to create and run Flutter apps on your PC. Here, I am sharing the code of the main.dart file.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('ElevatedButton Rounded Corner Example'),
),
body: Center(
child: ElevatedButton(
onPressed: () {},
style: ElevatedButton.styleFrom(backgroundColor: Colors.red),
child: const Text(' Elevated Button'))));
}
}
Following is the output of the Flutter Button example:
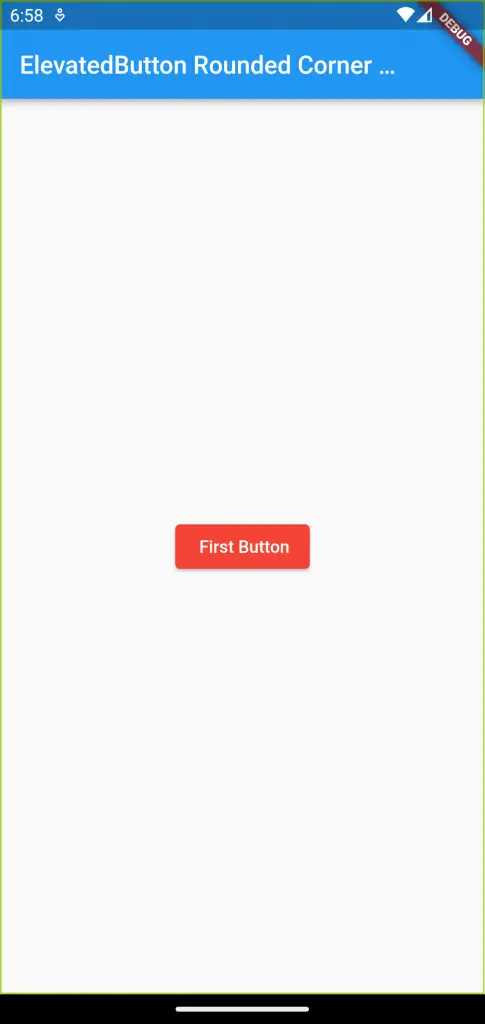
You can customize ElevatedButton according to your requirements. Following blog posts help you to do customizations with the button.
How to add Padding to ElevatedButton
How to create ElevatedButton with icon and text
How to change ElevatedButton color
How to add ElevatedButton border
How to add ElevatedButton with rounded corners
2 Comments