How to Show Alert Dialog in Flutter
Showing alerts for users is an important aspect of a mobile app user interface. In this blog post, I am sharing a Flutter Alert Dialog example with you.
The AlertDialog is a material design widget that informs the user about scenarios that need acknowledgment. It’s also very useful in situations that require confirmation from the user. We can show an optional title and optional actions with it.
Following is the code snippet for Flutter AlertDialog.
AlertDialog(
title: const Text('Alert!'),
content: const Text('This is a simple alert!'),
actions: <Widget>[
TextButton(
onPressed: () => Navigator.pop(context, 'Cancel'),
child: const Text('Cancel'),
),
TextButton(
onPressed: () => Navigator.pop(context, 'OK'),
child: const Text('OK'),
),
],
),
Here, we have AlertDialog with a title, description, and two buttons. The AlertDialog dismisses when any of the buttons is pressed.
Now, go through the complete code given below.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter AlertDialog Example'),
),
body: Center(
child: TextButton(
onPressed: () => showDialog<String>(
context: context,
builder: (BuildContext context) => AlertDialog(
title: const Text('Alert!'),
content: const Text('This is a simple alert!'),
actions: <Widget>[
TextButton(
onPressed: () => Navigator.pop(context, 'Cancel'),
child: const Text('Cancel'),
),
TextButton(
onPressed: () => Navigator.pop(context, 'OK'),
child: const Text('OK'),
),
],
),
),
child: const Text('Show Dialog'),
)));
}
}
Initially, we have a TextButton and the AlertDialog appears when the button is pressed.
Following is the output of the Flutter AlertDialog example.
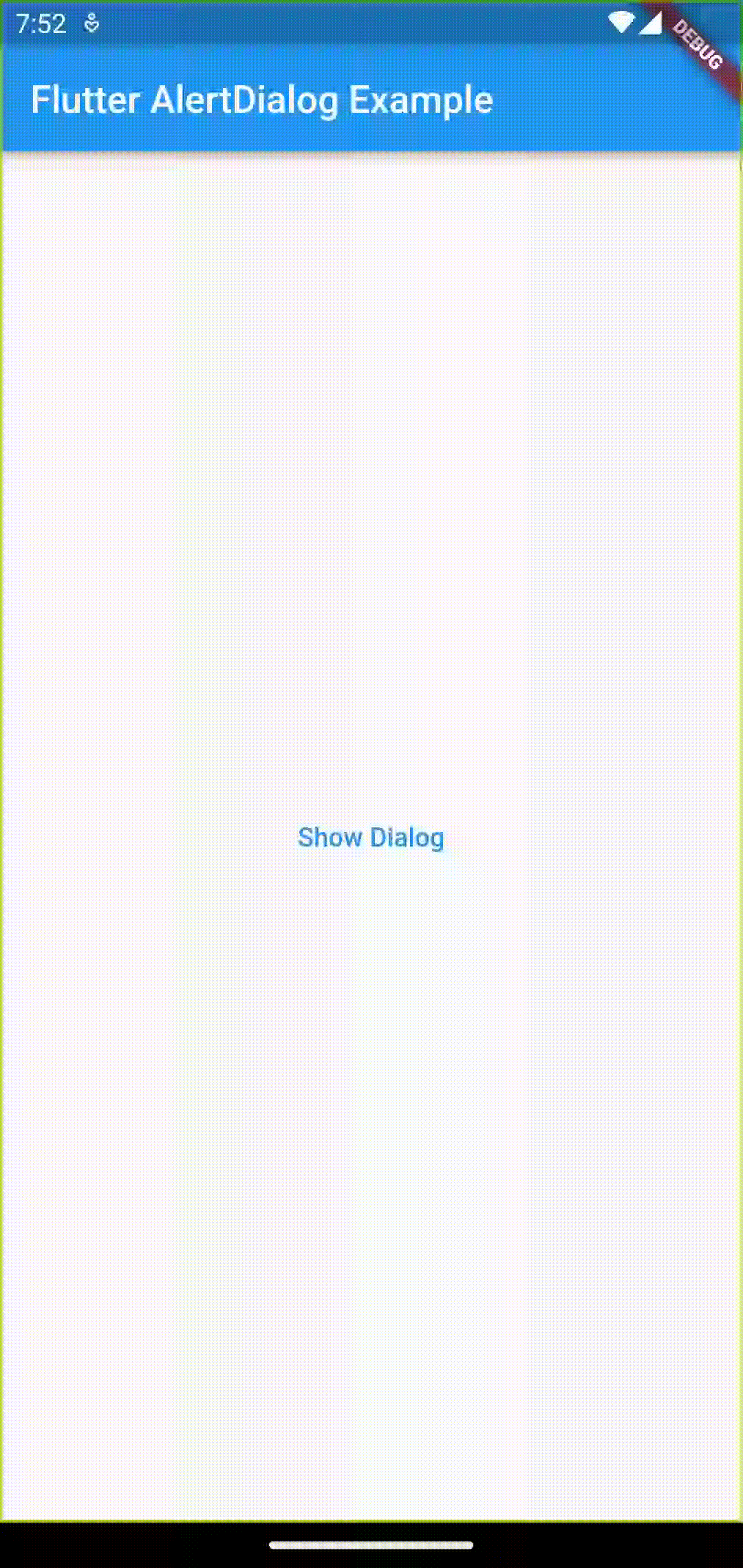
That’s how you add AlertDialog in Flutter.