How to Set Row Background Color in Flutter
In Flutter, a Row
widget is a flexible and widely-used container for laying out a list of widgets in the horizontal direction. One aspect that can significantly affect the look and feel of your app is the background color of your rows.
In this blog post, we’ll go over how to set and customize the background color of a Row
in Flutter.
Why Background Color Matters
The background color can serve several purposes in UI design. It can highlight a section of your app, improve readability, or simply make your app more aesthetically pleasing. In Flutter, setting a background color to a Row
can distinguish it from other sections or make certain content stand out.
Add Background Color to a Row
To set a background color for a Row
widget, you typically wrap it in a Container
widget. This is because the Row
widget itself doesn’t have a property to set the background color directly.
Here’s a simple example of a Row
with a background color:
Container(
color: Colors.lightBlueAccent,
child: const Row(
children: [
Icon(Icons.star, size: 50),
Text('Star-studded Row!', style: TextStyle(fontSize: 24)),
],
),
)
In this code snippet, the Container
‘s color
property sets the background color of the Row
.
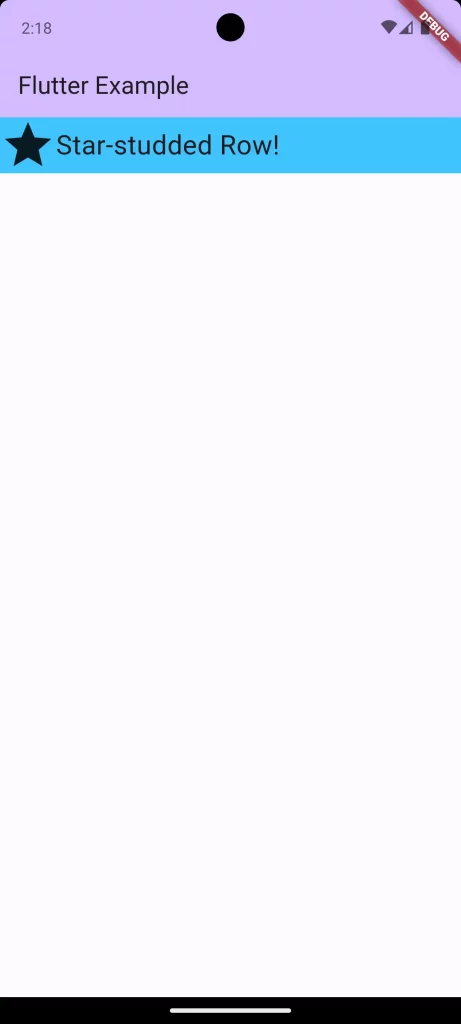
Full-Width Background Color
If you want the background color to stretch the full width of the screen, you should ensure the Row
or its Container
takes up the screen width. Here’s how to do it:
Container(
width: double.infinity,
color: Colors.lightBlueAccent,
child: const Row(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: [
Icon(Icons.star, size: 50),
Text('Star-studded Row!', style: TextStyle(fontSize: 24)),
],
),
)
By setting the width of the Container
to double.infinity
, the background color now stretches across the entire width of the screen.
Following is the complete code for reference.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple),
useMaterial3: true,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Theme.of(context).colorScheme.inversePrimary,
title: const Text(
'Flutter Example',
),
),
body: Container(
width: double.infinity,
color: Colors.lightBlueAccent,
child: const Row(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: [
Icon(Icons.star, size: 50),
Text('Star-studded Row!', style: TextStyle(fontSize: 24)),
],
),
));
}
}
Setting a background color for a Row
in Flutter is an easy and effective way to enhance the design of your app. Using a simple Container
you have the tools to create a beautiful and functional background for your rows.