How to Prevent Row Overflow in Flutter
In Flutter, the Row
widget is fundamental for horizontal layouts. However, when you add more children to a Row
than the available space allows, you will encounter an overflow error.
This is a common issue developers face when building UIs that need to adapt to different screen sizes. Let’s go through some practical solutions to prevent overflow in a Row
.
The Cause of Overflow
Before we look into solutions, it’s crucial to understand why overflow occurs. A Row
widget will attempt to size itself to contain its children. If the combined width of the children is greater than the Row
‘s available space, the excess overflows, usually resulting in a red error banner.
Use Flexible or Expanded
The Flexible
and Expanded
widgets give their child widgets the ability to fill the available space or share it with other widgets. Expanded
is a shorthand for Flexible
with a flex
factor of 1 and tight fitting, meaning the child is forced to fill the available space.
Example with Expanded:
Row(
children: <Widget>[
Expanded(
flex: 1,
child: Container(color: Colors.blue, child: const Text('Item 1')),
),
Expanded(
flex: 2,
child: Container(color: Colors.green, child: const Text('Item 2')),
),
],
)
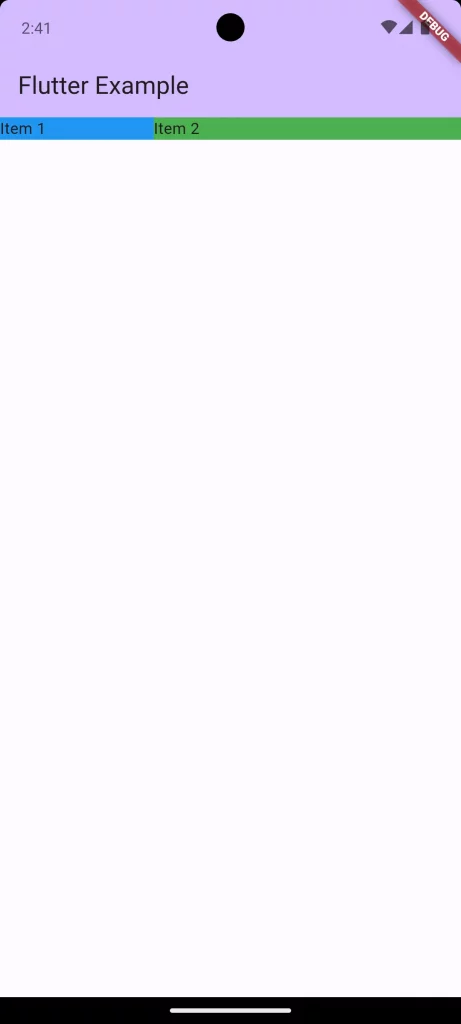
Scroll with SingleChildScrollView
If you want all your widgets to stay in a single line and to be accessible through scrolling, you can use a SingleChildScrollView
.
const SingleChildScrollView(
scrollDirection: Axis.horizontal,
child: Row(
children: <Widget>[
Text('This is the first text.'),
Text('This is the second text.'),
Text('This is the third text.'),
Text('This is the fourth text.'),
],
))
This allows users to scroll through the items in the Row
.
Adjust Child Widget Width
Sometimes, setting a max width for child widgets can prevent overflow by ensuring that no single child takes up too much space.
Row(
children: <Widget>[
ConstrainedBox(
constraints: const BoxConstraints(maxWidth: 100),
child: const Text('A very long item that should be cut off'),
),
const Text('Item 2'),
],
)
Here, the ConstrainedBox
limits the width of its child, preventing overflow.
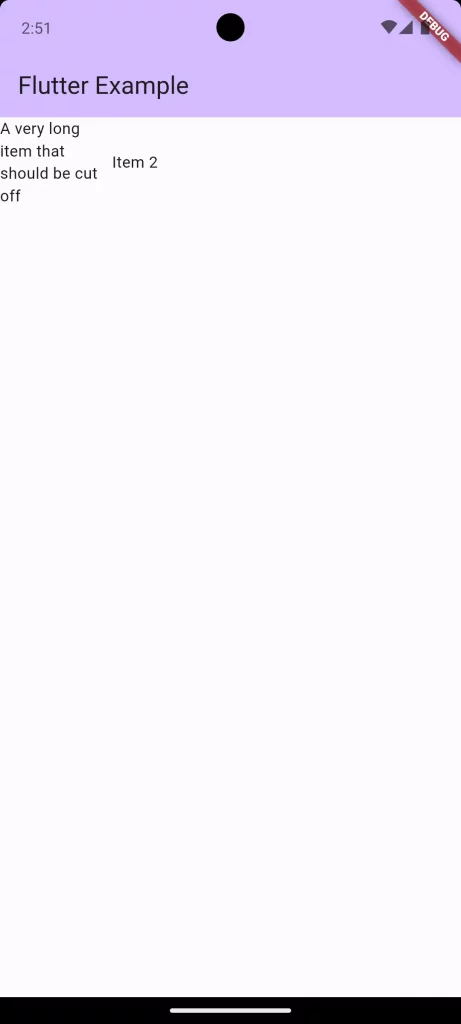
Preventing overflow in a Row
widget in Flutter is all about understanding the available space and how children can be sized or laid out within that space. Whether you choose to make your rows scrollable, wrap onto the next line, or expand within flexible constraints, the key is to test your layouts at various screen sizes and conditions.
By using these practical solutions, you can ensure that your Flutter applications are robust, responsive, and free of overflow errors.
One Comment