How to Create Rounded Corners in Flutter
Rounded corners are beautiful and sometimes outclass components with sharp corners. In this flutter tutorial, let’s check how to create rounded corners.
The ClipRRect widget is used to create rounded corners. It accepts the borderRadius property and we can use it to define border-radius.
Following is the code to create rounded corners.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
'Flutter Rounded Corner Example',
),
),
body: Center(
child: ClipRRect(
borderRadius: BorderRadius.circular(30.0),
child: Container(
color: Colors.red,
width: 200,
height: 200,
),
),
),
);
}
}
And here’s the output.
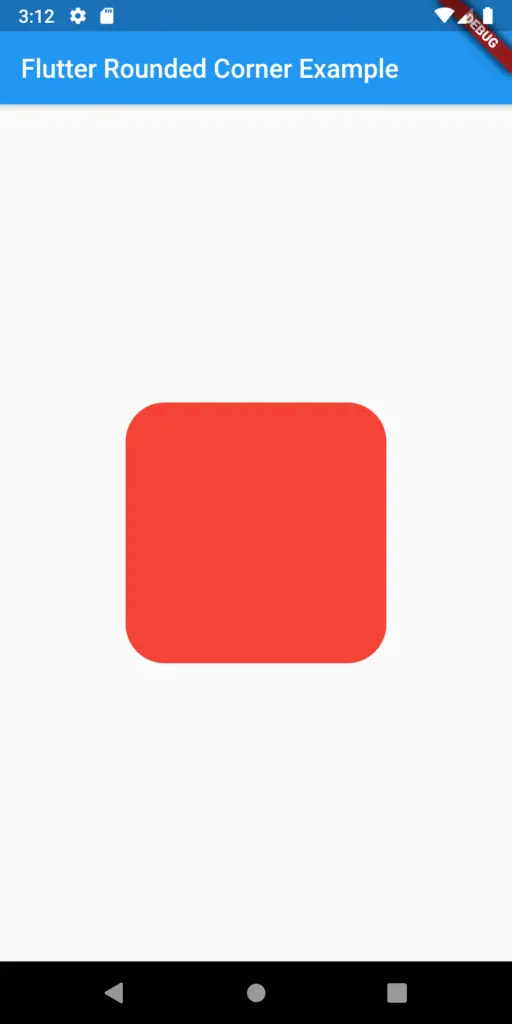
If you want to create oval shapes, then it would be appropriate to use ClipOval class.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
'Flutter Oval Corner Example',
),
),
body: Center(
child: ClipOval(
child: Container(
color: Colors.red,
width: 200,
height: 200,
),
),
),
);
}
}
And following the output of the Flutter example.
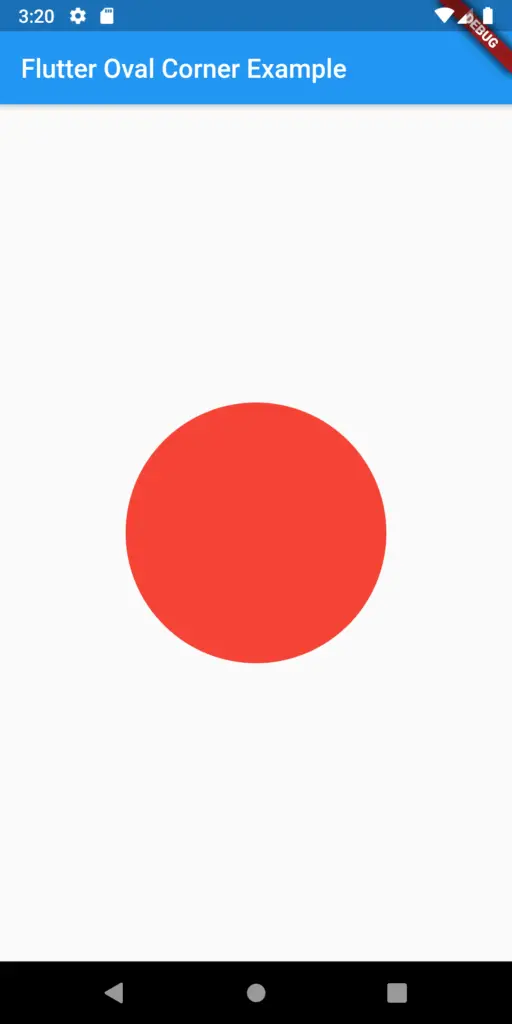
I hope this Flutter tutorial has helped you.