How to Add Hexadecimal Colors in Flutter
By default, you cannot add hexadecimal color code directly to Flutter. In this blog post, let’s check how to add hex color codes in Flutter.
The hexadecimal string is not accepted because the Colors class accepts only integers. Hence, while specifying hexadecimal colors we need to convert them into integers. For this, you just need to append 0xFF in front of the color code. 0xFF is the part that specifies the opacity of the given color.
For example, if I want to use hexadecimal color code #00BCD1 then I should convert it as 0xFF00BCD1. Still confused? Go through the following example where I change the background color of the scaffold widget.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: const Color(0xff00BCD1),
appBar: AppBar(
title: const Text('Flutter Hex Color'),
),
body: const Center(child: Text('codingwithrashid.com')));
}
}
Following is the output.
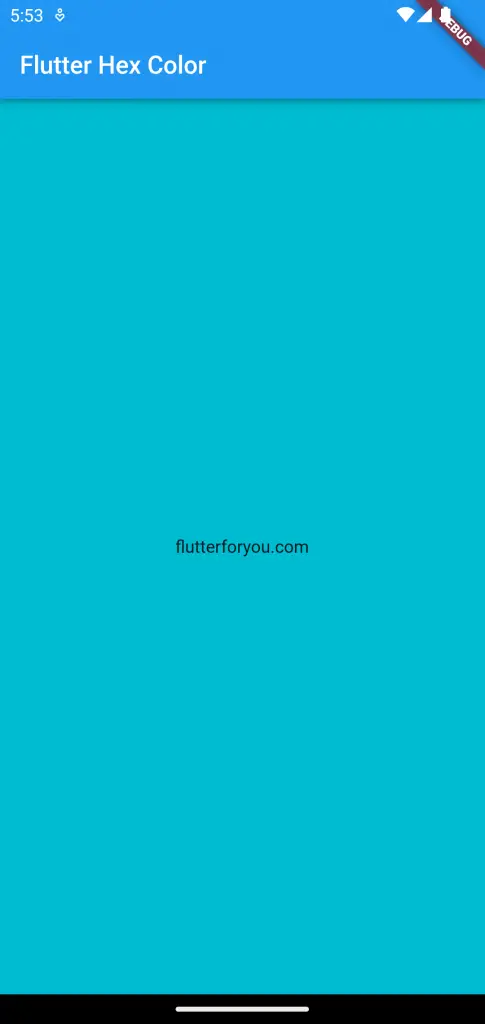
That’s it. I hope now you know how to add hex color codes in Flutter.