How to add ListView with Scroll to Bottom Button in Flutter
The ListView is a highly useful widget in Flutter to display items as a scrollable list. In this tutorial, let’s learn how to add a ListView with a scroll to the bottom button in Flutter.
The ScrollController class helps us to manage the scrolling of the ListView widget. We can make the ListView scroll to the bottom using it.
We have a FAB and a ListView in the following example. When the floating action button is pressed, the ListView scrolls to the bottom.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyStatefulWidget(),
);
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
var data = [
"one",
"two",
"three",
"four",
"five",
"six",
"seven",
"eight",
"nine",
"ten"
];
final ScrollController _scrollController = ScrollController();
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter ListView with Scroll to Bottom'),
),
floatingActionButton: FloatingActionButton(
onPressed: () {
WidgetsBinding.instance.addPostFrameCallback((_) {
if (_scrollController.hasClients) {
_scrollController.animateTo(
_scrollController.position.maxScrollExtent,
duration: const Duration(milliseconds: 500),
curve: Curves.fastOutSlowIn);
}
});
},
child: const Icon(Icons.arrow_downward),
),
body: ListView.builder(
controller: _scrollController,
itemCount: data.length,
itemBuilder: (BuildContext context, int index) {
return Container(
height: 150,
color: Colors.white,
child: Center(
child: Text(
data[index],
)),
);
}),
);
}
}
The post-frame callback should be made for dynamic content. We should also check _scrollController.hasClients to be true. The _scrollController.animateTo method helps to scroll with animation.
The ListView scrolls to the bottom when the position is defined as _scrollController.position.maxScrollExtent.
Following is the output.
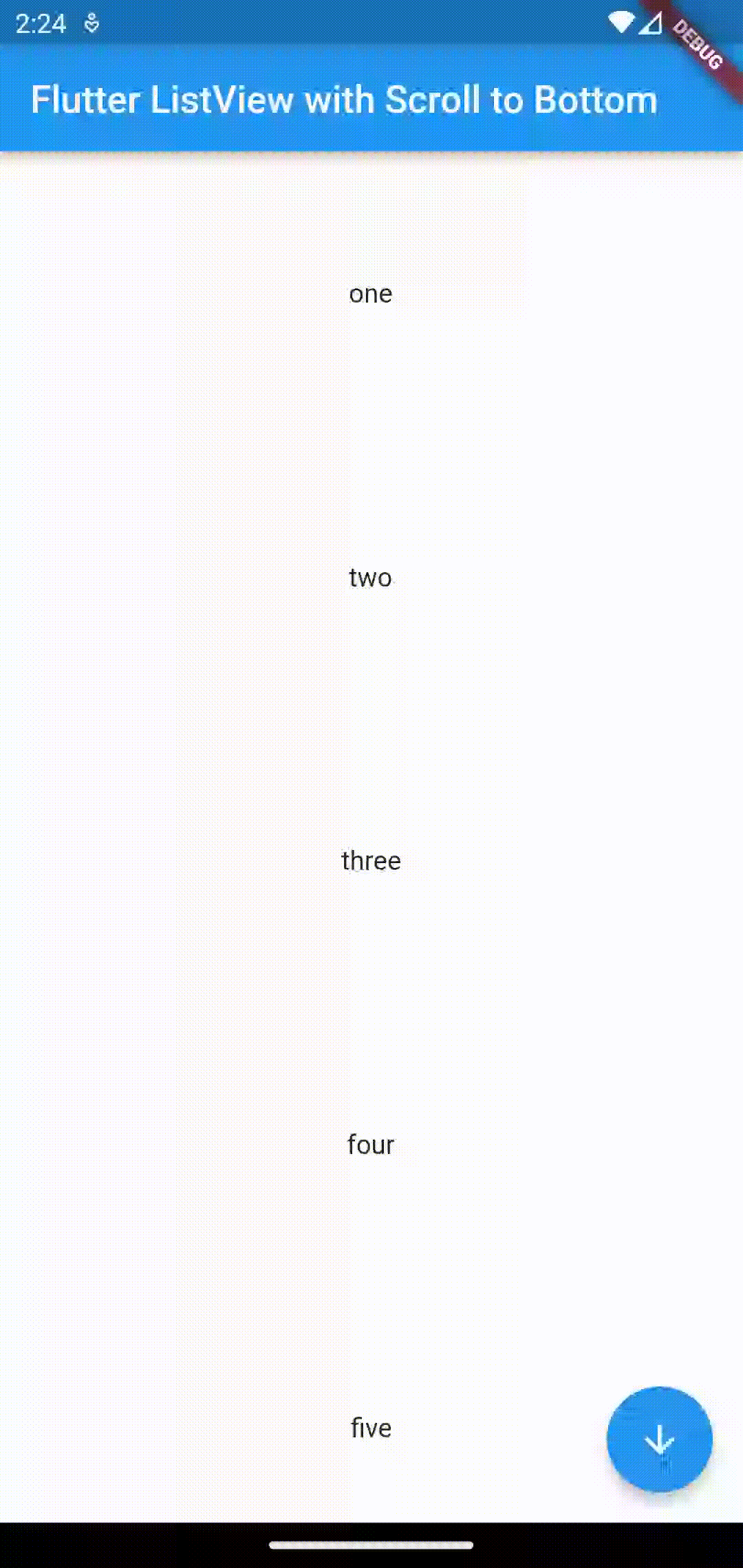
That’s how you add the scroll to the bottom feature in Flutter ListView.