How to Do API Calling in Flutter
Most of the mobile applications we develop have to use internet for various purposes. Hence, let’s learn how to fetch data from APIs in Flutter.
We are using a sample API https://jsonplaceholder.typicode.com/todos/1 that gives the following data in JSON.
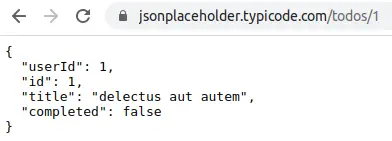
First of all install http package into your Flutter project. Open your pubspec.yaml file and add the dependency as given below.
dependencies:
http: 0.12.2
flutter:
sdk: flutter
You can import the http package as given below.
import 'package:http/http.dart' as http;
As we want to convert the response from API into Dart Object, we create a model class named Data and converts JSON.
class Data {
final int userId;
final int id;
final String title;
final bool completed;
Data({this.userId, this.id, this.title, this.completed});
factory Data.fromJson(Map<String, dynamic> json) {
return Data(
userId: json['userId'],
id: json['id'],
title: json['title'],
completed: json['completed']
);
}
}
We can handle JSON using another ways too. Refer this link for more.
We can fetch the data from API as given below. We use the get method here.
Future<Data> fetchData() async {
final response =
await http.get('https://jsonplaceholder.typicode.com/todos/1');
if (response.statusCode == 200) {
return Data.fromJson(jsonDecode(response.body));
} else {
throw Exception('Unexpected error occured!');
}
}
Using a stateful widget we can display the fetched data. For simplicity, only the title is shown as Text. A progress indicator is shown when the API is loading. See the complete Flutter API example below.
import 'dart:async';
import 'dart:convert';
import 'package:flutter/material.dart';
import 'package:http/http.dart' as http;
Future<Data> fetchData() async {
final response =
await http.get('https://jsonplaceholder.typicode.com/todos/1');
if (response.statusCode == 200) {
return Data.fromJson(jsonDecode(response.body));
} else {
throw Exception('Unexpected error occured!');
}
}
class Data {
final int userId;
final int id;
final String title;
final bool completed;
Data({this.userId, this.id, this.title, this.completed});
factory Data.fromJson(Map<String, dynamic> json) {
return Data(
userId: json['userId'],
id: json['id'],
title: json['title'],
completed: json['completed']
);
}
}
void main() => runApp(MyApp());
class MyApp extends StatefulWidget {
MyApp({Key key}) : super(key: key);
@override
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
Future<Data> futureData;
@override
void initState() {
super.initState();
futureData = fetchData();
}
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter API Example',
home: Scaffold(
appBar: AppBar(
title: Text('Flutter API'),
),
body: Center(
child: FutureBuilder<Data>(
future: futureData,
builder: (context, snapshot) {
if (snapshot.hasData) {
return Text(snapshot.data.title);
} else if (snapshot.hasError) {
return Text("${snapshot.error}");
}
// By default show a loading spinner.
return CircularProgressIndicator();
},
),
),
),
);
}
}
This API example gives you the following output.
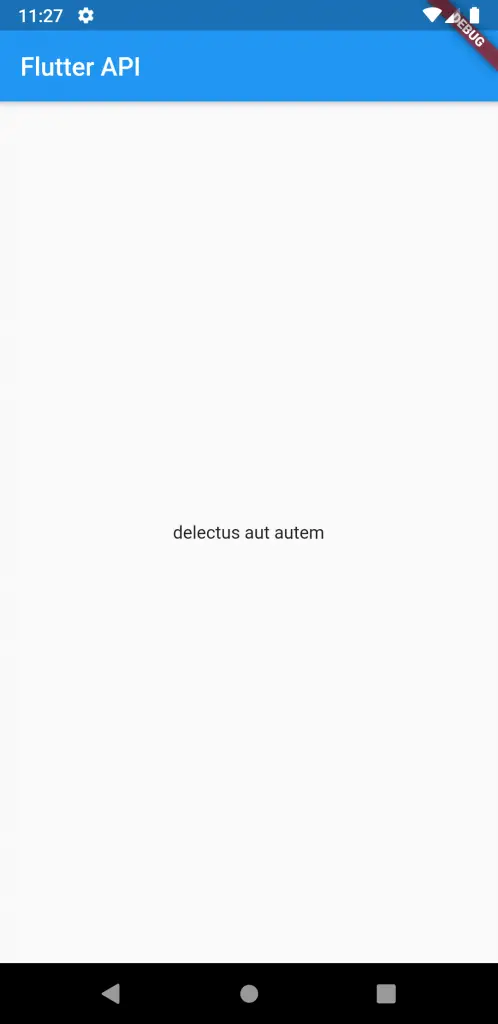
I hope this tutorial on handling APIs in Flutter will be helpful for you.