How to Change DataTable Border Radius in Flutter
Creating visually appealing data tables is an important aspect of user interface design, and adding a touch of customization can make your app stand out. One way to do this is by changing the border radius of your data table.
In this blog post, let’s learn how to change the border radius of DataTable in Flutter.
The DataTable has a border property that helps us to add borders using TableBorder class. You can use the borderRadius property with TableBorder.all constructor.
See the code snippet given below.
DataTable(
border: TableBorder.all(
width: 1,
borderRadius: BorderRadius.circular(20),
columns: const <DataColumn>[
DataColumn(
label: Text('Name'),
),
DataColumn(
label: Text('Age'),
numeric: true,
),
DataColumn(
label: Text('Role'),
),
],
rows: List.generate(data.length, (index) {
final item = data[index];
return DataRow(
cells: [
DataCell(Text(item['Name'])),
DataCell(Text(item['Age'].toString())),
DataCell(Text(item['Role'])),
],
);
}),
)
Then you will get the following output.
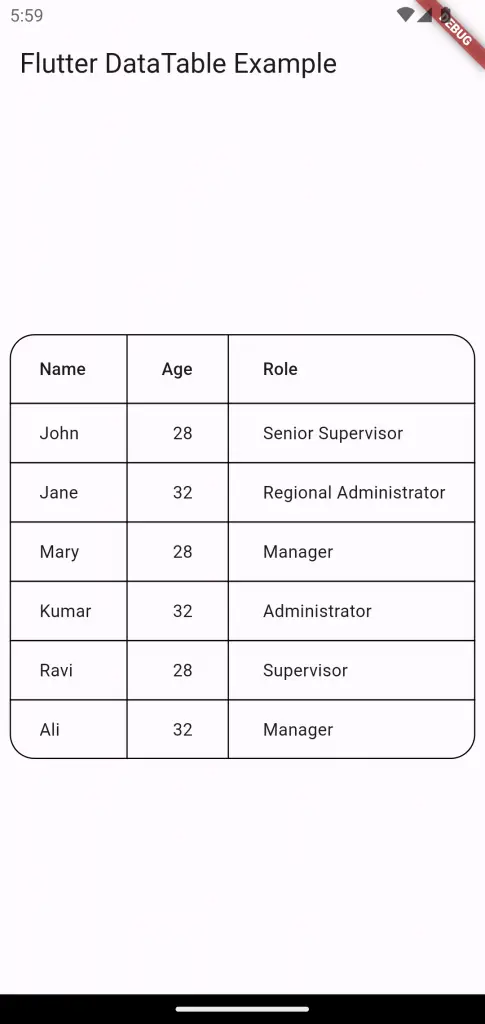
Sometimes, you may want to try different border radii for each corner. In such cases, you can use BorderRadius.only constructor.
See the code snippet given below.
DataTable(
border: TableBorder.all(
width: 1,
borderRadius: const BorderRadius.only(
topLeft: Radius.circular(10),
topRight: Radius.circular(20),
bottomLeft: Radius.circular(30),
bottomRight: Radius.circular(40))),
columns: const <DataColumn>[
DataColumn(
label: Text('Name'),
),
DataColumn(
label: Text('Age'),
numeric: true,
),
DataColumn(
label: Text('Role'),
),
],
rows: List.generate(data.length, (index) {
final item = data[index];
return DataRow(
cells: [
DataCell(Text(item['Name'])),
DataCell(Text(item['Age'].toString())),
DataCell(Text(item['Role'])),
],
);
}),
)
Then you will have a different border radius.
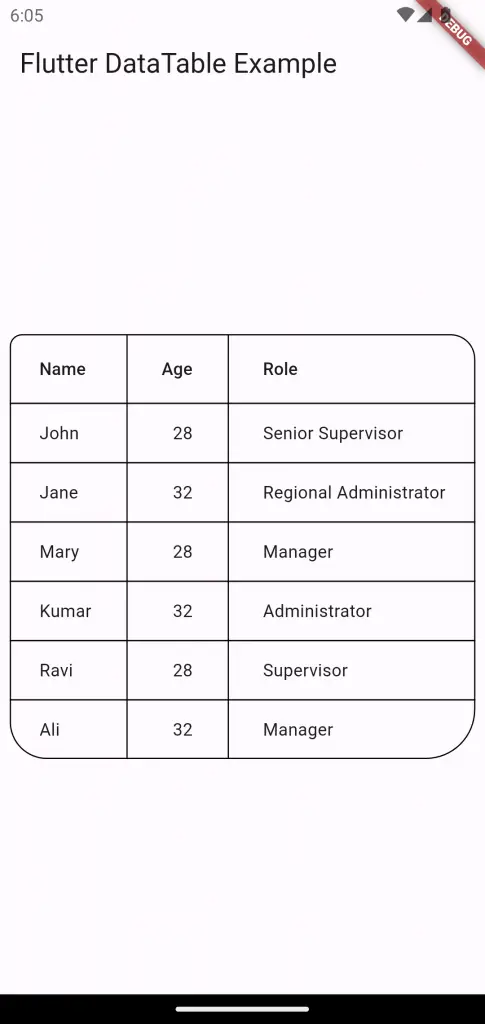
Following is the complete code for reference.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
List<dynamic> data = [
{"Name": "John", "Age": 28, "Role": "Senior Supervisor"},
{"Name": "Jane", "Age": 32, "Role": "Regional Administrator"},
{"Name": "Mary", "Age": 28, "Role": "Manager"},
{"Name": "Kumar", "Age": 32, "Role": "Administrator"},
];
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
useMaterial3: true,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter DataTable Example'),
),
body: Center(
child: DataTable(
border: TableBorder.all(
width: 1,
borderRadius: const BorderRadius.only(
topLeft: Radius.circular(10),
topRight: Radius.circular(20),
bottomLeft: Radius.circular(30),
bottomRight: Radius.circular(40))),
columns: const <DataColumn>[
DataColumn(
label: Text('Name'),
),
DataColumn(
label: Text('Age'),
numeric: true,
),
DataColumn(
label: Text('Role'),
),
],
rows: List.generate(data.length, (index) {
final item = data[index];
return DataRow(
cells: [
DataCell(Text(item['Name'])),
DataCell(Text(item['Age'].toString())),
DataCell(Text(item['Role'])),
],
);
}),
)),
);
}
}
That’s how you change the Flutter DataTable border radius easily.