How to set Checkbox Border Radius in Flutter
The proper usage of border radius helps you to give some pretty neat UI components. In this blog post, let’s learn how to set the CheckBox border radius in Flutter.
The CheckBox widget has so many properties for customization. You can change the border color, border width, border radius, etc. You can customize border radius using the shape property.
The shape property accepts RoundedRectangleBorder class. See the following code snippet.
Checkbox(
shape:
RoundedRectangleBorder(borderRadius: BorderRadius.circular(5)),
value: checkBoxValue,
onChanged: (bool? newValue) {
setState(() {
checkBoxValue = newValue!;
});
})
Then you will get the following output.
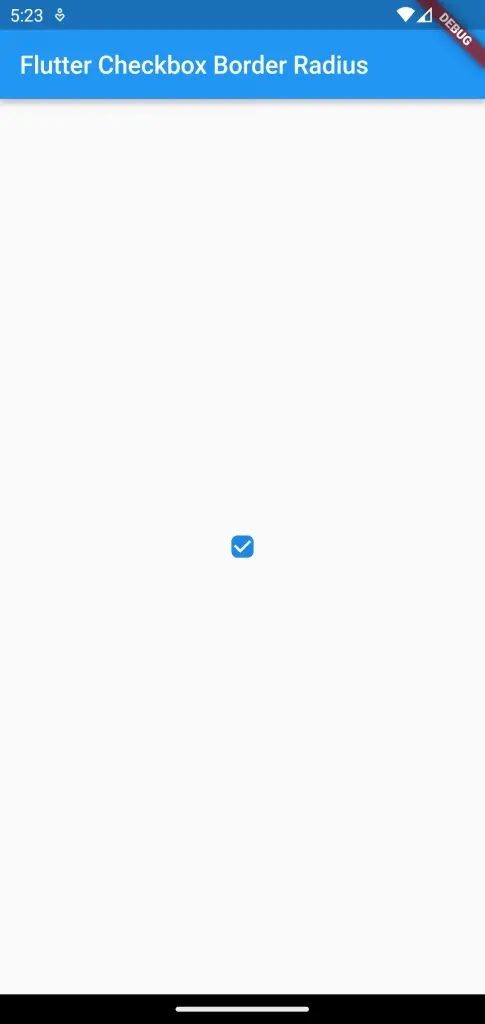
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Checkbox Border Radius'),
),
body: const MyStatefulWidget());
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
bool checkBoxValue = false;
@override
Widget build(BuildContext context) {
return Center(
child: Checkbox(
shape:
RoundedRectangleBorder(borderRadius: BorderRadius.circular(5)),
value: checkBoxValue,
onChanged: (bool? newValue) {
setState(() {
checkBoxValue = newValue!;
});
}));
}
}
That’s how you change the default border radius of CheckBox in Flutter.
If you want to make the CheckBox round then see this Flutter tutorial to create a circular CheckBox.