How to change Floating Action Button Position in Flutter
The Floating Action Button or FAB is an important button widget in Flutter. In this blog post, let’s learn how to change the position of the Floating Action Button in Flutter.
By default, the position of the FloatingActionButton is bottom right. You can change the default FAB position using the floatingActionButtonLocation property of Scaffold.
See the code snippet given below.
floatingActionButtonLocation: FloatingActionButtonLocation.centerFloat,
floatingActionButton: FloatingActionButton(
onPressed: () {},
child: const Icon(Icons.add),
),
Here, the position of FAB will be the bottom center.
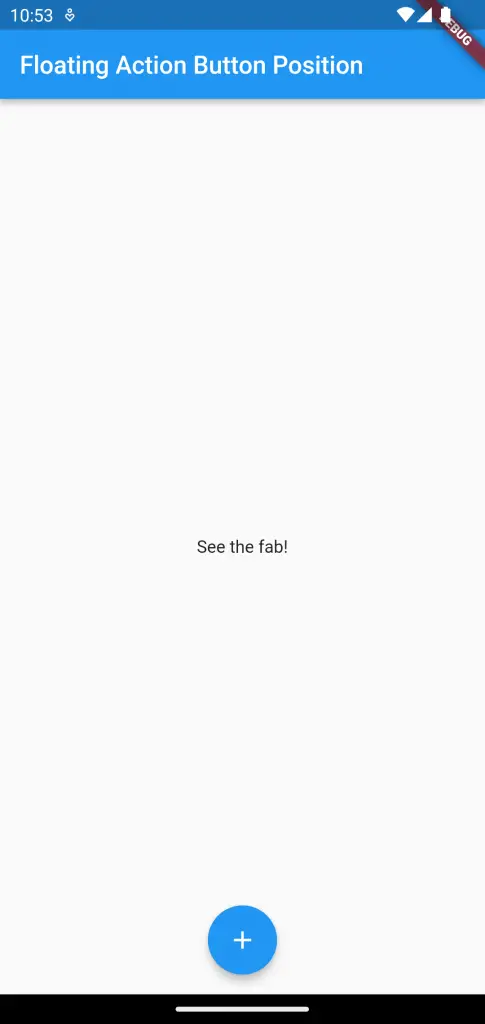
If you want to change the position of FAB to the left bottom then you should choose FloatingActionButtonLocation.startFloat.
floatingActionButtonLocation: FloatingActionButtonLocation.startFloat,
floatingActionButton: FloatingActionButton(
onPressed: () {},
child: const Icon(Icons.add),
),
See the following output.
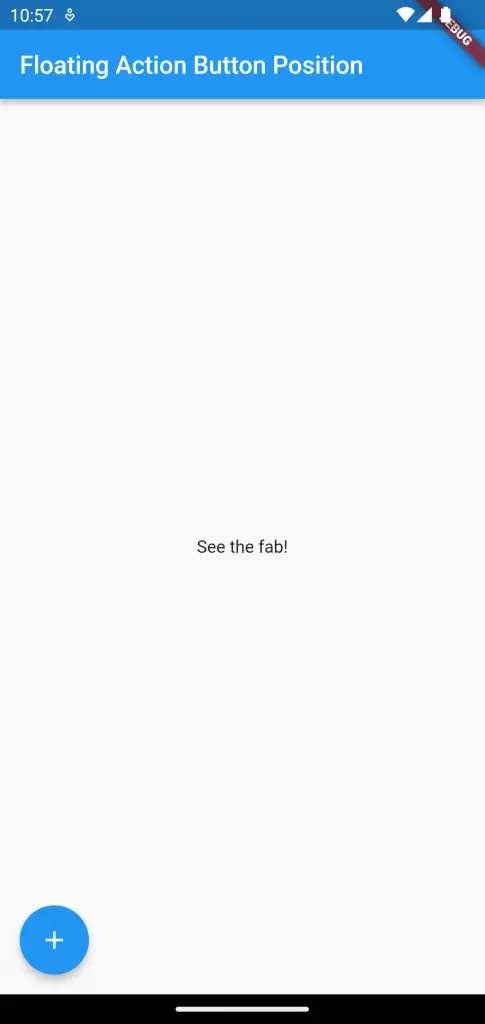
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Floating Action Button Position'),
),
body: const Center(child: Text('See the fab!')),
floatingActionButtonLocation: FloatingActionButtonLocation.startFloat,
floatingActionButton: FloatingActionButton(
onPressed: () {},
child: const Icon(Icons.add),
),
);
}
}
You can try different locations by using FloatingActionButtonLocation to get the desired result.
That’s how you change Floating Action Button position in Flutter.