How to change Shadow Color of Elevated Button in Flutter
Nowadays, many Flutter developers prefer ElevatedButton as their button. In this Flutter tutorial, let’s check how to change the ElevatedButton shadow color.
The styling of the ElevatedButton is done with the help of the ButtonStyle class. The ElevatedButton has shadows by default because of elevation. You can customize the shadow color using the shadowColor property of the ButtonStyle class.
ElevatedButton(
style: ElevatedButton.styleFrom(
elevation: 20, shadowColor: Colors.green),
onPressed: () {},
child: const Text('Elevated Button Shadow')),
As you see, the elevation and shadow color are controlled using ElevatedButton.styleFrom method. We changed the default elevation color to green color. The output is given below.
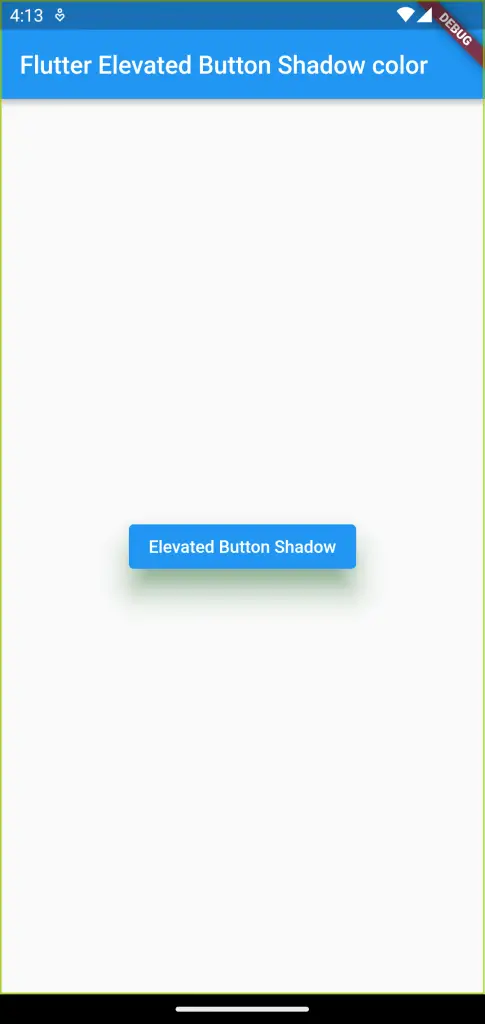
Following is the complete example of ElevatedButton with custom shadow color in Flutter.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
'Flutter Elevated Button Shadow color',
),
),
body: Center(
child: ElevatedButton(
style: ElevatedButton.styleFrom(
elevation: 20, shadowColor: Colors.green),
onPressed: () {},
child: const Text('Elevated Button Shadow')),
));
}
}
Like this, you can do a lot of customizations to the ElevatedButton. For example, you can customize the borders and the border colors.
That’s it. I hope this Flutter tutorial of ElevatedButton with custom elevation color is helpful for you.