How to set Background Image for Flutter Row
Incorporating images into your Flutter application can greatly enhance the visual experience. Sometimes, you might want to display a row of widgets over a background image. This is particularly useful for creating attractive headers, banners, or other design elements.
In this blog post, we’ll explore how to add a background image to a Row
widget in Flutter.
The Row
widget doesn’t directly support background images. To add an image behind a Row
, you must use a Container
or a DecoratedBox
. These widgets come with the decoration
property, allowing you to add various decorations, including an image.
Set Up Your Image Asset
Before coding, ensure your image asset is added to the project. Update your pubspec.yaml
file to include the asset:
flutter:
assets:
- assets/background.jpg
Set Background Image
To set a background image for a Row
, you’ll start with a Container
. Containers are incredibly versatile and allow for decoration customization, which is necessary for adding a background image.
Here’s the example code:
Container(
width: double.infinity, // Full width of the screen
height: 300.0, // Set a fixed height for the container
decoration: const BoxDecoration(
image: DecorationImage(
image: AssetImage("assets/background.jpg"),
fit: BoxFit.cover, // Ensures the image covers the container
),
),
child: const Row(
children: [
Text(
'A Row with a Background Image',
),
],
),
)
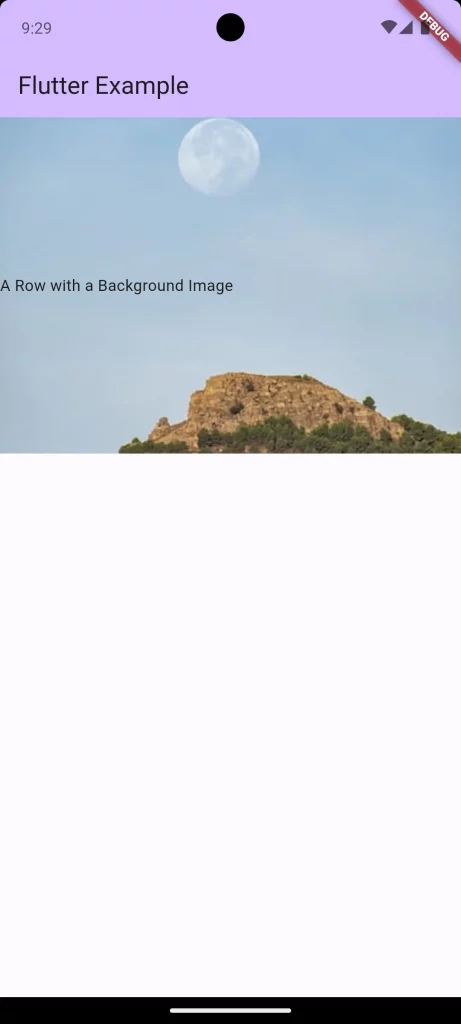
Let’s break down the key parts of this implementation:
Full-Screen Width
width: double.infinity
sets the container’s width to match the full width of the screen, ensuring the background image spans across the entire width.
Fixed Height
height: 300.0
gives the container a fixed height of 300 pixels. This is important because it determines the vertical space your background image will occupy.
BoxDecoration
The decoration
property of the container is set to a BoxDecoration
. The BoxDecoration
allows us to add the background image via the image
property, which takes a DecorationImage
.
DecorationImage
DecorationImage
has an image
property where you specify the image provider, in this case, AssetImage("assets/background.jpg")
. This tells Flutter to look for the specified image in the project’s asset folder.
BoxFit.cover
fit: BoxFit.cover
is important for a full and responsive cover of the given space by the background image. It tells Flutter how to handle the image’s sizing when laying it out in the container, ensuring the image fully covers the container without distorting its aspect ratio.
Inside the Container
, a Row
widget is placed as a child. This Row
can have any number of children inside it, and they will all be laid out on top of the background image.
Following is the complete code for reference.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple),
useMaterial3: true,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Theme.of(context).colorScheme.inversePrimary,
title: const Text(
'Flutter Example',
),
),
body: Container(
width: double.infinity, // Full width of the screen
height: 300.0, // Set a fixed height for the container
decoration: const BoxDecoration(
image: DecorationImage(
image: AssetImage("assets/background.jpg"),
fit: BoxFit.cover, // Ensures the image covers the container
),
),
child: const Row(
children: [
Text(
'A Row with a Background Image',
),
],
),
));
}
}
Adding a background image to a Row
in Flutter is an effective way to create visually compelling UI components. With the combination of a Container
and its decoration properties, you can ensure that your image spans the desired area and that any child widgets are well-positioned over it.