How to Create a Full Width Row in Flutter
When designing the layout for your Flutter app, you may find the need for a Row
that stretches across the entire width of the screen. This is often required when you’re aiming to create header sections, footers, or any horizontal elements that need to span the full screen.
In Flutter, making a Row
widget expand to the full width is quite straightforward. This post will guide you through the process of creating a full-width Row
in Flutter.
Row Widget
A Row
is a widget that displays its children in a horizontal array. By default, it sizes itself to contain its children, meaning it will be as wide as the sum of the widths of its children. To make it stretch across the entire width of the screen, we need to take a different approach.
Use the MainAxisSize Property
The Row
widget has a property called mainAxisSize
that determines how much space it should occupy on the main axis (which is horizontal for a Row
). By setting this property to MainAxisSize.max
, you can make the Row
as wide as possible:
Row(
mainAxisSize: MainAxisSize.max,
children: [
// Your widgets here
],
)
However, just setting mainAxisSize
to MainAxisSize.max
doesn’t always guarantee that the Row
will fill the width. This is where other widgets and properties come into play.
Use Expanded Widget
When you have multiple children in a Row
and you want them to fill the space, wrapping a child with an Expanded
widget tells Flutter to let the child expand to fill the available space:
Row(
children: [
Expanded(
child: Container(
color: Colors.blue,
child: const Text('Full-width element within a Row'),
),
),
],
)
In this example, the Container
takes up all the available horizontal space within the Row
.
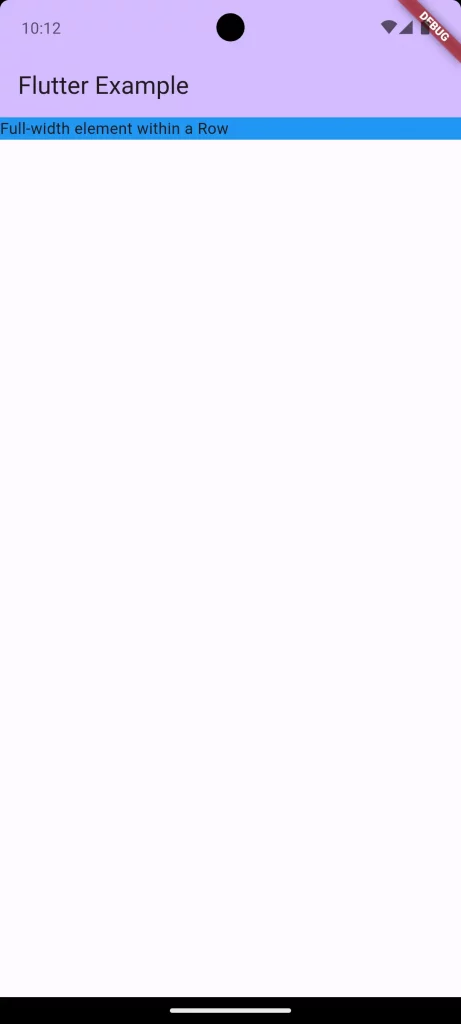
CrossAxisAlignment
To ensure your Row
elements are stretched fully in terms of width and appropriately aligned vertically, you can combine CrossAxisAlignment
with Expanded
:
Row(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: [
Expanded(
child: Container(
color: Colors.red,
child: const Text('Stretched full-width Row with CrossAxisAlignment'),
),
),
],
)
The crossAxisAlignment: CrossAxisAlignment.stretch
ensures that any child widgets that do not have a fixed height will be stretched to fill the Row
vertically.
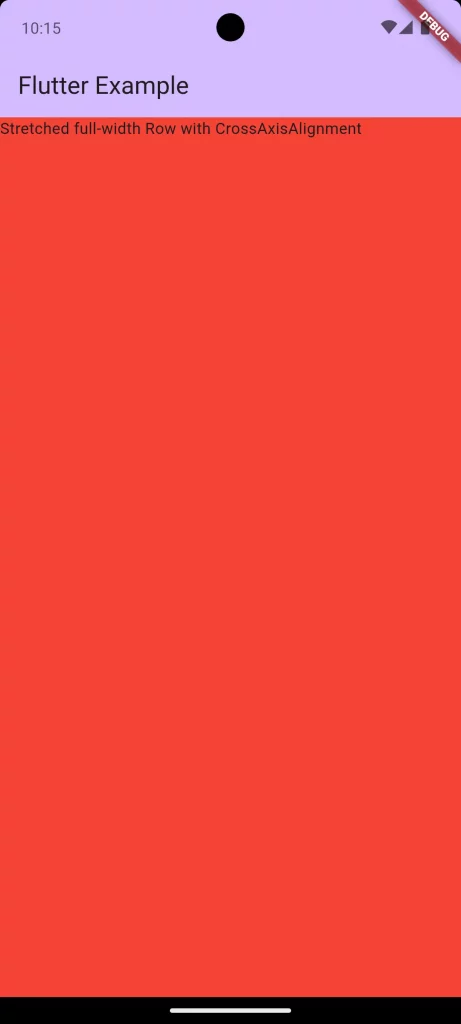
Full-Width Row with double.infinity
Here’s how you can make a Row
full-width by enclosing it within a Container
and setting its width to double.infinity
:
Container(
width: double.infinity,
color: Colors.blueGrey, // Just for visibility
child: const Row(
children: [
Text('Full-width Row using double.infinity'),
// More widgets can be added here
],
),
)
In this example, the Container
encapsulating the Row
will expand to the full width of the screen, thus making the Row
appear full-width.
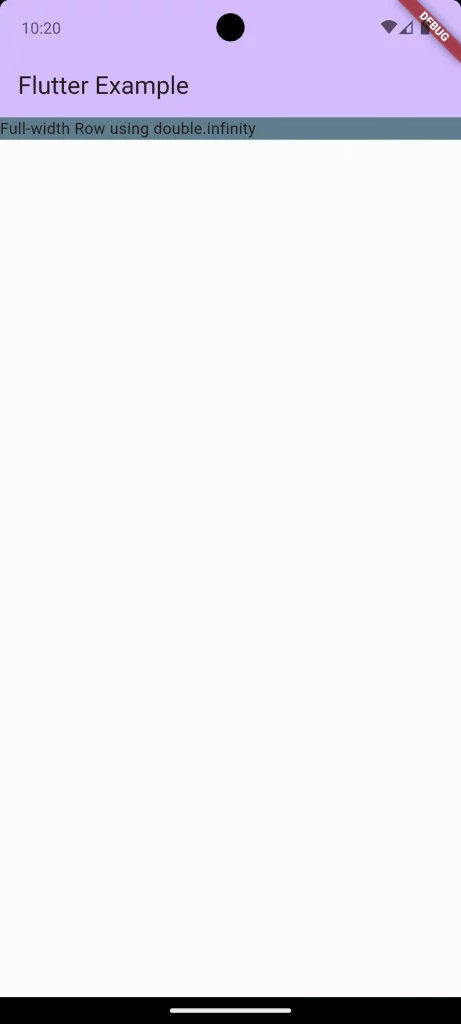
Creating a full-width Row
in Flutter is essential for developing responsive and visually appealing layouts. By manipulating the mainAxisSize
property and using widgets like Expanded
, you can control how your Row
behaves in terms of width.
One Comment