How to show Text in Lowercase in Flutter
Sometimes, you may want to show text always in a specific case- lowercase or uppercase. In this short Flutter tutorial, let’s learn how to display Text always in lowercase.
The Text widget doesn’t have any property related to capitalization. Hence, we should use Dart method toLowerCase() to obtain the desired result.
See the code snippet given below.
var text = 'FLUTTER TEXT LOWERCASE'.toLowerCase();
Text(text,
style: const TextStyle(
fontSize: 18,
color: Colors.red,
)),
Firstly, declare a string variable and convert it to lowercase using toLowerCase method. Then include the variable as data in the Text widget.
Following is the output.
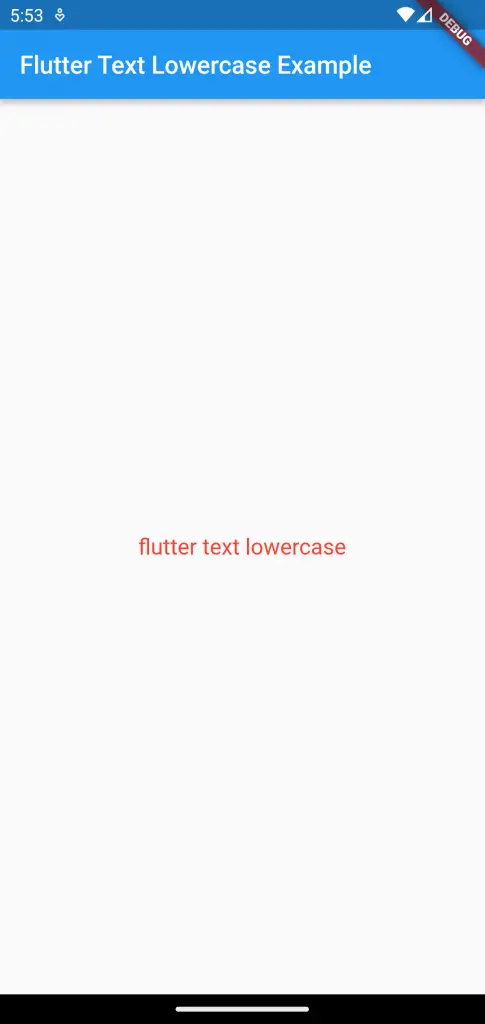
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
var text = 'FLUTTER TEXT LOWERCASE'.toLowerCase();
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Text Lowercase Example'),
),
body: Center(
child: Text(text,
style: const TextStyle(
fontSize: 18,
color: Colors.red,
)),
),
);
}
}
That’s how you show Text in small case in Flutter.