How to show Image from URL in Flutter
Images are one of the most used UI components of a mobile app. In this blog post, let’s learn how to display an image that loads from the internet in Flutter.
Flutter has Image widget to display images. The Image widget has multiple constructors such as Image.new, Image.network, Image.file, etc. We use Image.network constructor to display an image from a URL.
The code snippet is given below.
Image.network(
'https://images.pexels.com/photos/6955476/pexels-photo-6955476.jpeg?auto=compress&cs=tinysrgb&w=1260&h=750&dpr=2',
width: 300,
height: 500)
You can specify width, height, cacheWidth, and cacheHeight to adjust the size of the image loading from the URL.
Following is the complete code for reference.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Image from URL'),
),
body: Center(
child: Image.network(
'https://images.pexels.com/photos/6955476/pexels-photo-6955476.jpeg?auto=compress&cs=tinysrgb&w=1260&h=750&dpr=2',
width: 300,
height: 500),
));
}
}
Here I used a free image from Unsplash. Following is the output.
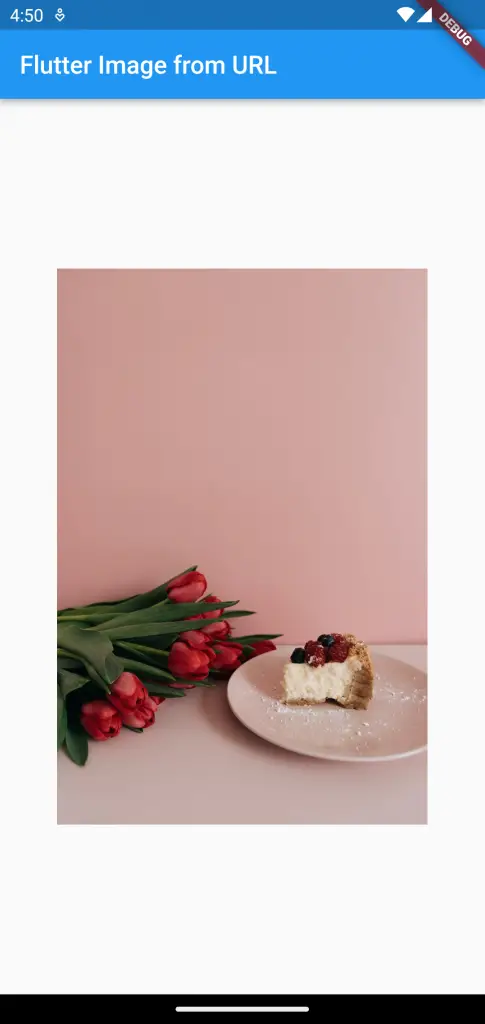
Here, there are no effects on the image while loading from the internet. You can even add a fade-in effect and a placeholder to make the loading of the image more attractive.
In the case of loading local images, you can use Image.asset constructor to load images from assets.
I hope this Flutter image from a URL tutorial is useful for you.