How to Show Border Around Image in Flutter
I have already posted on how to add an image from assets in flutter and how to show image from url in flutter. In this blog post, Let’s learn how to add a border around an image in Flutter.
In order to show local images on your flutter app, you need to add images inside your project folder. You can follow the steps to add images from this link. After adding an image what you need is to draw a border around the image.
We use BoxDecoration class to show the border. Box decoration has body and border properties. Here I am using a red color border with a width of 10 around the image.
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Welcome to Flutter',
home: Scaffold(
appBar: AppBar(
title: Text('Flutter Image with border Example'),
),
body: Center(
child: Body()
),
),
);
}
}
/// This is the stateless widget that the main application instantiates.
class Body extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Container(
decoration: BoxDecoration(
color: const Color(0xff7c94b6),
image: DecorationImage(
image: ExactAssetImage('images/girl.png'),
fit: BoxFit.cover,
),
border: Border.all(
color: Colors.red,
width: 10.0,
),
),
);
}
}
The output of the flutter example is given below.
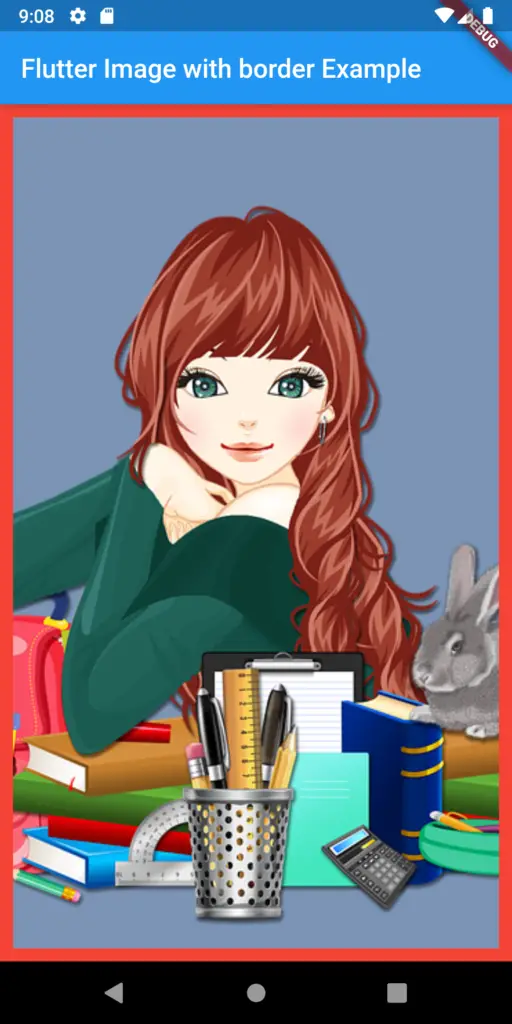
That’s how you add a border around the image in Flutter.