Change Elevated Button Color on Press in Flutter
The ElevatedButton is the ready-to-go button for most of the Flutter developers there. In this blog post, let’s learn how to change the color of the elevated button at the time of pressing.
The ElevatedButton is styled using the ButtonStyle class. You can change the background color of an ElevatedButton using MaterialStateProperty class. You can alter the color of the button based on the states too. See the code snippet given below.
ElevatedButton(
style: ButtonStyle(
backgroundColor: MaterialStateProperty.resolveWith<Color>(
(Set<MaterialState> states) {
if (states.contains(MaterialState.pressed)) {
return Colors.green;
}
return Colors.greenAccent;
},
),
),
onPressed: () {
print('Pressed');
},
child: const Text('Elevated Button'),
),
Here, we set the green color when the button state is pressed whereas the green accent color is set for all other button states.
Following is the complete code for this Flutter tutorial.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Elevated Button OnPress Color Change'),
),
body: Center(
child: ElevatedButton(
style: ButtonStyle(
backgroundColor: MaterialStateProperty.resolveWith<Color>(
(Set<MaterialState> states) {
if (states.contains(MaterialState.pressed)) {
return Colors.green;
}
return Colors.greenAccent;
},
),
),
onPressed: () {},
child: const Text('Elevated Button'),
),
));
}
}
The button with green accent color changed into a green color button when it is pressed. See the output given below.
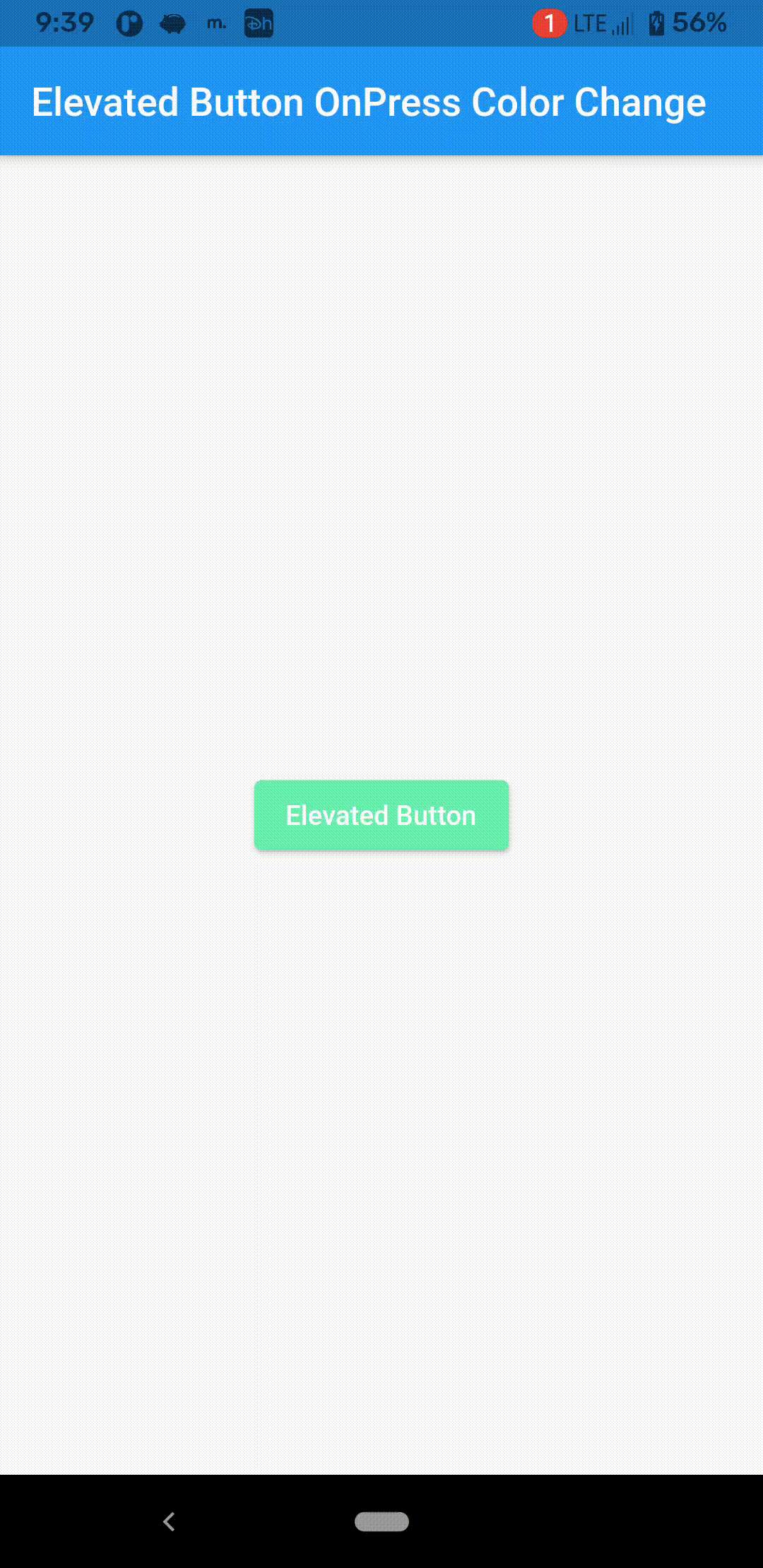
That’s how you change the background color for ElevatedButton when pressed in Flutter.
If you want to change the color of the ElevatedButton irrespective of button states then check out the ElevatedButton Color tutorial.