How to Disable Zoom in Flutter WebView
WebView is used in mobile apps to display web content such as web apps, web pages, etc. Sometimes, as a developer, you may want to disable the WebView zoom feature. In this blog post, let’s check how to disable zoom in Flutter WebView.
In this tutorial, we use the webview_flutter package to add WebView in the Flutter app. You can install the package using the following command.
flutter pub add webview_flutter
After that, you can import the package as given below.
import 'package:webview_flutter/webview_flutter.dart';
By default, the WebView can be zoomed in and zoomed out by the user. You can disable zoom in WebView by using the enableZoom method of the WebViewController. You just need to make its value false.
controller.enableZoom(false);
Following is the example of a WebView with a progress indicator in Flutter. We just disable the zoom by setting enableZoom false.
import 'package:flutter/material.dart';
import 'package:webview_flutter/webview_flutter.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
useMaterial3: true,
),
home: const MyStatefulWidget());
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
late final WebViewController controller;
var loadingPercentage = 0;
@override
void initState() {
super.initState();
controller = WebViewController()
..setNavigationDelegate(NavigationDelegate(
onPageStarted: (url) {
setState(() {
loadingPercentage = 0;
});
},
onProgress: (progress) {
setState(() {
loadingPercentage = progress;
});
},
onPageFinished: (url) {
setState(() {
loadingPercentage = 100;
});
},
))
..loadRequest(
Uri.parse('https://flutter.dev'),
);
controller.enableZoom(false);
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter WebView example'),
),
body: Stack(
children: [
WebViewWidget(
controller: controller,
),
if (loadingPercentage < 100)
LinearProgressIndicator(
value: loadingPercentage / 100.0,
),
],
));
}
}
The MyStatefulWidget widget is a stateful widget that creates a WebViewController instance to control the WebView, and sets up a NavigationDelegate to handle navigation events in the WebView.
In the initState() method of the MyStatefulWidget widget, the WebViewController instance is initialized with the NavigationDelegate, and loads the URL of a website to display in the WebView. Additionally, the zoom functionality is disabled.
The build() method of the MyStatefulWidget widget returns a Scaffold widget that displays an AppBar with a title and a WebViewWidget that displays the web content.
A LinearProgressIndicator is also displayed if the web content is still loading, which is controlled by the loadingPercentage variable that is updated by the NavigationDelegate.
You will get the following output of webview that can’t be zoomed.
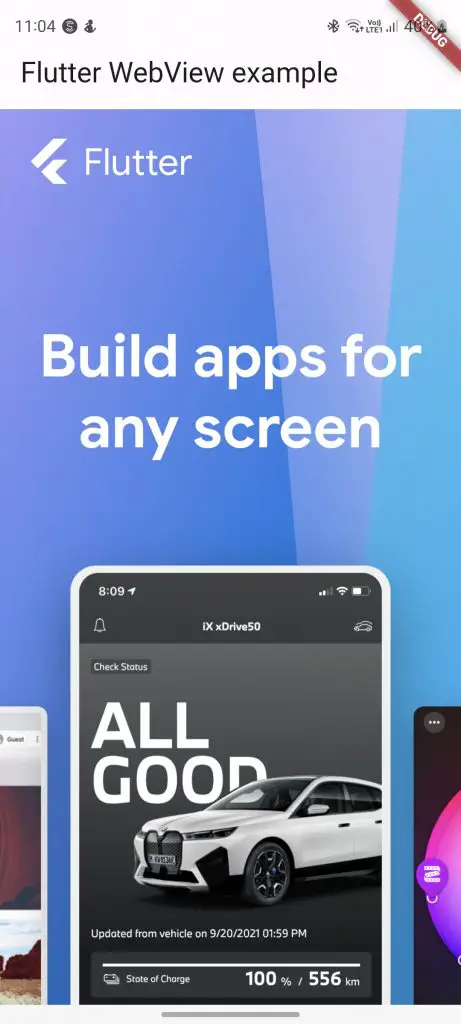
That’s how you disable zoom in Flutter WebView. I hope this Flutter tutorial is helpful for you.