How to Create a WebView with Progress Indicator in Flutter
When you are using web view to show some web pages inside your mobile app you may always want to show a progress indicator at the time of loading. In this blog post let’s see how to add a webview with a progress indicator in Flutter.
If you don’t know how to add a webview in Flutter, then please check out Flutter WebView tutorial.
First, you need to install webview flutter using the command given below.
flutter pub add webview_flutter
Make sure that minSdkVersion in android/app/build.gradle is 19 or greater than 19.
android {
defaultConfig {
minSdkVersion 19
...
}
}
Also, add internet permission in the Android Manifest file.
<uses-permission android:name="android.permission.INTERNET" />
You can import the package as given below.
import 'package:webview_flutter/webview_flutter.dart';
The WebView widget offers several page load progress events that your app can monitor, including onPageStarted, onProgress, and onPageFinished, which are fired at various stages during the WebView page load cycle.
This flutter webview with a progress indicator example uses widgets such as Stack and LinearProgressIndicator.
Following is the complete code.
import 'package:flutter/material.dart';
import 'package:webview_flutter/webview_flutter.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
useMaterial3: true,
),
home: const MyStatefulWidget());
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
late final WebViewController controller;
var loadingPercentage = 0;
@override
void initState() {
super.initState();
controller = WebViewController()
..setNavigationDelegate(NavigationDelegate(
onPageStarted: (url) {
setState(() {
loadingPercentage = 0;
});
},
onProgress: (progress) {
setState(() {
loadingPercentage = progress;
});
},
onPageFinished: (url) {
setState(() {
loadingPercentage = 100;
});
},
))
..loadRequest(
Uri.parse('https://flutter.dev'),
);
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter WebView Example'),
),
body: Stack(
children: [
WebViewWidget(
controller: controller,
),
if (loadingPercentage < 100)
LinearProgressIndicator(
value: loadingPercentage / 100.0,
),
],
));
}
}
Following is the output of this flutter tutorial.
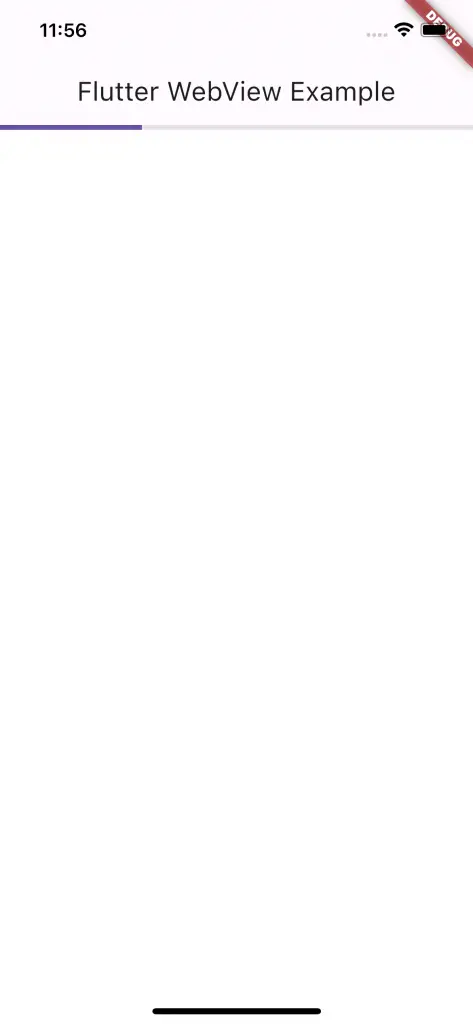
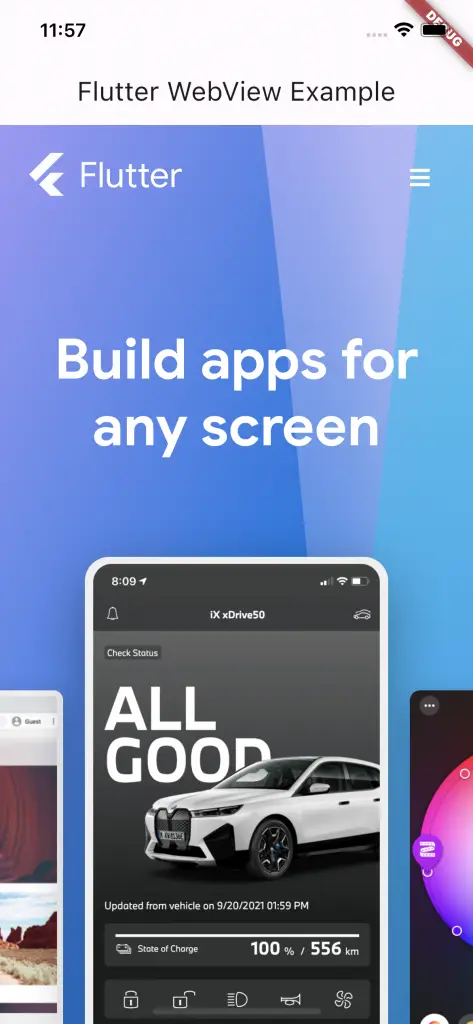
I hope this flutter tutorial of webview with loading will help you. Stay tuned for more tutorials.
This gives a nice look, thank you!