How to set Full Screen Image Background in Flutter
As a mobile app developer, everyone wants to make each screen of their apps beautiful and unique. In some scenarios, you may prefer to set an image background to screens such as splash screens. In this Flutter tutorial, let’s check how to set the image background in Flutter.
Here, I am explaining two ways to set an image background. The first way is by using the Stack widget. The Stack widget helps us to create multiple layers of widgets that overlay each other in a given order.
Stack(
children: <Widget>[
Bottom(),
Middle(),
Top(),
],
),
Here, the Bottom widget will be the bottom most widget. The Middle widget overlays over Bottom widget. The Top widget appears over both the Bottom and Middle widgets.
The trick is to use the Image as the bottom widget of the Stack. Place other widgets on the screen over the image widget. Go through the example given below for a full idea.
import 'package:flutter/material.dart';
void main() => runApp(App());
class App extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Image Background Example',
home: FlutterExample(),
);
}
}
class FlutterExample extends StatelessWidget {
FlutterExample({Key key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
body: Stack(
children: <Widget>[
Image.network(
'https://cdn.pixabay.com/photo/2015/03/26/09/41/phone-690091_960_720.jpg',
width: MediaQuery.of(context).size.width,
height: MediaQuery.of(context).size.height,
fit: BoxFit.cover,
),
Center(
child: Text("This is a Flutter Example",
style: TextStyle(
fontWeight: FontWeight.bold,
fontSize: 28.0,
color: Colors.red)),
),
],
));
}
}
The Text appears over the background image. See the following output.
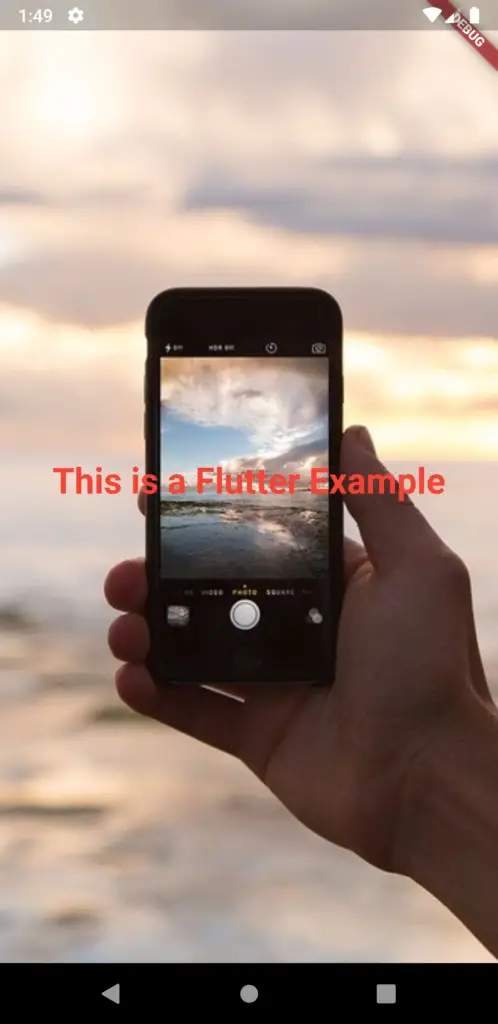
Another way to create an image background is by using the Container and the BoxDecoration widgets. The following is an example of adding an image background in Flutter using the BoxDecoration widget.
import 'package:flutter/material.dart';
void main() => runApp(App());
class App extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Image Background Example',
home: FlutterExample(),
);
}
}
class FlutterExample extends StatelessWidget {
FlutterExample({Key key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
body: Container(
constraints: BoxConstraints.expand(),
decoration: BoxDecoration(
image: DecorationImage(
image: NetworkImage(
'https://cdn.pixabay.com/photo/2015/03/26/09/41/phone-690091_960_720.jpg',
),
fit: BoxFit.cover,),),
child: Center(
child:Text("Flutter Example",
style: TextStyle(
fontWeight: FontWeight.bold,
fontSize: 28.0,
color: Colors.red)),)
));
}
}
Following is the output of this Flutter example.
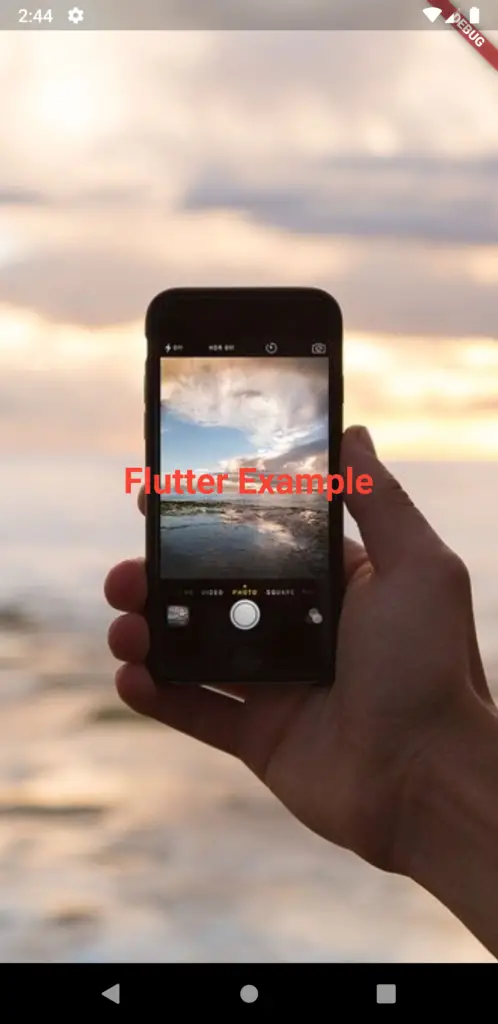
I hope this Flutter Image background tutorial will help you. Stay tuned for more Flutter tutorials.