How to Add Margins to a Widget in Flutter
Margins are required to align and place components of your user interface properly and neatly. In this blog post let’s check how to add margins to a widget in Flutter.
I had already posted on how to add padding to a widget in flutter. In order to set margins to a widget, you need to wrap that widget with a Container widget. The Container has a property named margin.
Hence, you can add margins to a widget as given below:
Container(
margin: EdgeInsets.only(left:30.0, top:20.0,right:30.0,bottom:20.0),
child: Text("Check out my margins!"),
)
If you want the same margin for all edges then you can use the following snippet.
Container(
margin: EdgeInsets.all(30.0),
child: Text("Check out my margins!"),
)
Following is the complete flutter example to use margins.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Margins example'),
),
body: Container(
margin: const EdgeInsets.all(30),
child: const Text("Check out my margins!"),
),
);
}
}
Following is the output screenshot of the flutter example.
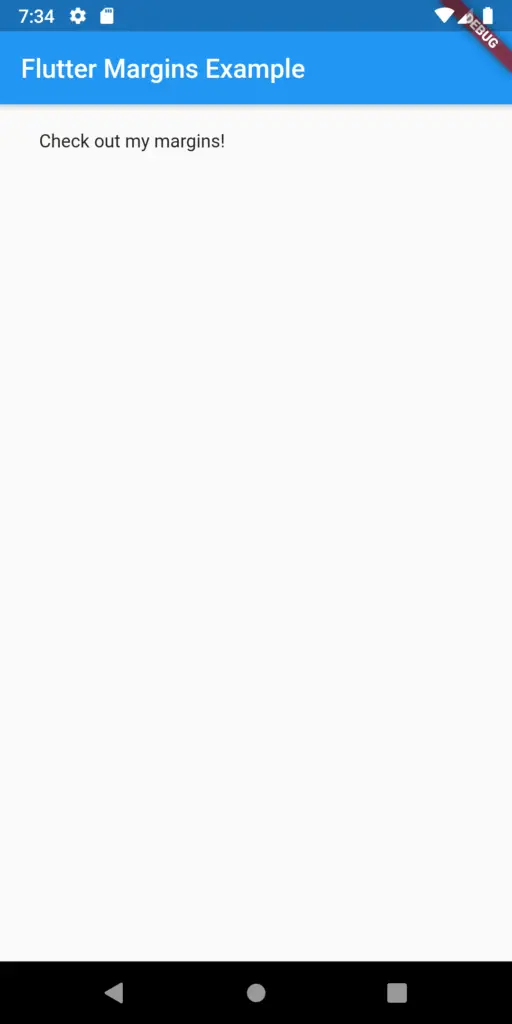
That’s how you add margin to widgets in Flutter.