How to add ElevatedButton with Transparent Background
You can do so many visual customizations to make ElevatedButton more beautiful. Sometimes, you may want to set the ElevatedButton background transparent. Let’s look at that case in this Flutter tutorial.
The ElevatedButton.styleFrom method is used to customize the style of ElevatedButton. Its property backgroundColor helps to set a background color. We make Colors.transparent as the background color.
See the code snippet given below for more understanding.
ElevatedButton(
onPressed: () {},
style: ElevatedButton.styleFrom(
backgroundColor: Colors.transparent,
foregroundColor: Colors.black,
elevation: 0,
side: const BorderSide(
width: 1.0,
color: Colors.red,
)),
child: const Text(' Elevated Button'))
Here, the foregroundColor determines the text color. You also need to set the elevation as 0. Otherwise a gray color background will be shown because of elevation. We also added a border to the ElevatedButton.
Following is the output.
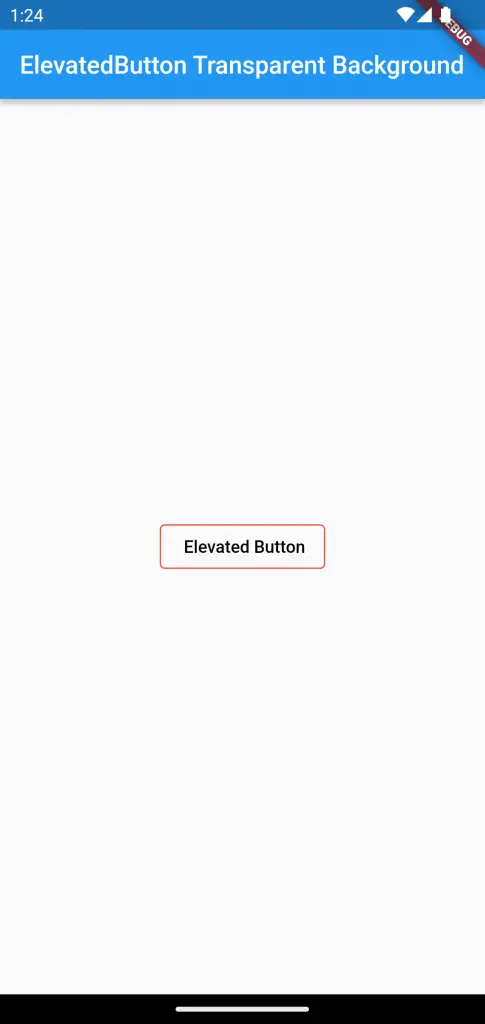
As you see, this makes the button completely transparent. If you want to show a partially transparent ElevatedButton then see this ElevatedButton opacity tutorial.
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('ElevatedButton Transparent Background'),
),
body: Center(
child: ElevatedButton(
onPressed: () {},
style: ElevatedButton.styleFrom(
backgroundColor: Colors.transparent,
foregroundColor: Colors.black,
elevation: 0,
side: const BorderSide(
width: 1.0,
color: Colors.red,
)),
child: const Text('Elevated Button'))));
}
}
I hope this Flutter ElevatedButton transparent background tutorial is helpful for you.