How to Load PDF from Assets in Flutter
PDF (Portable Document Format) is a widely-used file format for documents that need to maintain their formatting across multiple platforms and devices. In this blog post, we will explore how to load PDF files from the assets directory in a Flutter application.
Here, We are using the pdfx package to display PDF files. You can install pdfx using the following command.
flutter pub add pdfx
Now add a sample pdf to your assets directory by following the steps given below.
- Create an “assets” directory in the root of your Flutter project, if it does not already exist
- Add your PDF file to the “assets” directory.
- Update the pubspec.yaml file to include the PDF file as assets. To do this, add the following code under the “flutter:” section of the file.
assets:
- assets/sample.pdf
...
You can import the pdfx package as given below.
import 'package:pdfx/pdfx.dart';
Then pdfController is initialized as given below.
final pdfController = PdfController(
document: PdfDocument.openAsset('assets/sample.pdf'),
);
After that, you can use PdfView widget to display the pdf file.
PdfView(
controller: pdfController,
),
Following is the basic Flutter code to open a PDF file from the assets.
import 'package:flutter/material.dart';
import 'package:pdfx/pdfx.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
home: Scaffold(
appBar: AppBar(title: const Text('Flutter PDF Example')),
body: const MyStatefulWidget(),
),
);
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
final pdfController = PdfController(
document: PdfDocument.openAsset('assets/sample.pdf'),
);
@override
Widget build(BuildContext context) {
return PdfView(
controller: pdfController,
);
}
}
You will get the following output.
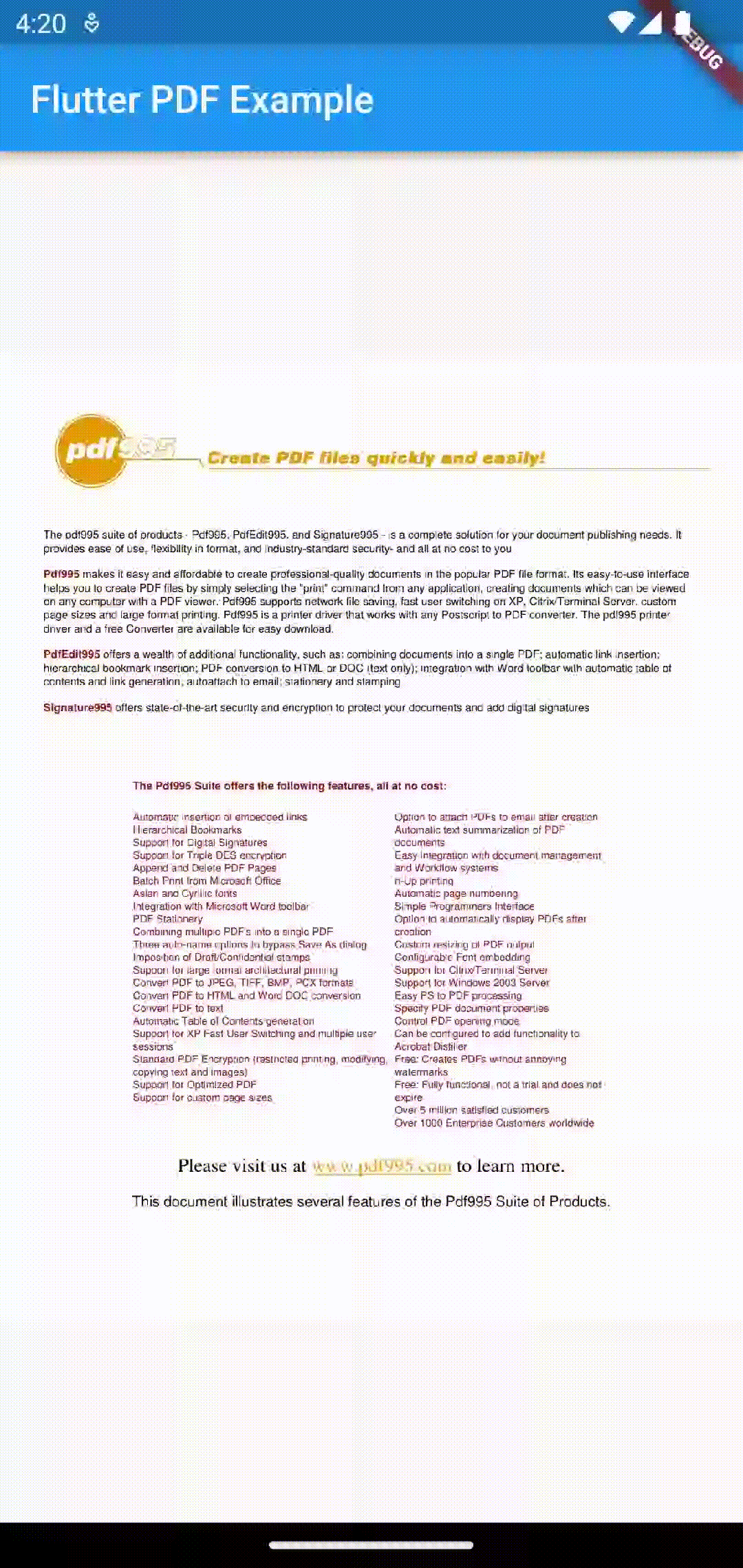
Most of the time, you may want a full-fledged pdf viewer with details such as page numbers, navigation options, etc. The pdfx package has many useful things to implement additional features.
See the following code.
import 'package:flutter/material.dart';
import 'package:pdfx/pdfx.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
useMaterial3: true,
),
home: const MyStatefulWidget());
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
static const int _initialPage = 1;
late PdfController _pdfController;
@override
void initState() {
super.initState();
_pdfController = PdfController(
document: PdfDocument.openAsset('assets/sample.pdf'),
initialPage: _initialPage,
);
}
@override
void dispose() {
_pdfController.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter PDF Example'),
actions: <Widget>[
IconButton(
icon: const Icon(Icons.navigate_before),
onPressed: () {
_pdfController.previousPage(
curve: Curves.ease,
duration: const Duration(milliseconds: 100),
);
},
),
PdfPageNumber(
controller: _pdfController,
builder: (_, loadingState, page, pagesCount) => Container(
alignment: Alignment.center,
child: Text(
'$page/${pagesCount ?? 0}',
style: const TextStyle(fontSize: 22),
),
),
),
IconButton(
icon: const Icon(Icons.navigate_next),
onPressed: () {
_pdfController.nextPage(
curve: Curves.ease,
duration: const Duration(milliseconds: 100),
);
},
),
],
),
body: PdfView(
builders: PdfViewBuilders<DefaultBuilderOptions>(
options: const DefaultBuilderOptions(),
documentLoaderBuilder: (_) =>
const Center(child: CircularProgressIndicator()),
pageLoaderBuilder: (_) =>
const Center(child: CircularProgressIndicator()),
),
controller: _pdfController,
),
);
}
}
And you will get the following output.
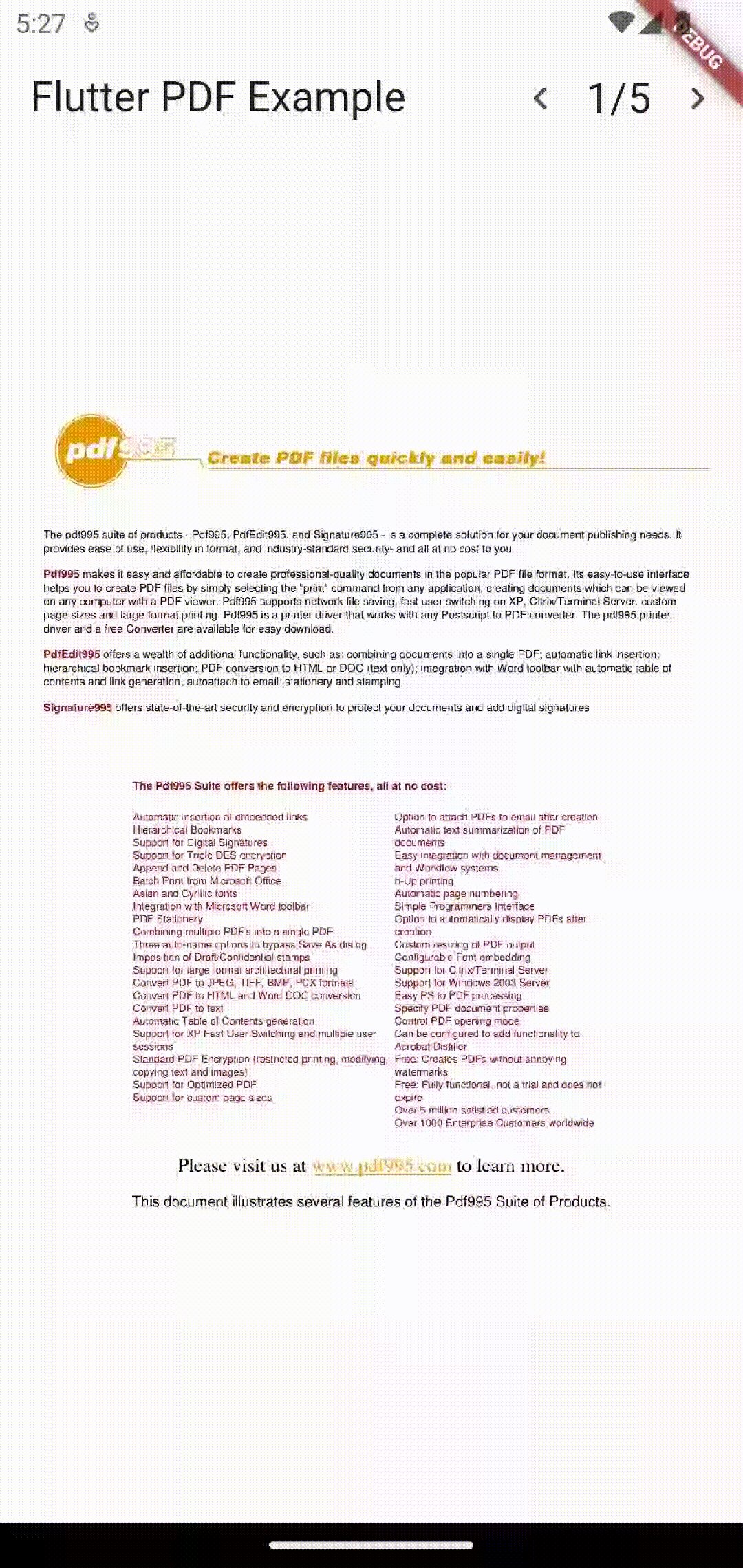
That’s how you show PDF files from assets in Flutter.