How to set Gradient Background for AppBar in Flutter
Gradients created with multiple colors always make UI components more attractive. Let’s learn how to add AppBar with a gradient background in Flutter.
The flexibleSpace property helps to add widgets to customize AppBar. Using BoxDecoration and LinearGradient you can create a gradient background.
See the code snippet given below.
AppBar(
title: const Text(
'Flutter AppBar Gradient Background',
),
flexibleSpace: Container(
decoration: const BoxDecoration(
gradient: LinearGradient(
begin: Alignment.centerLeft,
end: Alignment.centerRight,
colors: <Color>[Colors.cyan, Colors.green])),
)),
Following is the output of AppBar with gradient background.
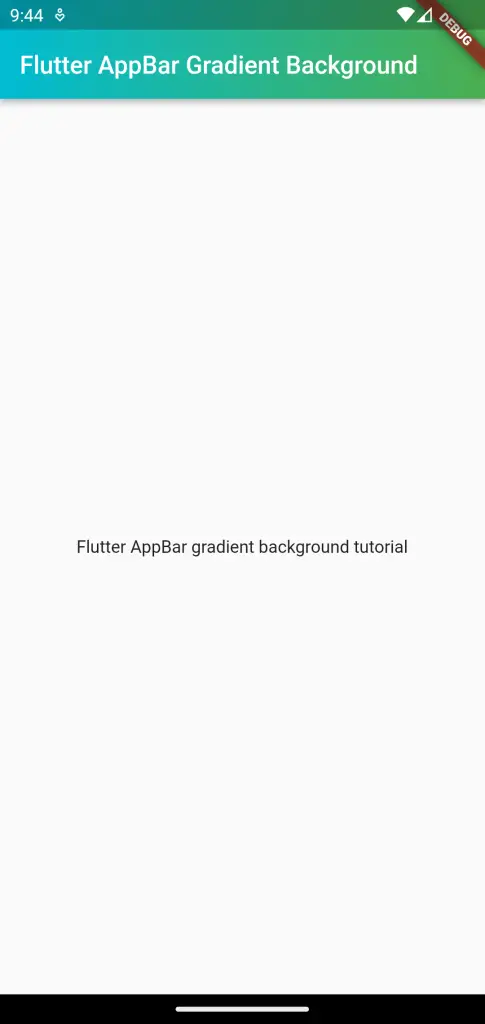
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
'Flutter AppBar Gradient Background',
),
flexibleSpace: Container(
decoration: const BoxDecoration(
gradient: LinearGradient(
begin: Alignment.centerLeft,
end: Alignment.centerRight,
colors: <Color>[Colors.cyan, Colors.green])),
)),
body: const Center(
child: Text('Flutter AppBar gradient background tutorial')));
}
}
I hope this Flutter AppBar gradient background example is helpful for you.