How to add Icon to Right of TextField in Flutter
The TextField widget in Flutter comes with various customizations. In this blog post, let’s learn how to add an icon to the right position of TextField in Flutter.
The TextInputDecoration class allows us to add icons inside the TextField. You can add an icon to the end of TextField using the suffixIcon property.
See the code snippet given below.
TextField(
decoration: const InputDecoration(suffixIcon: Icon(Icons.info)),
controller: _controller,
onSubmitted: (String value) {
debugPrint(value);
},
)
Following is the output.
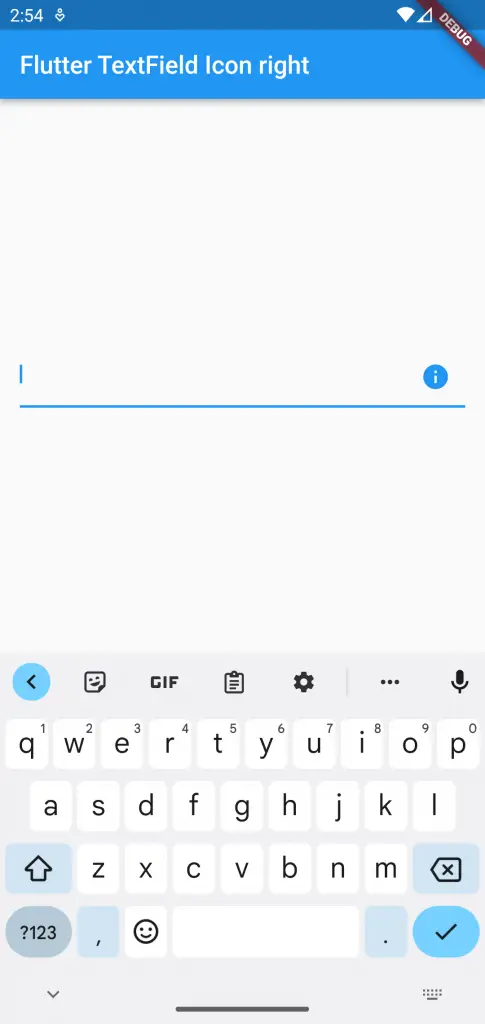
If you want to add an icon to the beginning then you should use the prefixIcon property.
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter TextField Icon right'),
),
body: const MyStatefulWidget());
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
late TextEditingController _controller;
@override
void initState() {
super.initState();
_controller = TextEditingController();
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Center(
child: Padding(
padding: const EdgeInsets.all(16.0),
child: TextField(
decoration: const InputDecoration(suffixIcon: Icon(Icons.info)),
controller: _controller,
onSubmitted: (String value) {
debugPrint(value);
},
),
));
}
}
That’s how you add the TextField icon to the right position in Flutter.