How to Find Difference Between Two Dates in Flutter
In this blog post, I am showing how to find the difference between two dates in Flutter. We have to make use of the DateTime class and its difference method for this purpose.
We can define two dates using the DateTime class as given below.
var start = DateTime(2022, DateTime.june, 6);
var end = DateTime(2022, DateTime.december, 10);
Now, you can get the difference using the difference method as well as the Duration class.
Duration difference = end.difference(start);
Following is the complete example where I show the output of the difference between two dates.
import 'package:flutter/material.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
useMaterial3: true,
),
home: const MyHomePage());
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
var start = DateTime(2023, DateTime.january, 6);
var end = DateTime(2023, DateTime.february, 10);
Duration difference = end.difference(start);
return Scaffold(
appBar: AppBar(
title: const Text(
'Flutter Days between two dates',
),
),
body: Center(child: Text('${difference.inDays.toString()} days')));
}
}
Following is the output.
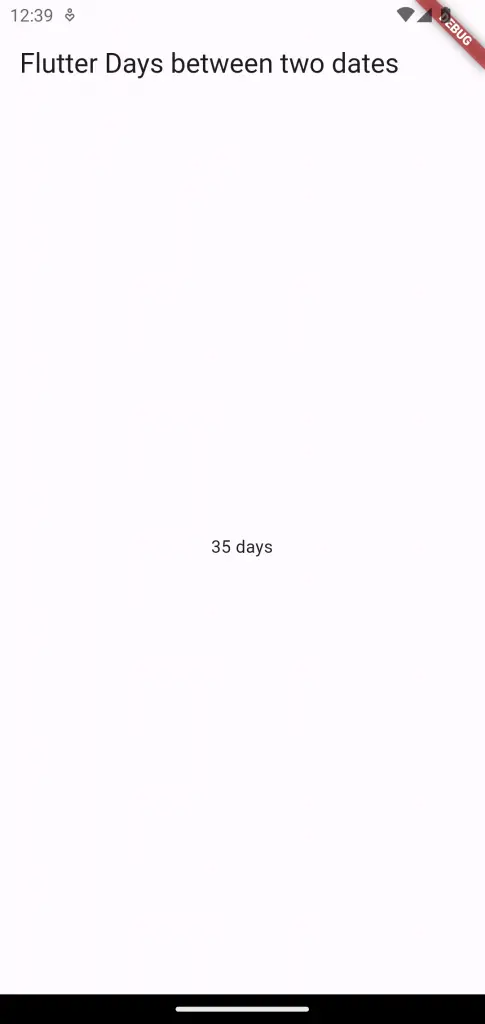
Sometimes, the date duration calculations can get messy. It’s always better to do some research before implementing it.