How to Validate TextField in Flutter
The TextField widget is the text input UI component in Flutter. But what if you want to show multiple text inputs in your app? You can use the Form widget for that.
The Form widget groups multiple form widgets and acts as a container. It makes validation of each form field widget easy. Using it with the TextFormField class will give you the desired output.
In this Flutter example, let’s see how to validate TextField using forms.
Following is the complete code to validate forms.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter TextField Validation'),
),
body: const MyStatefulWidget());
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
final _nameController = TextEditingController();
final _emailController = TextEditingController();
final _formKey = GlobalKey<FormState>();
@override
Widget build(BuildContext context) {
return Form(
key: _formKey,
child: Padding(
padding: const EdgeInsets.all(16.0),
child: Column(
crossAxisAlignment: CrossAxisAlignment.center,
children: <Widget>[
TextFormField(
decoration: const InputDecoration(
hintText: 'Enter your name',
),
controller: _nameController,
validator: (value) {
if (value!.isEmpty) {
return 'Please enter valid name';
}
return null;
},
),
TextFormField(
decoration: const InputDecoration(
hintText: 'Enter your email',
),
controller: _emailController,
validator: (value) {
if (!value!.contains('@')) {
return 'Please enter valid email';
}
return null;
},
),
Padding(
padding: const EdgeInsets.symmetric(vertical: 16.0),
child: ElevatedButton(
onPressed: () {
if (_formKey.currentState!.validate()) {
debugPrint('Validated!');
}
},
child: const Text('Submit'),
),
),
],
),
),
);
}
}
Every form must need a form key to validate its children. The key can be generated using GlobalKey class. Inside the Form widget, we use the TextFormField widget for each text input.
Each TextFormField widget has a validator property which is used for validation purposes. In the above example, we have two inputs and two validations. One field is validated for empty whereas the other for email. We validate the fields only when the submit button is pressed.
Following is the output of the Flutter form validation example.
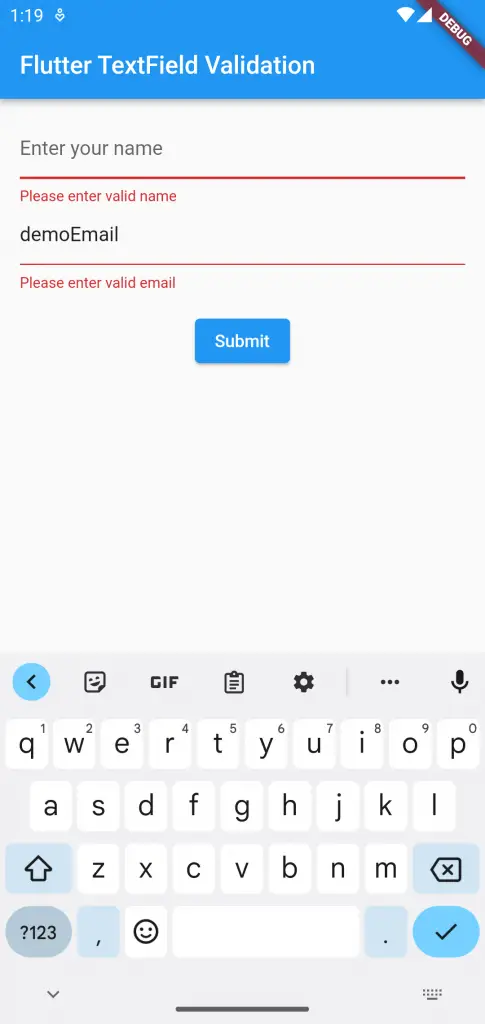
I hope you liked this Flutter tutorial. Thank you for reading.