How to Align Widgets at the Bottom of Column in Flutter
Layouts can be tricky, especially when you’re trying to align widgets in a specific way within a column. One such challenge is aligning widgets at the bottom of a column. In this blog post, let’s explore various ways to accomplish this task.
Use MainAxisAlignment
MainAxisAlignment in Flutter describes how child widgets should be laid out along the main axis within a parent Column or Row widget. It offers values like start, end, center, and spaceBetween, among others, to define the positioning and spacing of widgets.
The Code
Here’s a simple code snippet that demonstrates how to align widgets at the bottom of a column using mainAxisAlignment
:
Column(
mainAxisAlignment: MainAxisAlignment.end,
children: [
Text('First Child'),
Text('Second Child'),
],
)
Code Explanation
- Column Widget: The
Column
serves as the parent container for our widgets. - MainAxisAlignment.end: By setting the
mainAxisAlignment
property toMainAxisAlignment.end
, we align the children at the bottom of the column.
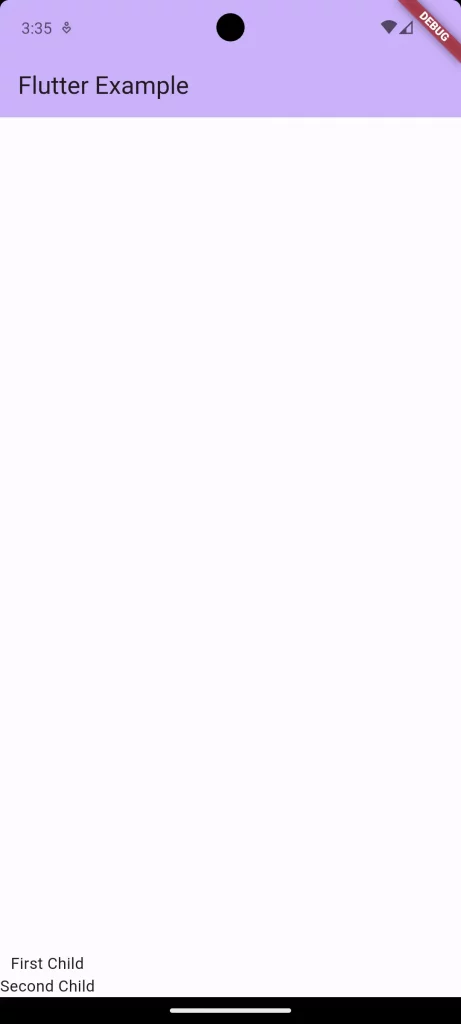
Use Spacer
Spacer
is a simple widget that takes up empty space in the layout, thus pushing other widgets away from itself. It’s particularly useful for creating gaps between widgets or for pushing widgets to the corners of the layout.
The Code
You can also use a Spacer
to push your widgets to the bottom:
Column(
children: [
Spacer(),
Text('First Child'),
Text('Second Child'),
],
)
Code Explanation
- Spacer: This widget takes up all available space, pushing the widgets that come after it to the bottom.
- Column: It serves as the parent container for our widgets, including the
Spacer
.
Aligning widgets at the bottom of a column in Flutter can be done in various ways. Each has its pros and cons. MainAxisAlignment
is simple, Spacer
is dynamic, and custom Padding
gives you the most control. Depending on your project’s needs, you can choose the best method for you.